1. 介绍
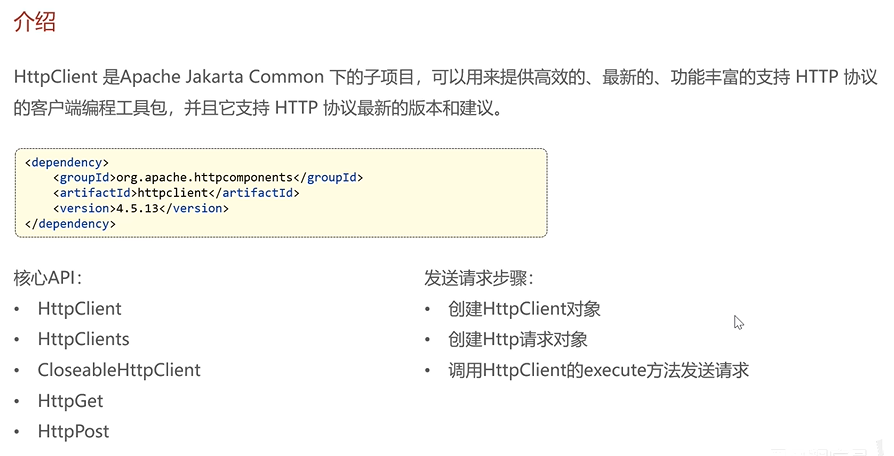
2. 发送Get方式请求
public void httpGetTest() throws Exception{
// 创建HttpClient对象
CloseableHttpClient httpClient = HttpClients.createDefault();
// 创建请求方式对象
HttpGet httpGet = new HttpGet("http://localhost/user/shop/status");
// 发送请求
CloseableHttpResponse response = httpClient.execute(httpGet);
// 解析响应结果
// 获取响应状态码
int statusCode = response.getStatusLine().getStatusCode();
System.out.println("响应状态码为, " + statusCode);
// 获取响应结果
HttpEntity entity = response.getEntity();
String body = EntityUtils.toString(entity);
System.out.println("响应结果为:" + body);
// 关闭资源
response.close();
httpClient.close();
}
3. 发送Post方式请求
public void httpPostTest() throws Exception {
// 创建HttpClient对象
CloseableHttpClient httpClient = HttpClients.createDefault();
// 创建请求方式对象
HttpPost httpPost = new HttpPost("http://localhost:8080/admin/employee/login");
// 构建请求参数
JSONObject params = new JSONObject();
params.put("username", "admin");
params.put("password", "123456");
System.out.println(params.toString());
StringEntity entity = new StringEntity(params.toString());
// 设置请求参数类型和编码格式
entity.setContentEncoding("utf-8");
entity.setContentType("application/json");
httpPost.setEntity(entity);
// 发送请求
CloseableHttpResponse response = httpClient.execute(httpPost);
// 解析响应结果
// 获取响应状态码
int statusCode = response.getStatusLine().getStatusCode();
System.out.println(statusCode);
// 获取响应结果
HttpEntity responseEntity = response.getEntity();
String result = EntityUtils.toString(responseEntity);
System.out.println(result);
// 释放资源
response.close();
httpClient.close();
}