加法
export const add = (a, b) => {
a = typeof a === 'number' ? a.toString() : a;
b = typeof b === 'number' ? b.toString() : b;
let [intA = '0', decA = '0'] = a.split('.');
let [intB = '0', decB = '0'] = b.split('.');
decA = decA || '0';
decB = decB || '0';
const maxDecLen = Math.max(decA.length, decB.length);
decA = decA.padEnd(maxDecLen, '0');
decB = decB.padEnd(maxDecLen, '0');
let carry = 0;
let resultDec = '';
for (let i = maxDecLen - 1; i >= 0; i--) {
let sum = parseInt(decA[i]) + parseInt(decB[i]) + carry;
carry = Math.floor(sum / 10);
resultDec = (sum % 10) + resultDec;
}
let resultInt = '';
intA = intA.padStart(Math.max(intA.length, intB.length), '0');
intB = intB.padStart(Math.max(intA.length, intB.length), '0');
for (let i = intA.length - 1; i >= 0; i--) {
let sum = parseInt(intA[i]) + parseInt(intB[i]) + carry;
carry = Math.floor(sum / 10);
resultInt = (sum % 10) + resultInt;
}
if (carry) resultInt = carry + resultInt;
resultInt = resultInt.replace(/^0+/, '');
if (resultInt === '') resultInt = '0';
let result = resultInt + (resultDec !== '0' ? '.' + resultDec.replace(/0+$/, '') : '');
return result;
};
减法
export const subtract = (a, b) => {
a = typeof a === 'number' ? a.toString() : a;
b = typeof b === 'number' ? b.toString() : b;
let [intA = '0', decA = '0'] = a.split('.');
let [intB = '0', decB = '0'] = b.split('.');
decA = decA || '0';
decB = decB || '0';
const maxDecLen = Math.max(decA.length, decB.length);
decA = decA.padEnd(maxDecLen, '0');
decB = decB.padEnd(maxDecLen, '0');
let borrow = 0;
let resultDec = '';
for (let i = maxDecLen - 1; i >= 0; i--) {
let diff = parseInt(decA[i]) - parseInt(decB[i]) - borrow;
if (diff < 0) {
diff += 10;
borrow = 1;
} else {
borrow = 0;
}
resultDec = diff + resultDec;
}
let resultInt = '';
intA = intA.padStart(Math.max(intA.length, intB.length), '0');
intB = intB.padStart(Math.max(intA.length, intB.length), '0');
for (let i = intA.length - 1; i >= 0; i--) {
let diff = parseInt(intA[i]) - parseInt(intB[i]) - borrow;
if (diff < 0) {
diff += 10;
borrow = 1;
} else {
borrow = 0;
}
resultInt = diff + resultInt;
}
resultInt = resultInt.replace(/^0+/, '');
if (resultInt === '') resultInt = '0';
let result = resultInt + (resultDec !== '0' ? '.' + resultDec.replace(/0+$/, '') : '');
return result;
};
乘法
const multiply = (a, b) => {
a = typeof a === 'number' ? a.toString() : a;
b = typeof b === 'number' ? b.toString() : b;
let [intA, decA] = a.split('.');
let [intB, decB] = b.split('.');
decA = decA || '0';
decB = decB || '0';
const totalDecLen = decA.length + decB.length;
const intPartA = intA + decA;
const intPartB = intB + decB;
let result = Array(intPartA.length + intPartB.length).fill(0);
for (let i = intPartA.length - 1; i >= 0; i--) {
for (let j = intPartB.length - 1; j >= 0; j--) {
let product = parseInt(intPartA[i]) * parseInt(intPartB[j]) + result[i + j + 1];
result[i + j + 1] = product % 10;
result[i + j] += Math.floor(product / 10);
}
}
result = result.join('').replace(/^0+/, '');
if (totalDecLen > 0) {
const integerLen = result.length - totalDecLen;
if (integerLen <= 0) {
result = '0.' + '0'.repeat(-integerLen) + result;
} else {
result = result.slice(0, integerLen) + '.' + result.slice(integerLen);
}
result = result.replace(/(\.[0-9]*?)0+$/, '$1').replace(/\.$/, '');
} else {
result = result || '0';
}
return result;
};
除法
export const highPrecisionDivision = (dividend, divisor, precision = 28) => {
const dividendStr = String(dividend);
const divisorStr = String(divisor);
let [dividendInt = '0', dividendDec = ''] = dividendStr.split('.');
let [divisorInt = '0', divisorDec = ''] = divisorStr.split('.');
const maxDecLength = Math.max(dividendDec.length, divisorDec.length, precision);
dividendDec = dividendDec.padEnd(maxDecLength, '0');
divisorDec = divisorDec.padEnd(maxDecLength, '0');
const dividendFull = dividendInt + '.' + dividendDec;
const divisorFull = divisorInt + '.' + divisorDec;
const bigDividend = parseFloat(dividendFull);
const bigDivisor = parseFloat(divisorFull);
let result = (bigDividend / bigDivisor).toFixed(precision);
result = result.replace(/\.?0+$/, '');
return result;
};
add(0.1, "0.3")
add(0.1, 0.3)
add(0.1687486415614614, 0.3)
add("5614", "999999999999999991454444444444444444444444444444")
subtract("5", "3")
subtract(123.45, "67.89")
subtract('561456.514614', "679")
subtract("1000000000000000000000000000000", "1")
multiply("123", "456")
multiply(0.52, "67.89")
multiply(0.548568482, "0.5688974989")
multiply("1000000000000000000000000000000", "1")
highPrecisionDivision(10,2)
highPrecisionDivision(0.456,0.00458)
highPrecisionDivision('0.456',0.08)
highPrecisionDivision(42424,424732545354332543543)
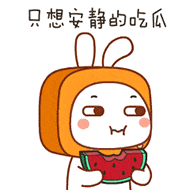
感谢你的阅读,如对你有帮助请收藏+关注!
只分享干货实战和精品,从不啰嗦!!!
如某处不对请留言评论,欢迎指正~
博主可收徒、常玩QQ飞车,可一起来玩玩鸭~