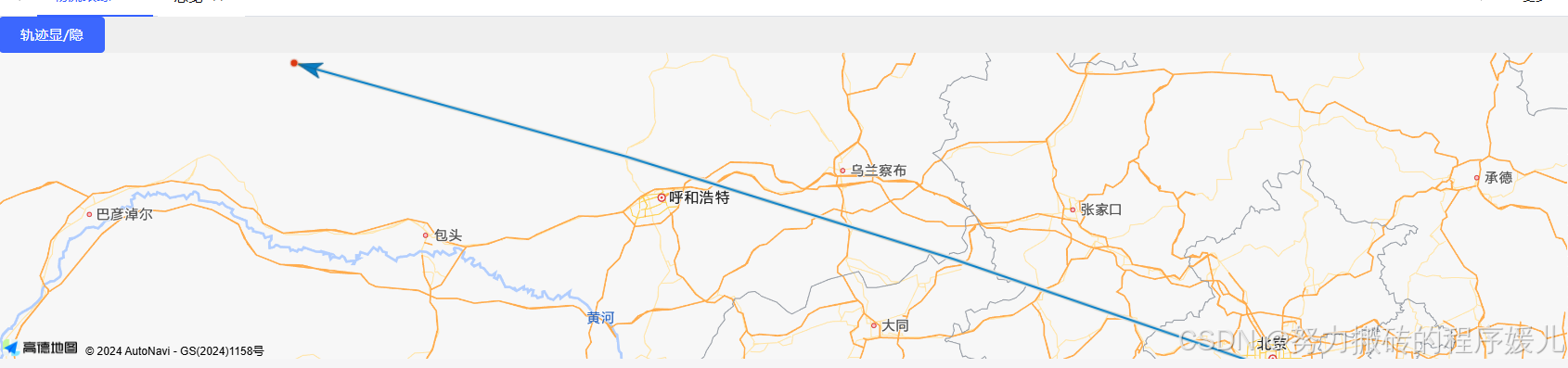
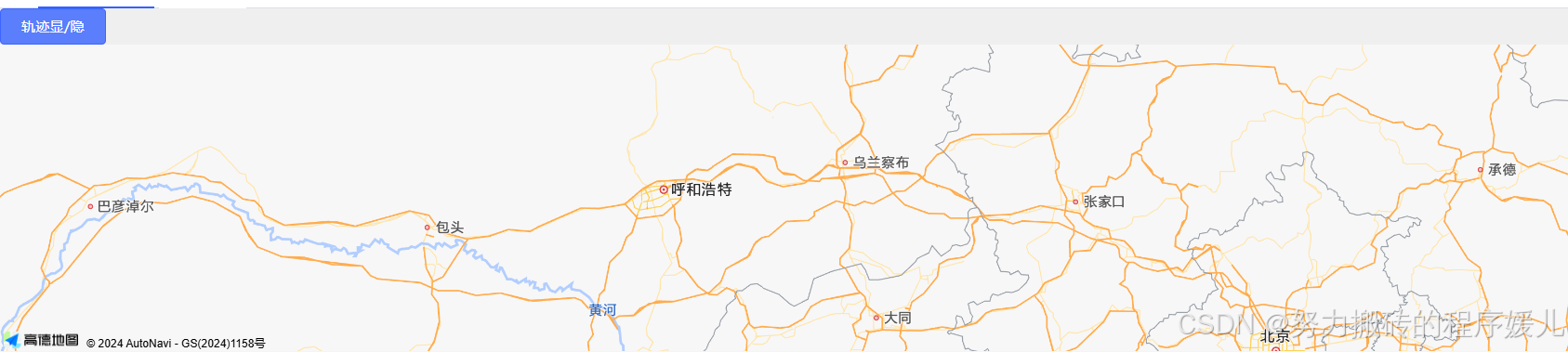
<template>
<div>
<el-button type="primary" @click="pathShowOrHide">
轨迹显/隐
</el-button>
<div id="container" />
</div>
</template>
<script>
import AMapLoader from '@amap/amap-jsapi-loader'
export default {
name: 'MapView',
data() {
return {
map: null,
PathSimplifier: null,
$: null,
pathState: true
}
},
mounted() {
this.initAMap()
},
unmounted() {
this.map?.destroy()
},
methods: {
initPage(PathSimplifier, $) {
const pathSimplifierIns = new PathSimplifier({
zIndex: 100,
// autoSetFitView:false,
map: this.map, // 所属的地图实例
getPath: function(pathData, pathIndex) {
return pathData.path
},
getHoverTitle: function(pathData, pathIndex, pointIndex) {
return null
}
})
window.pathSimplifierIns = pathSimplifierIns
pathSimplifierIns.setData([{
name: '测试',
path: [
[116.405289, 39.904987],
[113.964458, 40.54664],
[111.47836, 41.135964],
[108.949297, 41.670904]
]
}])
const pathNavigatorStyles = [{
width: 16,
height: 24,
content: 'defaultPathNavigator'
}
]
function extend(dst) {
if (!dst) {
dst = {}
}
const slist = Array.prototype.slice.call(arguments, 1)
for (let i = 0, len = slist.length; i < len; i++) {
const source = slist[i]
if (!source) {
continue
}
for (const prop in source) {
if (source.hasOwnProperty(prop)) {
dst[prop] = source[prop]
}
}
}
return dst
}
let idx = 0
const navg1 = pathSimplifierIns.createPathNavigator(0, {
loop: true,
speed: 1000000,
pathNavigatorStyle: extend({}, pathNavigatorStyles[0])
})
navg1.start()
function changeNavgContent() {
// 获取到pathNavigatorStyle的引用
const pathNavigatorStyle = navg1.getStyleOptions()
// 覆盖修改
extend(pathNavigatorStyle, pathNavigatorStyles[(++idx) % pathNavigatorStyles.length])
// 重新绘制
pathSimplifierIns.renderLater()
}
setInterval(changeNavgContent, 500)
},
initAMap() {
const that = this
AMapLoader.load({
key: 'ed030cd90d1a6014ea01f26d51250f40', // 申请好的Web端开发者Key,首次调用 load 时必填
version: '2.0', // 指定要加载的 JSAPI 的版本,缺省时默认为 1.4.15
plugins: ['AMap.Scale'], // 需要使用的的插件列表,如比例尺'AMap.Scale',支持添加多个如:['...','...']
AMapUI: {
version: '1.1',
plugins: ['overlay/SimpleMarker']
}// 需要使用的的插件列表,如比例尺'AMap.Scale'等
})
.then((AMap) => {
this.map = new AMap.Map('container', {
// 设置地图容器id
viewMode: '3D', // 是否为3D地图模式
zoom: 4, // 初始化地图级别
center: [116.397428, 39.90923] // 初始化地图中心点位置
})
AMapUI.load(['ui/misc/PathSimplifier', 'lib/$'], (PathSimplifier, $) => {
if (!PathSimplifier.supportCanvas) {
alert('当前环境不支持 Canvas!')
return
}
this.PathSimplifier = PathSimplifier
this.$ = $
this.initPage(PathSimplifier, $)
})
})
.catch((e) => {
console.log(e)
})
},
pathShowOrHide() {
if (this.pathState) {
window.pathSimplifierIns.setData([])
this.pathState = false
} else {
this.initPage(this.PathSimplifier, this.$)
this.pathState = true
}
}
}
}
</script>
<style scoped>
#container {
width: 100%;
height: 300px;
}
</style>