参考资料:
- 《C++ Primer》第5版
- 《C++ Primer 习题集》第5版
9.5 额外的string
操作(P320)
9.5.1 构造string
的其他方法
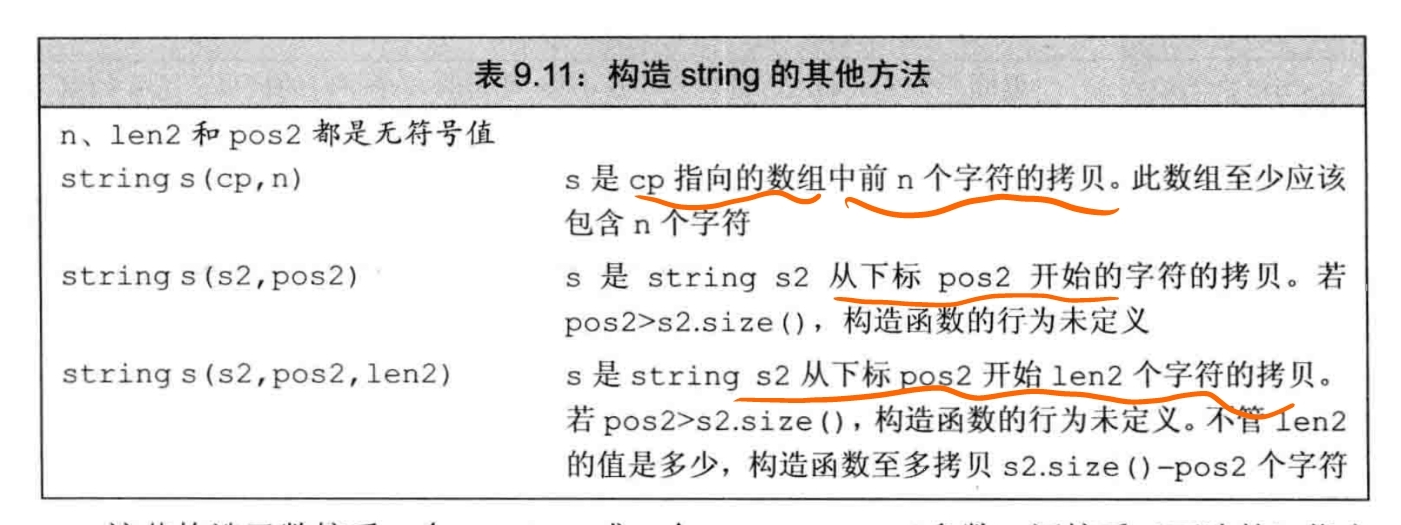
const char *cp = "hello, world!";
char arr[] = { 'h','\0','i','\0' };
string s1(cp); // s1 == "hello, world!"
string s2(arr, 2); // s2 == "h", size为2
string s3(arr); // s3 == "h", size为1
string s4(cp + 7); // s4 == "world!"
string s5(s1, 7); // s5 == "world!"
string s6(s1, 7, 3); // s6 == "wor"
string s7(s1, 16); // 抛出out_of_range异常
string s8(arr, 10); // s8 == "hi烫烫烫"
用 const char*
创建 string
时,指向的数组必须以空字符结尾,拷贝操作遇到空字符停止。如果我们还提供了一个计数值,数组就不必以空字符结尾。如果我们未传递计数值且数组不以空字符结尾,或计数值大于数组大小,则构造函数的行为是未定义的。
用 string
创建 string
时,我们可以提供一个可选的开始位置和一个计数值,开始位置必须小于等于给定 string
的大小。不管我们要求拷贝多少个字符,标准库最多拷贝到 string
结尾。
substr
操作

如果开始位置超过 string
的大小,函数会抛出 out_of_range
异常。substr
最多拷贝到字符串末尾。
9.5.2 改变string
的其他方法
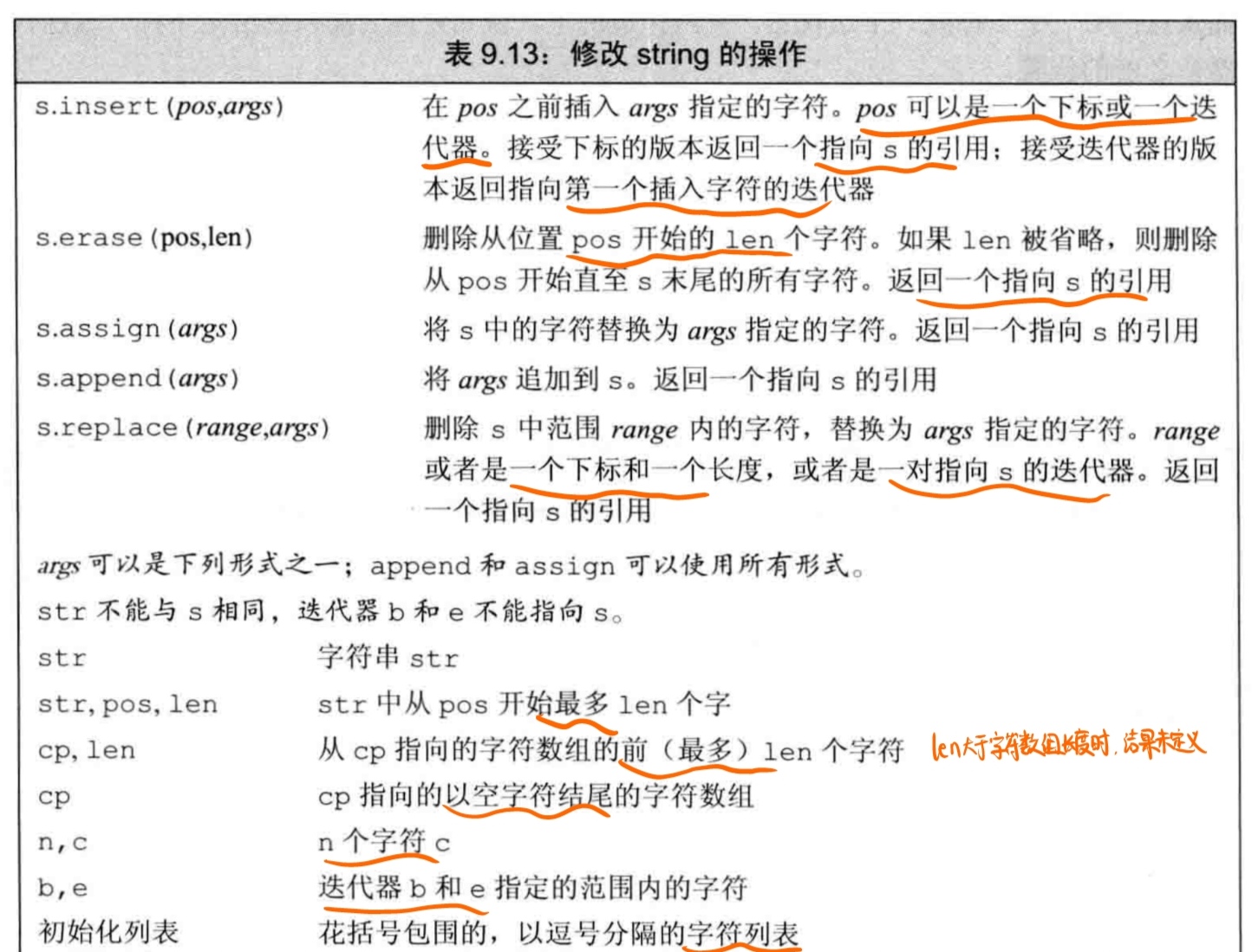
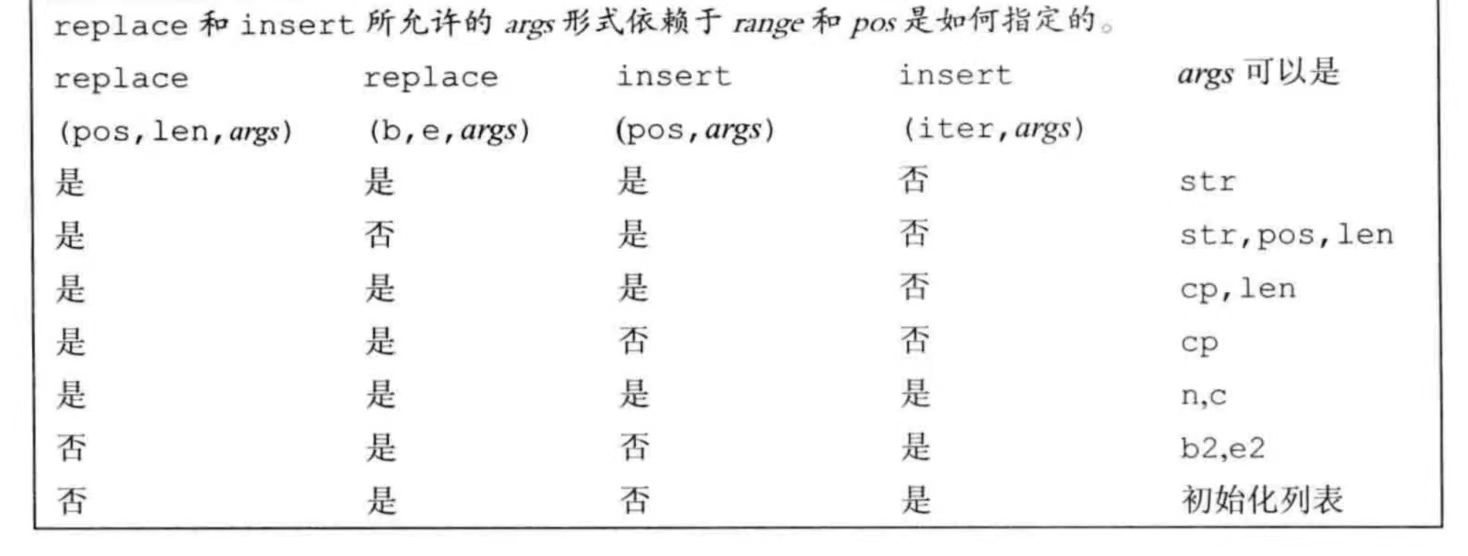
9.5.3 string
搜索操作(P325)
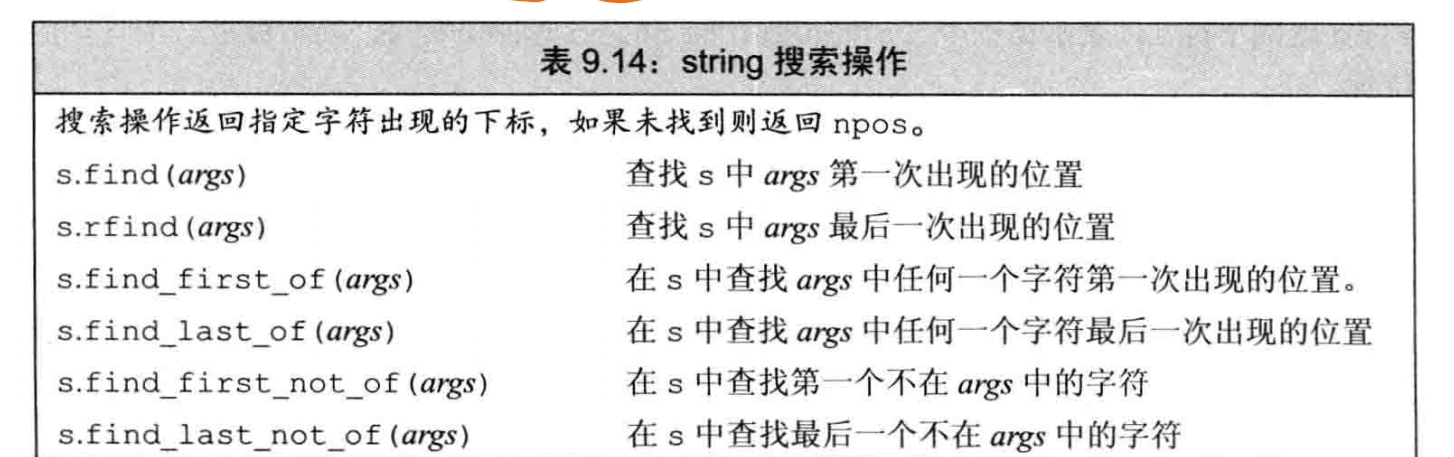
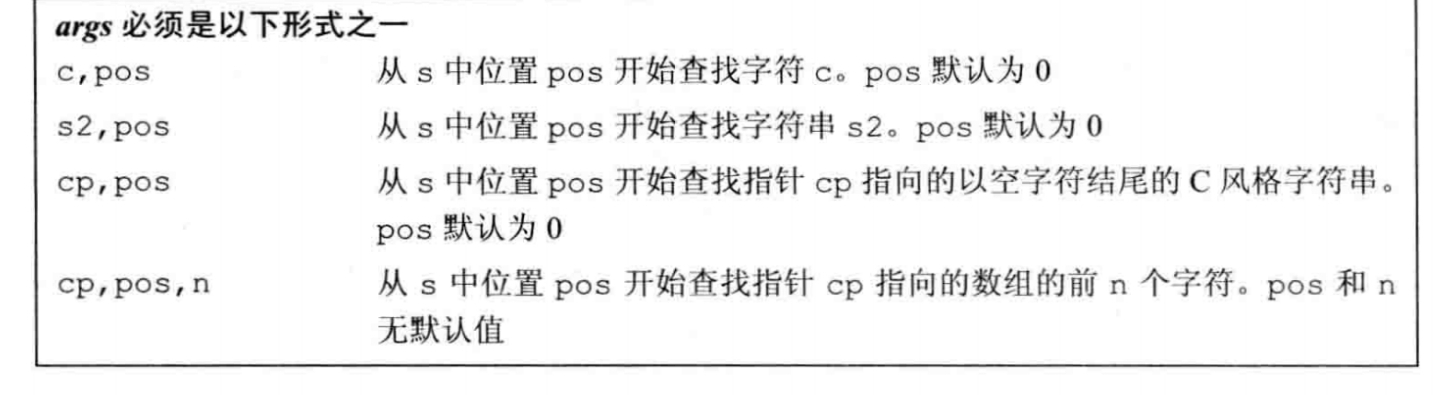
每个搜索操作都有 4 个重载版本,都返回一个 string::size_type
值,表示匹配发生位置的下标;如果搜索失败,则返回一个名为 string::npos
的 static
成员,其类型为 const string::size_type
,初始化为值 -1
,由于 npos
是无符号类型,所以 -1
意味着 string
最大的可能大小。
string
搜索函数返回的是无符号类型,所以最好不要有int
等类型保存
string s("hello, world!1234");
auto pos1 = s.find("wor"); // pos1 == 7
string number("0123456789");
auto pos2 = s.find_first_of(number); // pos2 == 13
auto pos3 = s.find_first_not_of(number); // pos3 == 0
auto pos4 = s.find_last_not_of(number); // pos4 == 12
指定从哪里开始搜索
string number("0123456789");
string s("0t1w15h218d");
string::size_type pos = 0;
// 输出所有数字的下标
while ((pos = s.find_first_of(number, pos)) != string::npos) {
cout << pos << ' ';
++pos; // 移动到下一个字符
}
逆向搜索
9.5.4 compare
函数(P327)
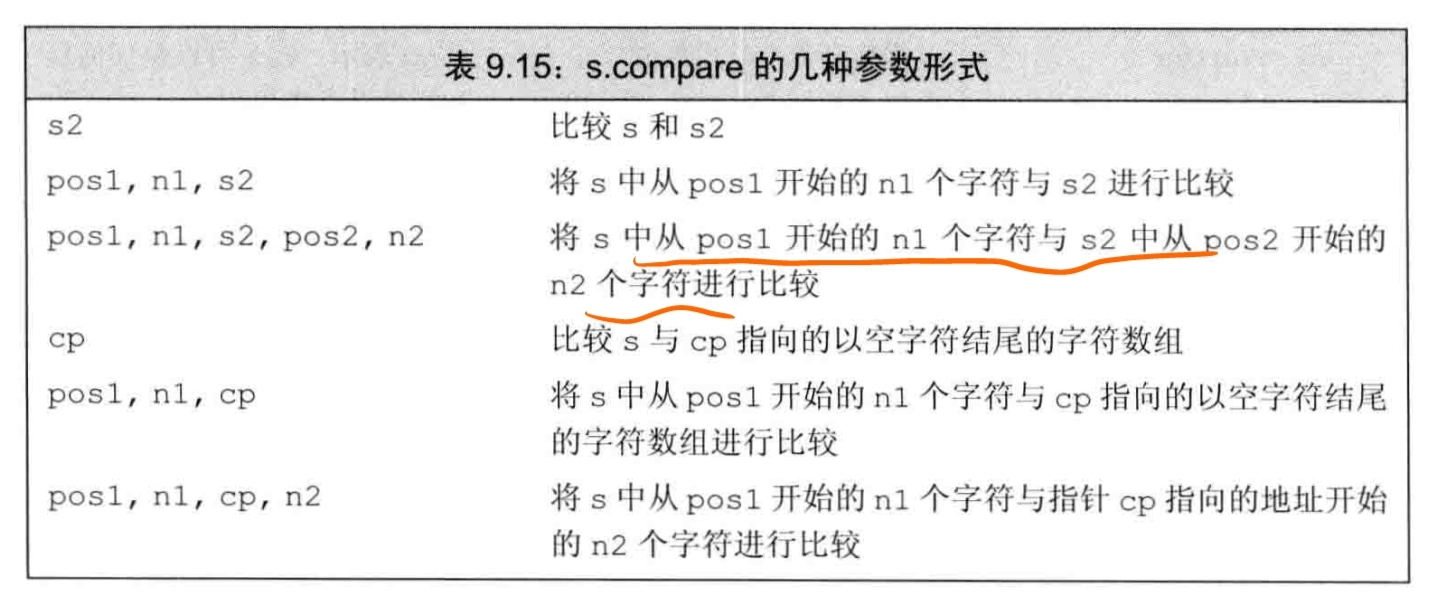
9.5.5 数值转换(P327)
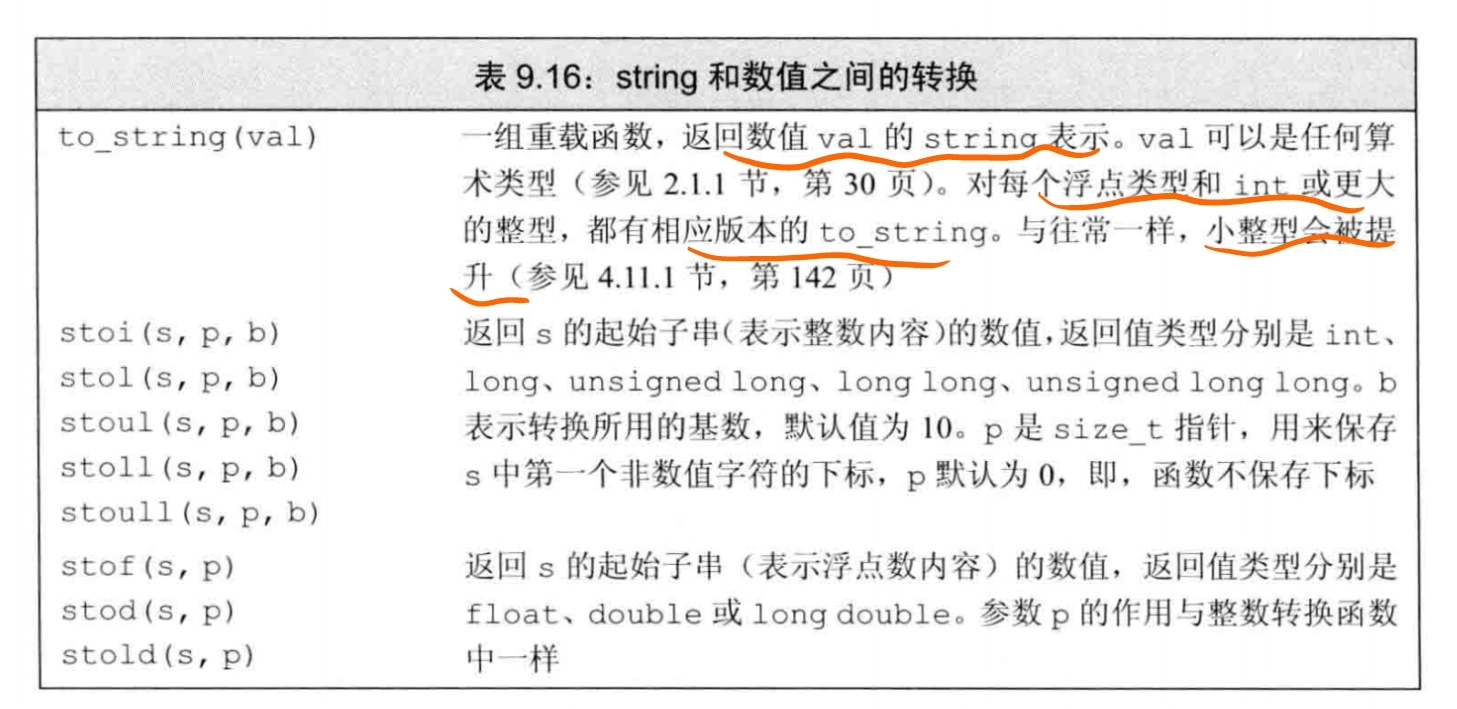
如果 string
不能转换成一个数值,则函数抛出 invalid_argument
异常;如果得到的数值无法用任何类型表示,则抛出 out_of_range
异常。
string s("pi = 3.14, hello");
string number("0123456789");
size_t pos = 0;
auto d = stod(s.substr(s.find_first_of(number)), &pos);
9.6 容器适配器(P329)
标准库定义了三个顺序容器适配器(adaptor):stack
、queue
、priority_queue
。