1. list 容器
1.1 初始化,获取读取
#include <iostream>
#include<list>
using namespace std;
void printList(const list<int>&L){
for(list<int>::const_iterator it = L.begin(); it != L.end(); it++){
cout << *it <<" ";
}
cout << endl;
}
int main()
{
list<int>L1;
list<int>L2 = {0, 1,2,3};
L1.push_back(10);
L1.push_back(20);
L1.push_back(30);
// cout << *( next(L1.begin(), 1) ) << endl; //迭代器后移
printList(L2);
return 0;
}
1.2 插入删除
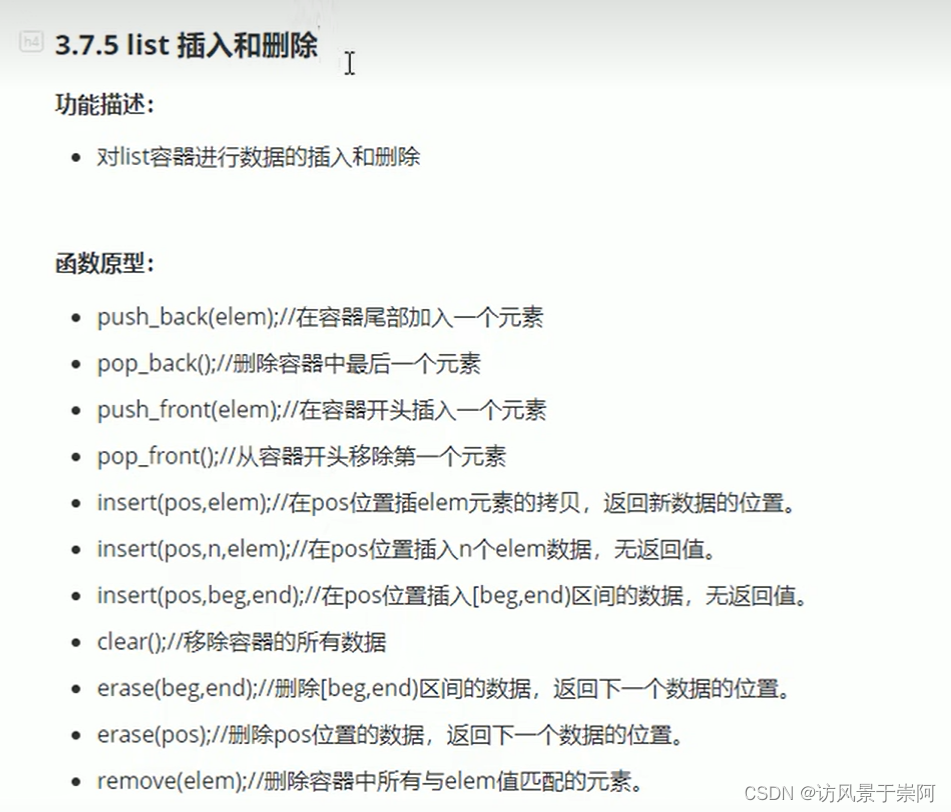
#include <iostream>
#include<list>
using namespace std;
void printList(const list<int>&L){
for(list<int>::const_iterator it = L.begin(); it != L.end(); it++){
cout << *it <<" ";
}
cout << endl;
}
int main()
{
list<int>L1;
list<int>L2 = {0, 1,2,3};
L1.push_back(10);
L1.push_back(20);
L1.push_back(30);
L1.insert(++L1.begin(), 100);
list<int>::iterator it= L1.begin();
int n=3;
while(n--){
it++;
}
L1.insert(it, 999); //迭代器指向了最后一个位置,在其前面插入
printList(L1);
//删除
L1.erase(it);
printList(L1);
L1.remove(100); //直接删除
// cout << *( next(L1.begin(), 1) ) << endl; //迭代器后移
printList(L1);
return 0;
}