文章目录
- 1、ProductController
- 2、AdminCommonService
- 3、ProductApiService
- 4、ProductCommonService
- 5、ProductSqlService
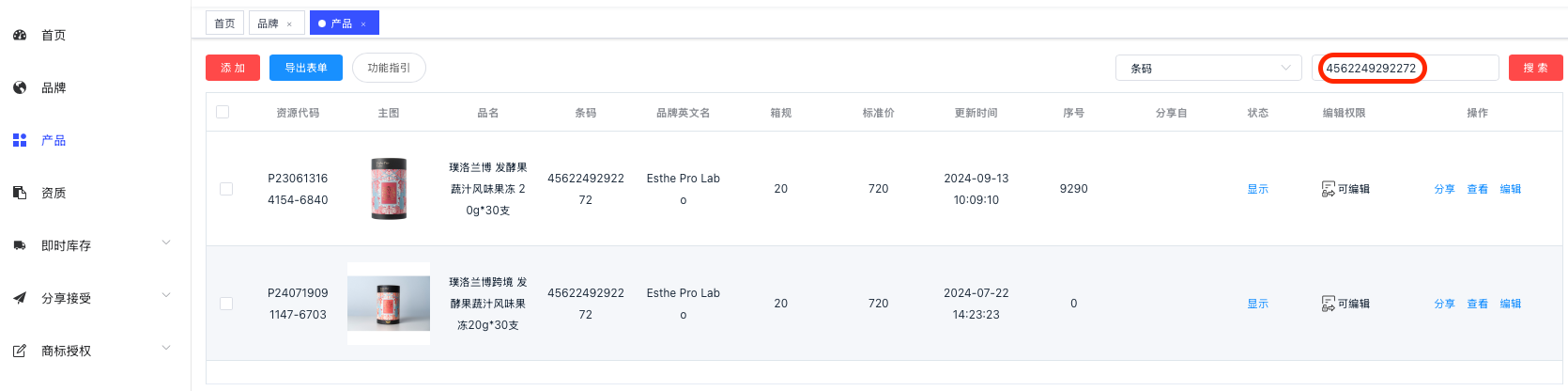
https://api.crossbiog.com/product/admin/list?page=0&size=10&field=jancode&value=4562249292272
1、ProductController
@GetMapping("admin/list")
@ApiOperation("分页列表")
public BaseResult list(PageWithSearch basePage, @ApiIgnore @SessionAttribute(Constants.ADMIN_ID) Integer adminId) {
checkParam(basePage.getField(), basePage.getValue());
adminId = adminCommonService.getVipIdByProduct(adminId);
return BaseResult.success(productApiService.findPage(adminId, basePage));
}
2、AdminCommonService
/**
* 获取商品管理人的上级vip id
* 当操作者为商品管理人时获取上级的vip id
* 否则返回自身
*/
public Integer getVipIdByProduct(Integer nowId) {
return hasRole(nowId, Admin.ROLE_PRODUCT) || hasRole(nowId, Admin.ROLE_QUALIFICATION) || hasRole(nowId, Admin.ROLE_STORE) ? findCompanySuperId(nowId) : nowId;
}
/**
* 查询公司超管id
*/
public Integer findCompanySuperId(Integer adminId) {
return adminService.findCompanySuperId(adminId);
}
3、ProductApiService
/**
* 分页列表
*/
public Page<ProductListDto> findPage(Integer nowId, PageWithSearch page) {
Page<ProductWithShareDto> productPage = productCommonService.findPage(nowId, page);
return new PageImpl<>(convertToListDto(productPage.getContent(), nowId), page.toPageable(), productPage.getTotalElements());
}
4、ProductCommonService
/**
* 产品管理-产品分页列表
*/
public Page<ProductWithShareDto> findPage(Integer nowId, PageWithSearch basePage) {
return productSqlService.findPage(nowId, basePage);
}
5、ProductSqlService
/**
* 产品管理-分页列表
*/
public Page<ProductWithShareDto> findPage(Integer nowId, PageWithSearch basePage) {
StringBuilder sql = new StringBuilder();
Map<String, Object> paramMap = new HashMap<>(4);
sql.append("SELECT DISTINCT ").append(SqlUtil.sqlGenerate("p", Product.class)).append(",a.send_id, a.edit_auth FROM product p ");
if (!StringUtils.isEmpty(basePage.getField()) && !StringUtils.isEmpty(basePage.getValue()) && brandParamStr.contains(basePage.getField())) {
sql.append("INNER JOIN brand b ON p.brand_id = b.id AND b.").append(SqlUtil.camelToUnderline(basePage.getField().replaceAll("brand", ""))).append(" LIKE :").append(basePage.getField()).append(" ");
paramMap.put(basePage.getField(), "%" + basePage.getValue() + "%");
}
//sql.append("LEFT JOIN product_admin_mapping a ON p.id = a.product_id ");
//sql.append("AND a.admin_id=").append(nowId).append(" AND a.read_auth =").append(CommonStatusEnum.NORMAL.getValue()).append(" ");
//sql.append("WHERE (p.creator_id =").append(nowId).append(" OR ").append("a.id IS NOT NULL) ");
sql.append("INNER JOIN product_admin_mapping a ON p.id = a.product_id AND a.admin_id=").append(nowId).append(" ");
//有编辑权限 || (有查看权限 && 产品状态为显示)
sql.append("WHERE (a.edit_auth =").append(CommonStatusEnum.NORMAL.getValue()).append(" OR (a.read_auth =").append(CommonStatusEnum.NORMAL.getValue()).append(" AND p.status =").append(CommonStatusEnum.NORMAL.getValue()).append(")) ");
paramHandle(sql, paramMap, basePage.getField(), basePage.getValue());
sql.append("ORDER BY p.ranks DESC,p.created_date DESC ");
List result = executeSql(sql, paramMap, basePage.getPage(), basePage.getSize());
if (result.isEmpty()) {
return new PageImpl<>(Collections.emptyList(), basePage.toPageable(), 0);
}
return new PageImpl<>(parseToProductWithShare(result), basePage.toPageable(), countPage(nowId, basePage.getField(), basePage.getValue()));
}
需求:微信小程序使用二维码扫描条形码查询商品,接口使用上述pc端的。
// 扫描二维码
scanQrcode: function() {
wx.scanCode({
onlyFromCamera: false, // 允许从相机和相册中选择图片
success: (res) => {
const jancode = res.result;
console.log("扫描结果:", jancode);
this.getProductByJancode(jancode);
},
fail: (err) => {
wx.showToast({
title: '扫描失败,请重试',
icon: 'none'
});
}
});
},
// 获取 token
getToken: function() {
return new Promise((resolve,reject)=>{
const token = wx.getStorageSync('token')
console.log('Token:', token);
resolve(token)
});
},
// 根据条码查询产品信息
getProductByJancode: function(jancode) {
this.getToken().then((token) => {
if (!token) {
wx.showToast({
title: '获取 token 失败,请重试',
icon: 'none'
});
return;
}
// 构建带有查询参数的URL
const url = `https://api.crossbiog.com/product/admin/list?page=0&size=10&field=jancode&value=${encodeURIComponent(jancode)}`;
wx.request({
url: url, // 使用新的URL
//url: `https://api.crossbiog.com/product/admin/detailByJancode`, // 使用配置文件中的URL
//url: `http://localhost:8087/product/admin/detailByJancode`,
method: 'GET',
data: {
//jancode: jancode
},
header: {
'token': `${token}`
},
success: (res) => {
console.log("res=" + res);
console.log("后端返回的数据:", res.data); // 添加日志输出
// if (res.statusCode === 200 && res.data && res.data.data) {
// const product = res.data.data;
// if (product) {
// // 显示产品信息
// this.setData({
// products: [product],
// showNoResultsImage: false // 如果有结果,隐藏无结果图片
// });
// } else {
// // 没有找到产品
// wx.showToast({
// title: '未找到该条码对应的产品',
// icon: 'none'
// });
// this.setData({
// showNoResultsImage: true // 如果没有结果,显示无结果图片
// });
// }
// } else {
// wx.showToast({
// title: '数据加载失败',
// icon: 'none'
// });
// }
if (res.statusCode === 200 && res.data) {
const products = res.data.data.content || []; // 获取产品列表,如果没有则为空数组
const formattedProducts = products.map(product => ({
...product,
image: `https://www.crossbiog.com/${product.image}`
}));
if (products.length > 0) {
// 显示产品信息
this.setData({
products: [...formattedProducts],
//products: products, // 直接赋值整个产品列表
showNoResultsImage: false // 如果有结果,隐藏无结果图片
});
} else {
// 没有找到产品
wx.showToast({
title: '未找到该条码对应的产品',
icon: 'none'
});
this.setData({
showNoResultsImage: true // 如果没有结果,显示无结果图片
});
}
} else {
wx.showToast({
title: '数据加载失败',
icon: 'none'
});
}
},
fail: (err) => {
wx.showToast({
title: '请求失败',
icon: 'none'
});
}
});
}).catch((err) => {
wx.showToast({
title: err.message,
icon: 'none'
});
});
},