C#学习
- 1.B站丑萌气质狗
- C#的循环-判断
- 泛型
- 错误处理
- 面向对象
- static的使用
- 定义showInfo类和Hero类
- 在这里插入图片描述 然后在该解决方案add新建一个类库,点击rebuild,会在bin文件夹下生成.dll文件 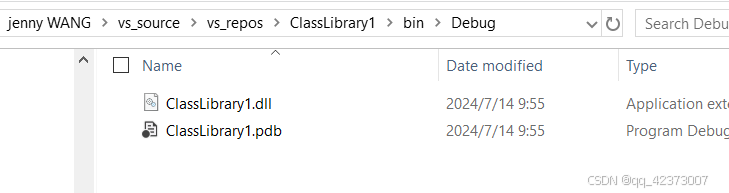 现在解决方案里有ConsoleAppFrameWork和ClassLibrary两个项目,需要把类库中的.dll文件引入进来,然后会在ConsoleAppFrameWork的References下面看到。 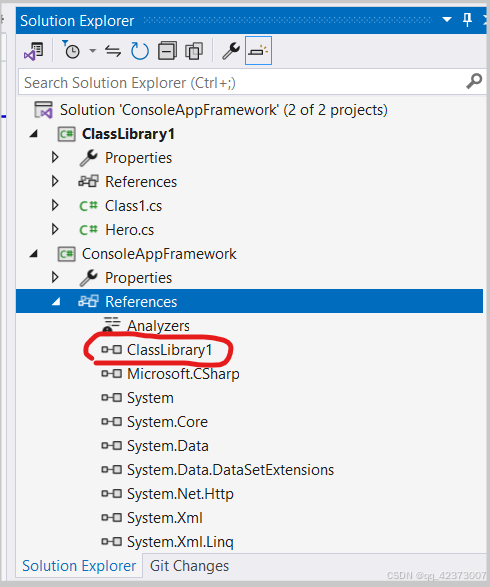 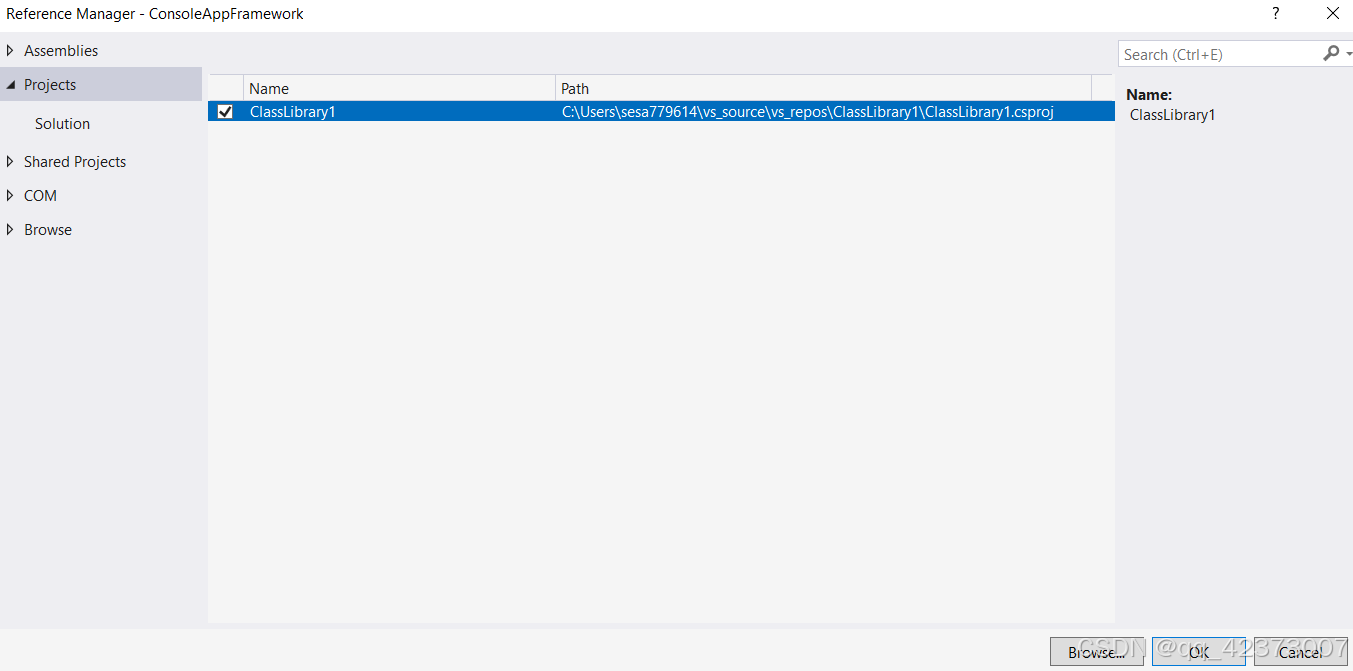
- 文件读写
1.B站丑萌气质狗
视频链接
C#的循环-判断
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleAppFramework
{
internal class Program
{
static void Main(string[] args)
{
for (int i=0; i<=2;i++) {
GetUserInfo();//第一个用户
}
Console.ReadKey();
}
static string ChangeData(String name1) {
if (name1 == "张三")
{
Console.WriteLine("你输入的是张三");
name1 = "法外狂徒张三";
}
else if (name1 == "李四")
{
Console.WriteLine("你输入的是李四");
name1 = "无情铁手李四";
}
else if (name1 == "王五")
{
Console.WriteLine("你输入的是王五");
name1 = "柔情姐姐王五";
}
else {
Console.WriteLine("你输入的不正确,无法修改");
}
return name1;
}
public static void GetUserInfo() {
Console.WriteLine("这是我的第一个程序");
Console.WriteLine("请输入你的故乡");
string home = Console.ReadLine();
Console.WriteLine("请输入你的名字");
string name = Console.ReadLine();
name=ChangeData(name);
Console.WriteLine("请输入你的年龄");
string age = Console.ReadLine();
Console.WriteLine("请输入你的爱好");
string hobby = Console.ReadLine();
Console.WriteLine("");
Console.WriteLine($"故乡:{home} 名字:{name} 年龄:{age} 爱好:{hobby}");
}
}
}
泛型
功能:查找泛型集合中有几个【张三】
static void Main(string[] args)
{
//泛型集合
List<string> names = new List<string>();
names.Add("张三");
names.Add("李四");
names.Add("王五");
int num = 0;
for (int i = 0; i < names.Count; i++)
{
if (names[i] == "张三")
{
num = num + 1;
}
}
Console.WriteLine(num);
Console.ReadKey();
}
错误处理
(1)不循环的错误处理
static void Main(string[] args)
{
int age = 0;
Console.WriteLine("请输入年龄:");
string input = Console.ReadLine();
try {
age = int.Parse(input);
}
catch {
Console.WriteLine("请输入正确的年龄(数字字符串)!");
return;//捕获到错误后直接return,要不然后面的代码还会执行,错误的打印出10
}
age += 10;
Console.WriteLine("你的年龄是:"+age.ToString());
Console.ReadKey();
}
(2)循环的错误处理,直到控制台输入正确的年龄,跳出循环
static void Main(string[] args)
{
int age = 0;
bool flag = true;
for (; flag; ) {//直到输入正确的年龄,跳出循环
Console.WriteLine("请输入年龄:");
string input = Console.ReadLine();
try
{
age = int.Parse(input);
flag= false;
}
catch
{
Console.WriteLine("请输入正确的年龄(数字字符串)!");
flag = true;//出现年龄错误时一直进入循环,直到输入正确的年龄
}
age += 10;
// Console.WriteLine("你的年龄是:" + age.ToString());及时出错也会进入这一步,年龄是0+10=10
}
Console.WriteLine("请输入姓名!");
string name = Console.ReadLine();
Console.WriteLine("请输入家乡!");
string hometown = Console.ReadLine();
Console.WriteLine($"故乡:{hometown} 名字:{name} 十年后的年龄:{age}");
Console.ReadKey();
}
面向对象
基本属性
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("锄禾日当午!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("汗滴禾想吐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("谁知盘中餐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("粒粒皆辛苦!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("这位农民工是谁?");
string name=Console.ReadLine();
Console.WriteLine("这位农民原来是--->" + name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位农民的基本信息,技能及伤害
string sex = "男";
int age = 22;
int high = 180;
int shanghai = 100;
int xueliang = 100;
string jineng1 = "飞檐走壁";
int jinneg1shanghai = 20;
string jineng2 = "飞沙走石";
int jinneg2shanghai = 30;
Console.WriteLine("性别:"+sex);
Console.WriteLine("年龄:"+age);
Console.WriteLine("身高:"+high);
Console.WriteLine("基础伤害:"+shanghai);
Console.WriteLine("基础血量:"+xueliang);
Console.WriteLine("技能1伤害:"+jinneg1shanghai);
Console.WriteLine("技能2伤害:"+jinneg2shanghai);
Console.ReadKey();
// Console.WriteLine("基础伤害:");
Console.WriteLine("此时远处传来一个粗犷的声音");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("走进一看是一位警察");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("这个警察的名称");
string jingchaname = Console.ReadLine();
Console.WriteLine("这位警察原来是--->" + jingchaname);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位警察的基本信息,技能及伤害
string sex2 = "男";
int age2 = 22;
int high2 = 180;
int shanghai2 = 100;
int xueliang2 = 100;
string jineng11 = "跆拳道";
int jinneg11shanghai = 20;
string jineng22 = "狙击手";
int jinneg22shanghai = 30;
Console.WriteLine("性别:" + sex2);
Console.WriteLine("年龄:" + age2);
Console.WriteLine("身高:" + high2);
Console.WriteLine("基础伤害:" + shanghai2);
Console.WriteLine("基础血量:" + xueliang2);
Console.WriteLine("技能1伤害:" + jinneg11shanghai);
Console.WriteLine("技能2伤害:" + jinneg22shanghai);
Console.ReadKey();
}
(2)封装类属性后创键的对象
class Hero {
public string name = "";
public string sex = "男";
public int age = 22;
public int high = 180;
public int shanghai = 100;
public int xueliang = 100;
public string jineng1 = "飞檐走壁";
public int jinneg1shanghai = 20;
public string jineng2 = "飞沙走石";
public int jinneg2shanghai = 30;
}
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("锄禾日当午!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("汗滴禾想吐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("谁知盘中餐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("粒粒皆辛苦!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("这位农民工是谁?");
Hero hr1=new Hero();
hr1.name =Console.ReadLine();
Console.WriteLine("这位农民原来是--->" + hr1.name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位农民的基本信息,技能及伤害
hr1.sex = "男";
hr1.age = 22;
hr1.high = 180;
hr1.shanghai = 100;
hr1.xueliang = 100;
hr1.jineng1 = "飞檐走壁";
hr1.jinneg1shanghai = 20;
hr1.jineng2 = "飞沙走石";
hr1.jinneg2shanghai = 30;
Console.WriteLine("性别:"+ hr1.sex);
Console.WriteLine("年龄:"+ hr1.age);
Console.WriteLine("身高:"+ hr1.high);
Console.WriteLine("基础伤害:"+ hr1.shanghai);
Console.WriteLine("基础血量:"+ hr1.xueliang);
Console.WriteLine("技能1伤害:"+ hr1.jinneg1shanghai);
Console.WriteLine("技能2伤害:"+ hr1.jinneg2shanghai);
Console.ReadKey();
// Console.WriteLine("基础伤害:");
Console.WriteLine("此时远处传来一个粗犷的声音");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("走进一看是一位警察");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("这个警察的名称");
Hero hr2 = new Hero();
hr2.name = Console.ReadLine();
Console.WriteLine("这位警察原来是--->" + hr2.name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位警察的基本信息,技能及伤害
hr2.sex= "男";
hr2.age = 22;
hr2.high = 180;
hr2.shanghai = 100;
hr2.xueliang= 100;
hr2.jineng1 = "跆拳道";
hr2.jinneg1shanghai = 20;
hr2.jineng2 = "狙击手";
hr2.jinneg2shanghai = 30;
Console.WriteLine("性别:" + hr2.sex);
Console.WriteLine("年龄:" + hr2.age);
Console.WriteLine("身高:" + hr2.high);
Console.WriteLine("基础伤害:" + hr2.shanghai);
Console.WriteLine("基础血量:" + hr2.xueliang);
Console.WriteLine("技能1伤害:" + hr2.jinneg1shanghai);
Console.WriteLine("技能2伤害:" + hr2.jinneg2shanghai);
Console.ReadKey();
}
(3)封装到hero类中,并定义showInfo方法
class Hero {
public string name = "";
public string sex = "男";
public int age = 22;
public int high = 180;
public int shanghai = 100;
public int xueliang = 100;
public string jineng1 = "飞檐走壁";
public int jinneg1shanghai = 20;
public string jineng2 = "飞沙走石";
public int jinneg2shanghai = 30;
public void showInfo(){
Console.WriteLine("性别:" + sex);
Console.WriteLine("年龄:" + age);
Console.WriteLine("身高:" + high);
Console.WriteLine("基础伤害:" + shanghai);
Console.WriteLine("基础血量:" + xueliang);
Console.WriteLine("技能1伤害:" + jinneg1shanghai);
Console.WriteLine("技能2伤害:" + jinneg2shanghai);
}
}
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("锄禾日当午!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("汗滴禾想吐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("谁知盘中餐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("粒粒皆辛苦!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("这位农民工是谁?");
Hero hr1=new Hero();
hr1.name =Console.ReadLine();
Console.WriteLine("这位农民原来是--->" + hr1.name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位农民的基本信息,技能及伤害
hr1.sex = "男";
hr1.age = 22;
hr1.high = 180;
hr1.shanghai = 100;
hr1.xueliang = 100;
hr1.jineng1 = "飞檐走壁";
hr1.jinneg1shanghai = 20;
hr1.jineng2 = "飞沙走石";
hr1.jinneg2shanghai = 30;
//Console.WriteLine("性别:" + hr1.sex);
//Console.WriteLine("年龄:" + hr1.age);
//Console.WriteLine("身高:" + hr1.high);
//Console.WriteLine("基础伤害:" + hr1.shanghai);
//Console.WriteLine("基础血量:" + hr1.xueliang);
//Console.WriteLine("技能1伤害:" + hr1.jinneg1shanghai);
//Console.WriteLine("技能2伤害:" + hr1.jinneg2shanghai);
hr1.showInfo();
Console.ReadKey();
// Console.WriteLine("基础伤害:");
Console.WriteLine("此时远处传来一个粗犷的声音");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("走进一看是一位警察");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("这个警察的名称");
Hero hr2 = new Hero();
hr2.name = Console.ReadLine();
Console.WriteLine("这位警察原来是--->" + hr2.name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位警察的基本信息,技能及伤害
hr2.sex= "男";
hr2.age = 22;
hr2.high = 180;
hr2.shanghai = 100;
hr2.xueliang= 100;
hr2.jineng1 = "跆拳道";
hr2.jinneg1shanghai = 20;
hr2.jineng2 = "狙击手";
hr2.jinneg2shanghai = 30;
//Console.WriteLine("性别:" + hr2.sex);
//Console.WriteLine("年龄:" + hr2.age);
//Console.WriteLine("身高:" + hr2.high);
//Console.WriteLine("基础伤害:" + hr2.shanghai);
//Console.WriteLine("基础血量:" + hr2.xueliang);
//Console.WriteLine("技能1伤害:" + hr2.jinneg1shanghai);
//Console.WriteLine("技能2伤害:" + hr2.jinneg2shanghai);
hr2.showInfo();
Console.ReadKey();
}
static的使用
static可以直接使用,不用对类实例化。
定义showInfo类和Hero类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleAppFramework
{
class Hero {
public string name = "";
public string sex = "男";
public int age = 22;
public int high = 180;
public int shanghai = 100;
public int xueliang = 100;
public string jineng1 = "飞檐走壁";
public int jinneg1shanghai = 20;
public string jineng2 = "飞沙走石";
public int jinneg2shanghai = 30;
}
class ShowInfo{
public static void showInfo(Hero hero)
{
Console.WriteLine("性别:" + hero.sex);
Console.WriteLine("年龄:" + hero.age);
Console.WriteLine("身高:" + hero.high);
Console.WriteLine("基础伤害:" + hero.shanghai);
Console.WriteLine("基础血量:" + hero.xueliang);
Console.WriteLine("技能1伤害:" + hero.jinneg1shanghai);
Console.WriteLine("技能2伤害:" + hero.jinneg2shanghai);
}
}
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("锄禾日当午!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("汗滴禾想吐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("谁知盘中餐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("粒粒皆辛苦!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("这位农民工是谁?");
Hero hr1=new Hero();
hr1.name =Console.ReadLine();
Console.WriteLine("这位农民原来是--->" + hr1.name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位农民的基本信息,技能及伤害
hr1.sex = "男";
hr1.age = 22;
hr1.high = 180;
hr1.shanghai = 100;
hr1.xueliang = 100;
hr1.jineng1 = "飞檐走壁";
hr1.jinneg1shanghai = 20;
hr1.jineng2 = "飞沙走石";
hr1.jinneg2shanghai = 30;
ShowInfo.showInfo(hr1);//不需要实例化ShowInfo类
Console.ReadKey();
Console.WriteLine("此时远处传来一个粗犷的声音");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("走进一看是一位警察");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("这个警察的名称");
Hero hr2 = new Hero();
hr2.name = Console.ReadLine();
Console.WriteLine("这位警察原来是--->" + hr2.name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位警察的基本信息,技能及伤害
hr2.sex= "男";
hr2.age = 22;
hr2.high = 180;
hr2.shanghai = 100;
hr2.xueliang= 100;
hr2.jineng1 = "跆拳道";
hr2.jinneg1shanghai = 20;
hr2.jineng2 = "狙击手";
hr2.jinneg2shanghai = 30;
ShowInfo.showInfo(hr1);//不需要实例化ShowInfo类
Console.ReadKey();
}
}
}
把类抽离出来,放到新的文件里【新建了Hero和ShowInfo文件夹】
program.cs
using MyShowInfo;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleAppFramework
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("锄禾日当午!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("汗滴禾想吐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("谁知盘中餐!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("粒粒皆辛苦!");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();//代码暂停,输入任意键继续
Console.WriteLine("这位农民工是谁?");
Hero hr1=new Hero();
hr1.name =Console.ReadLine();
Console.WriteLine("这位农民原来是--->" + hr1.name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位农民的基本信息,技能及伤害
hr1.sex = "男";
hr1.age = 22;
hr1.high = 180;
hr1.shanghai = 100;
hr1.xueliang = 100;
hr1.jineng1 = "飞檐走壁";
hr1.jinneg1shanghai = 20;
hr1.jineng2 = "飞沙走石";
hr1.jinneg2shanghai = 30;
ShowInfo.showInfo(hr1);//不需要实例化ShowInfo类
Console.ReadKey();
Console.WriteLine("此时远处传来一个粗犷的声音");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("走进一看是一位警察");
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
Console.WriteLine("这个警察的名称");
Hero hr2 = new Hero();
hr2.name = Console.ReadLine();
Console.WriteLine("这位警察原来是--->" + hr2.name);
Console.WriteLine("(请按任意键继续)!");
Console.ReadKey();
//这位警察的基本信息,技能及伤害
hr2.sex= "男";
hr2.age = 22;
hr2.high = 180;
hr2.shanghai = 100;
hr2.xueliang= 100;
hr2.jineng1 = "跆拳道";
hr2.jinneg1shanghai = 20;
hr2.jineng2 = "狙击手";
hr2.jinneg2shanghai = 30;
ShowInfo.showInfo(hr1);//不需要实例化ShowInfo类
Console.ReadKey();
}
static string ChangeData(String name1) {
if (name1 == "张三")
{
Console.WriteLine("你输入的是张三");
name1 = "法外狂徒张三";
}
else if (name1 == "李四")
{
Console.WriteLine("你输入的是李四");
name1 = "无情铁手李四";
}
else if (name1 == "王五")
{
Console.WriteLine("你输入的是王五");
name1 = "柔情姐姐王五";
}
else {
Console.WriteLine("你输入的不正确,无法修改");
}
return name1;
}
public static void GetUserInfo() {
Console.WriteLine("这是我的第一个程序");
Console.WriteLine("请输入你的故乡");
string home = Console.ReadLine();
Console.WriteLine("请输入你的名字");
string name = Console.ReadLine();
name=ChangeData(name);
Console.WriteLine("请输入你的年龄");
string age = Console.ReadLine();
Console.WriteLine("请输入你的爱好");
string hobby = Console.ReadLine();
Console.WriteLine("");
Console.WriteLine($"故乡:{home} 名字:{name} 年龄:{age} 爱好:{hobby}");
}
}
}
ShowInfo.cs
using ConsoleAppFramework;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MyShowInfo
{
class ShowInfo
{
public static void showInfo(Hero hero)
{
Console.WriteLine("性别:" + hero.sex);
Console.WriteLine("年龄:" + hero.age);
Console.WriteLine("身高:" + hero.high);
Console.WriteLine("基础伤害:" + hero.shanghai);
Console.WriteLine("基础血量:" + hero.xueliang);
Console.WriteLine("技能1伤害:" + hero.jinneg1shanghai);
Console.WriteLine("技能2伤害:" + hero.jinneg2shanghai);
}
}
}
Hero.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleAppFramework
{
class Hero
{
public string name = "";
public string sex = "男";
public int age = 22;
public int high = 180;
public int shanghai = 100;
public int xueliang = 100;
public string jineng1 = "飞檐走壁";
public int jinneg1shanghai = 20;
public string jineng2 = "飞沙走石";
public int jinneg2shanghai = 30;
}
}
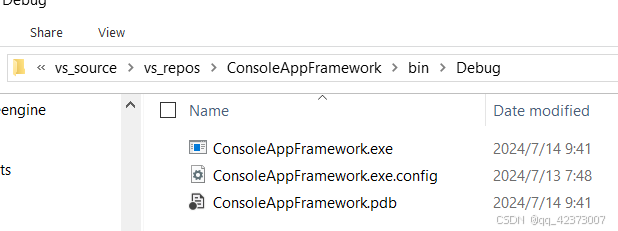
然后在该解决方案add新建一个类库,点击rebuild,会在bin文件夹下生成.dll文件
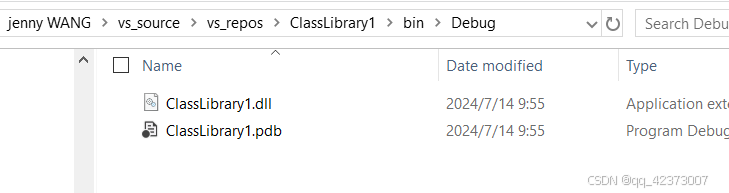
现在解决方案里有ConsoleAppFrameWork和ClassLibrary两个项目,需要把类库中的.dll文件引入进来,然后会在ConsoleAppFrameWork的References下面看到。
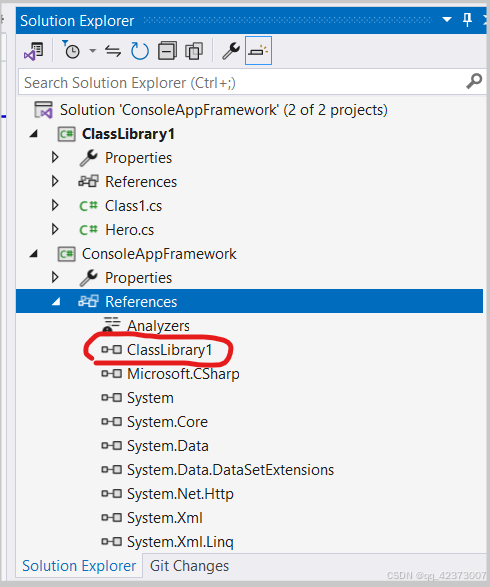
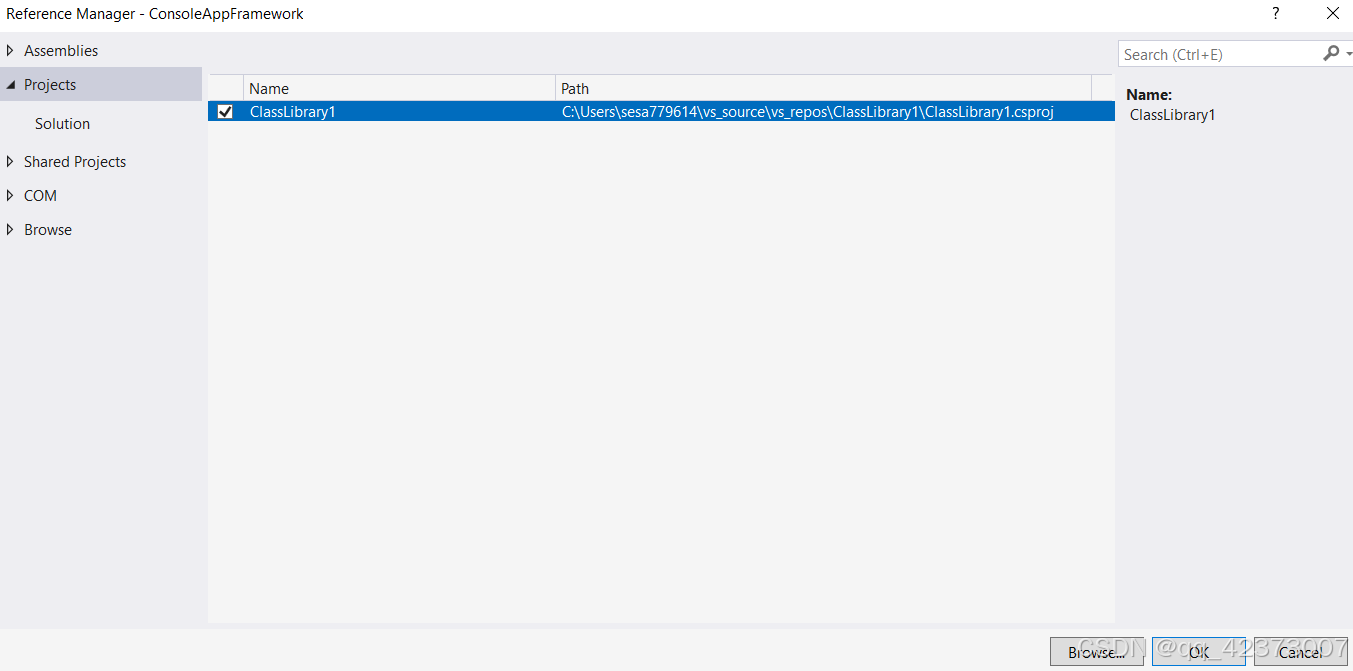
重新启动,将ConsoleAppFrameWork设为启动项,再次执行时多了两个文件classLibrary.cll和pdb文件,【这个是结果】
可能处出现的问题:哪些文件中使用到新建的类库,就在哪些文件里using 类库【类库记得rebuild,.dll文件】
文件读写
删除一个文件
ChangFile/programmer.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ChangeFile
{
internal class Program
{
static void Main(string[] args)
{
string path = "C:/Users/sesa779614/learning";
//获取路径下的所有文件
DirectoryInfo root=new DirectoryInfo(path);
FileInfo[] files= root.GetFiles();
List< FileInfo > filesInfo = files.ToList();
for (int i=0; i<filesInfo.Count;i++) {
FileInfo file = filesInfo[i];
if (file.Name== "Async in C# 5.0.pdf") {
System.IO.File.Delete(file.FullName);
Console.WriteLine("Async in C# 5.0.pdf已经被删除了");
}
// Console.WriteLine(file.FullName);
// Console.WriteLine(file.Name);
}
//string[] files = Directory.GetFiles(path, "*", SearchOption.AllDirectories);
//foreach (string file in files)
//{
// Console.WriteLine(file);
//}
Console.ReadKey();
}
}
}
文件改名
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ChangeFile
{
internal class Program
{
static void Main(string[] args)
{
string path = "C:/Users/sesa779614/learning";
//获取路径下的所有文件
DirectoryInfo root=new DirectoryInfo(path);
FileInfo[] files= root.GetFiles();
List< FileInfo > filesInfo = files.ToList();
for (int i=0; i<filesInfo.Count;i++) {
FileInfo file = filesInfo[i];
string filename = file.Name;
if (filename== "Async in C# 5.0.pdf") {
System.IO.File.Delete(file.FullName);
Console.WriteLine("Async in C# 5.0.pdf已经被删除了");
}
if (filename.Contains("C#")) {
//如果文件名中包含C#,就给文件改名称
string srgFileName=filesInfo[i].FullName;
string destFileName=filesInfo[i].Directory.FullName+"/gaigai"+ filesInfo[i].Extension;//文件夹路径+新的文件名
File.Move(srgFileName,destFileName);
}
Console.WriteLine(filename);
Console.WriteLine(filename.Contains("C#"));
}
//string[] files = Directory.GetFiles(path, "*", SearchOption.AllDirectories);
//foreach (string file in files)
//{
// Console.WriteLine(file);
//}
Console.ReadKey();
}
}
}