效果图:
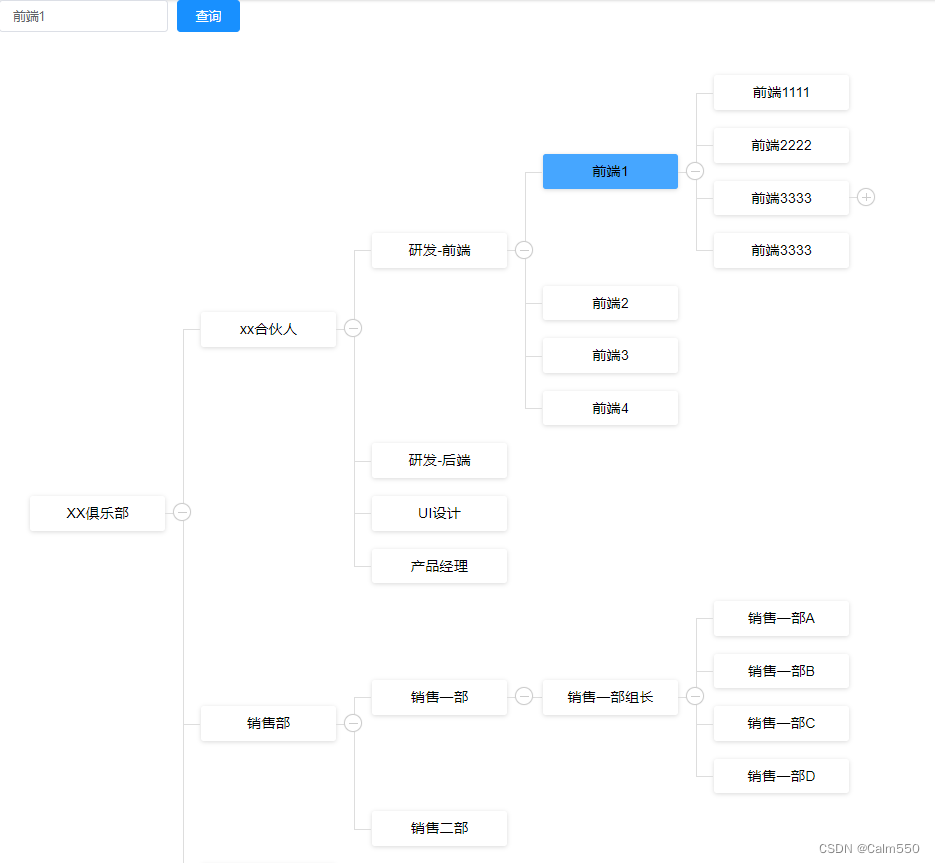
代码:
<template>
<div class="AllTree">
<el-form :inline="true" :model="formInline" class="demo-form-inline">
<el-form-item>
<el-input v-model="formInline.user" placeholder="请输入名称"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="handleSearch">查询</el-button>
</el-form-item>
</el-form>
<div ref="treeContainer" class="tree-container">
<org-tree :data="data" :horizontal="true" name="test" :label-class-name="labelClassName" collapsable
@on-expand="onExpand" @on-node-click="clickTree" ref="orgTree" :label-width="150"
:line-style="{ stroke: '#ccc', strokeWidth: 2 }">
</org-tree>
</div>
<!-- 俱乐部信息弹窗 -->
<el-drawer title="俱乐部信息" :visible.sync="drawer" :before-close="handleClose">
<span>我来啦!</span>
</el-drawer>
</div>
</template>
初始化定义数据:
import OrgTree from 'vue2-org-tree';
import 'vue2-org-tree/dist/style.css';
export default {
components: {
orgTree: OrgTree
},
data() {
return {
formInline: {
user: ''
},
id: null,
drawer: false,
data:{},
data1: {
id: 0,
label: "XX俱乐部",
children: [
{
id: 2,
label: "xx合伙人",
children: [
{
id: 5,
label: "研发-前端",
children: [
{
id: 55,
label: "前端1",
children: [
{
id: 555,
label: "前端1111",
},
{
id: 556,
label: "前端2222",
},
{
id: 557,
label: "前端3333",
children: [{
id: 5557,
label: "前端11111",
},
{
id: 5558,
label: "前端22222",
},
{
id: 5559,
label: "前端33333",
},
{
id: 5560,
label: "前端44444",
},
{
id: 5561,
label: "前端55555",
}]
},
{
id: 558,
label: "前端3333",
}
]
},
{
id: 56,
label: "前端2"
},
{
id: 57,
label: "前端3"
},
{
id: 58,
label: "前端4"
}
]
},
{
id: 6,
label: "研发-后端"
},
{
id: 9,
label: "UI设计"
},
{
id: 10,
label: "产品经理"
}
]
},
{
id: 3,
label: "销售部",
children: [
{
id: 7,
label: "销售一部",
children: [
{
id: 78,
label: "销售一部组长",
children: [{
id: 788,
label: "销售一部A",
},
{
id: 789,
label: "销售一部B",
},
{
id: 790,
label: "销售一部C",
},
{
id: 791,
label: "销售一部D",
}]
}
]
},
{
id: 8,
label: "销售二部"
}
]
},
{
id: 4,
label: "财务部"
},
{
id: 9,
label: "HR人事"
}
]
},
defaultProps: {
children: 'children',
label: 'label'
},
zoom: 1,
BasicSwich: false,
collapsable: true,
labelClassName: "org-bg-white",
clickTimeout: null
};
},
搜索事件定义方法:
created() {
this.getTreeData();
},
methods: {
//获取节点数据
getTreeData(){
this.data=this.data1
this.expandChange();
},
renderContent(h, data) {
return data.label;
},
// 树状结构折叠打开
onExpand(e, data) {
if ("expand" in data) {
data.expand = !data.expand;
if (!data.expand && data.children) {
this.collapse(data.children);
}
} else {
this.$set(data, "expand", true);
}
},
collapse(list) {
var _this = this;
list.forEach(function (child) {
if (child.expand) {
child.expand = false;
}
child.children && _this.collapse(child.children);
});
},
expandChange() {
this.toggleExpand(this.data, true);
},
toggleExpand(data, val) {
var _this = this;
if (Array.isArray(data)) {
data.forEach(function (item) {
_this.$set(item, "expand", val);
if (item.children) {
_this.toggleExpand(item.children, val);
}
});
} else {
this.$set(data, "expand", val);
if (data.children) {
_this.toggleExpand(data.children, val);
}
}
},
// 搜索事件
handleSearch() {
const isLabelFound = this.findLabel(this.data, this.formInline.user.trim());
console.log('isLabelFound',isLabelFound)
},
findLabel(node, targetLabel) {
// 清除先前设置的背景色
this.clearHighlight();
const searchInChildren = (node) => {
if (node.id == 0) {
node.expand = true
}
//检查当前节点
if (node.label === targetLabel) {
this.id = node.id;
node.expand = true;
this.highlightLabel(targetLabel, "#46a6ff"); // 设置背景色为 pink
return true;//找到目标节点
}
//递归搜索子节点
if (node.children) {
for (let child of node.children) {
if (searchInChildren(child)) {
child.expand = true
return true;//如果在子节点找到目标节点,直接返回true
}
}
}
return false;//当前节点及其子节点都未找到目标节点
}
const found = searchInChildren(node)
if (!found) {
this.$message.warning('未找到该项')
}
return found ? this.id : false
},
highlightLabel(targetLabel, color) {
// 异步更新,等待 DOM 渲染完成后再操作
setTimeout(() => {
let orgTreeList = document.getElementsByClassName('org-tree-node-label-inner');
for (let i = 0; i < orgTreeList.length; i++) {
if (orgTreeList[i].innerText === targetLabel) {
orgTreeList[i].style.backgroundColor = color;
break; // 找到第一个匹配的节点后即可退出循环
}
}
}, 0);
},
clearHighlight() {
let orgTreeList = document.getElementsByClassName('org-tree-node-label-inner');
for (let i = 0; i < orgTreeList.length; i++) {
orgTreeList[i].style.backgroundColor = ""; // 清除背景色
}},
//点击事件
clickTree(e, data) {
if (this.clickTimeout) {
// 如果存在单击事件的计时器,则视为双击事件
clearTimeout(this.clickTimeout);
this.$router.push({
path: "/dataVisualization/recommendedLinksDetail",
query: {
id: data.id,
},
});
} else {
// 否则,启动单击事件计时器
this.clickTimeout = setTimeout(() => {
this.drawer = true
this.clickTimeout = null; // 清除计时器
}, 500); // 200毫秒内判断是否双击
}
// const depth = this.getNodeDepth(data);
// if (depth === 1) {
// // 第一层节点,显示弹窗或其他操作
// this.drawer = true
// } else {
// // this.$router.push({
// // path: "/dataVisualization/recommendedLinksDetail",
// // query: {
// // id: data.id,
// // },
// // });
// console.log(111)
// }
},
getNodeDepth(node, depth = 0) {
// 递归计算节点的深度(层级)
console.log(node)
console.log(node.parent)
if (node.parent) {
return this.getNodeDepth(node.parent, depth + 1);
}
return depth;
},
handleClose() {
this.drawer = false
}
}
}