在我学习大模型之前,我一直是一个java哥,现在学习了大模型,我看视频学习,就只知道一个base llm,还有一个是instruction tuned llm,我理解了这些词汇的意义,然后进入了正式学习,那我们正式开始吧!
资源,配置,环境
首先我是通过DeepLearning.AI进行最基础的学习,网址是:[DLAI - Learning Platform ,在这里你可以学习到最基础的知识,里面有内置Jupyter,可以方便读者学习,通过视频的讲解加上自己的编写可以学的更快!
开始
首先我们会有两条对应原则,具体内容我们后面会进行讲解 如果你是在本机运行这些代码,那我们首先需要
pip install openai
当然如果你是在网站用内置jupyter那就不需要管这些问题了
好了让我们来看看这些晦涩难懂的大模型是什么吧
运行
import openai
import os
from dotenv import load_dotenv, find_dotenv
_ = load_dotenv(find_dotenv())
openai.api_key = os.getenv('OPENAI_API_KEY')
这段代码的目的是设置并使用OpenAI的API密钥以访问OpenAI的服务
import openai
import os
这两行代码导入了需要的模块:
openai
模块用于与OpenAI的API进行交互。os
模块用于与操作系统进行交互,特别是用于获取环境变量。
from dotenv import load_dotenv, find_dotenv
_ = load_dotenv(find_dotenv())
这两行代码的作用是加载环境变量:
load_dotenv
和find_dotenv
函数来自dotenv
库,dotenv
库用于读取.env
文件中的环境变量。find_dotenv()
函数会自动找到当前项目中的.env
文件路径。load_dotenv(find_dotenv())
会加载这个文件中的所有环境变量。
openai.api_key = os.getenv('OPENAI_API_KEY')
这行代码从环境变量中获取OpenAI的API密钥并设置它:
os.getenv('OPENAI_API_KEY')
从环境变量中获取名为OPENAI_API_KEY
的值。openai.api_key
将该值设置为OpenAI库使用的API密钥,以便在之后的代码中可以使用OpenAI的API。
这段代码的总体目的是从.env
文件中读取OpenAI的API密钥,并配置OpenAI库以便后续代码可以使用该密钥与OpenAI的服务进行交互。
然后点击下图这个按钮就可以运行然后显示为这个形式就是运行成功
然后我们继续运行后续的代码
def get_completion(prompt, model="gpt-3.5-turbo"):
messages = [{"role": "user", "content": prompt}]
response = openai.ChatCompletion.create(
model=model,
messages=messages,
temperature=0, # this is the degree of randomness of the model's output
)
return response.choices[0].message["content"]
这里我们写了一个辅助函数,何为辅助函数,用简单的话来说就是为了减少代码的冗余,而存在的函数
这里定义了一个名为get_completion
的辅助函数,用于通过OpenAI的gpt-3.5-turbo模型生成聊天补全
def get_completion(prompt, model="gpt-3.5-turbo"):
这行代码定义了一个函数get_completion
,它接受两个参数:
prompt
:这是用户提供的提示信息或问题。model
:这是使用的模型名称,默认为gpt-3.5-turbo
。
messages = [{"role": "user", "content": prompt}]
这行代码创建了一个包含字典的列表messages
,用于向OpenAI API发送请求。字典中有两个键:
"role"
:指定消息的角色,这里是"user"
,表示用户的消息。"content"
:包含实际的提示内容,即用户提供的prompt
。
response = openai.ChatCompletion.create(
model=model,
messages=messages,
temperature=0, # this is the degree of randomness of the model's output
)
这段代码调用OpenAI的ChatCompletion.create
方法生成聊天补全。传递的参数包括:
model
:使用的模型名称,这里使用函数参数model
,默认是gpt-3.5-turbo
。messages
:消息列表,这里是包含用户提示的messages
。temperature
:设置为0
,控制模型输出的随机性。温度值越低,输出越确定和一致;温度值越高,输出越随机。
return response.choices[0].message["content"]
这行代码从API的响应中提取并返回生成的消息内容:
response.choices[0].message["content"]
:从响应中提取第一个选择的消息内容,并将其返回。 总体来说,这个函数的作用是:
- 接收用户提供的提示信息。
- 调用OpenAI的gpt-3.5-turbo模型生成响应。
- 返回生成的消息内容。
然后我们就可以运行了
然后下面那个我们可以不用管他,如果这个In [2]显示了,就证明运行成功了
接下来就我们前面提到的两个原则:
- 原则1:编写清晰而具体的指令
- 原则2:给模型时间进行“思考” 然后我们运行这个后面的代码:
text = f"""
You should express what you want a model to do by \
providing instructions that are as clear and \
specific as you can possibly make them. \
This will guide the model towards the desired output, \
and reduce the chances of receiving irrelevant \
or incorrect responses. Don't confuse writing a \
clear prompt with writing a short prompt. \
In many cases, longer prompts provide more clarity \
and context for the model, which can lead to \
more detailed and relevant outputs.
"""
prompt = f"""
Summarize the text delimited by triple backticks \
into a single sentence.
```{text}```
"""
response = get_completion(prompt)
print(response)
这里定义了一个包含多行文本的字符串,并使用OpenAI的get_completion
函数生成一个总结。
text = f"""
You should express what you want a model to do by \
providing instructions that are as clear and \
specific as you can possibly make them. \
This will guide the model towards the desired output, \
and reduce the chances of receiving irrelevant \
or incorrect responses. Don't confuse writing a \
clear prompt with writing a short prompt. \
In many cases, longer prompts provide more clarity \
and context for the model, which can lead to \
more detailed and relevant outputs.
"""
这段代码使用三重引号定义了一个多行字符串text
。其中的\
用于在行尾进行换行,使代码更易读。这个字符串包含了关于如何编写有效提示的信息,强调了清晰和具体的重要性。
prompt = f"""
Summarize the text delimited by triple backticks \
into a single sentence.
```{text}```
"""
这段代码构建了一个新的字符串prompt
,该字符串是一个提示,要求模型总结用三重反引号括起来的文本。使用了f
字符串格式化方法,将前面定义的text
嵌入到提示中。生成的提示内容如下:
Summarize the text delimited by triple backticks
into a single sentence.
```You should express what you want a model to do by providing instructions that are as clear and specific as you can possibly make them. This will guide the model towards the desired output, and reduce the chances of receiving irrelevant or incorrect responses. Don't confuse writing a clear prompt with writing a short prompt. In many cases, longer prompts provide more clarity and context for the model, which can lead to more detailed and relevant outputs.```
response = get_completion(prompt)
这行代码调用了前面定义的get_completion
函数,传入构建的prompt
作为参数。该函数使用OpenAI的API生成对提示的响应。
print(response)
这行代码将API的响应打印出来,即模型对提示生成的总结。 总结起来,这段代码的作用是:
- 定义一段关于如何编写有效提示的文本。
- 构建一个提示,要求模型对这段文本进行总结。
- 使用OpenAI的模型生成总结并打印输出。
然后我们运行这个代码即可,以下图片是我运行时的结果
这里就可以看到我们通过openai进行了最基础的询问操作
然后就是结构化进行输出
我们为了使传递模型的输出更加容易我们可以让其输出HTML或者是JSON格式
然后我们运行后面这个代码
prompt = f"""
Generate a list of three made-up book titles along \
with their authors and genres.
Provide them in JSON format with the following keys:
book_id, title, author, genre.
"""
response = get_completion(prompt)
print(response)
这里定义了一个提示,要求OpenAI的模型生成三个虚构的书籍标题,并提供相关的作者和体裁信息,然后返回这些数据的JSON格式。
prompt = f"""
Generate a list of three made-up book titles along \
with their authors and genres.
Provide them in JSON format with the following keys:
book_id, title, author, genre.
"""
这段代码使用了多行字符串构建了一个提示字符串prompt
,内容如下:
Generate a list of three made-up book titles along
with their authors and genres.
Provide them in JSON format with the following keys:
book_id, title, author, genre.
具体来说,这个提示的意思是:
- 生成三个虚构的书名。
- 提供每本书的作者和体裁。
- 以JSON格式提供这些信息,并包含以下键:
book_id
,title
,author
,genre
。
response = get_completion(prompt)
这行代码调用了前面定义的get_completion
函数,传入构建的prompt
作为参数。该函数使用OpenAI的API生成对提示的响应。
print(response)
这行代码将API的响应打印出来。模型生成的响应将是一个包含三个虚构书籍信息的JSON格式字符串。 总结起来,这段代码的作用是:
- 构建一个提示,要求模型生成三本虚构书籍的标题、作者和体裁。
- 使用OpenAI的模型生成响应,并以JSON格式提供这些信息。
- 打印生成的响应。
然后我们运行代码
很显然,我们运行成功了,同样确实实现了相应功能
如果我们想要进行对应格式化输出其他的内容我们还可以这样进行,比如下列代码:
text_1 = f"""
Making a cup of tea is easy! First, you need to get some \
water boiling. While that's happening, \
grab a cup and put a tea bag in it. Once the water is \
hot enough, just pour it over the tea bag. \
Let it sit for a bit so the tea can steep. After a \
few minutes, take out the tea bag. If you \
like, you can add some sugar or milk to taste. \
And that's it! You've got yourself a delicious \
cup of tea to enjoy.
"""
prompt = f"""
You will be provided with text delimited by triple quotes.
If it contains a sequence of instructions, \
re-write those instructions in the following format:
Step 1 - ...
Step 2 - …
…
Step N - …
If the text does not contain a sequence of instructions, \
then simply write \"No steps provided.\"
\"\"\"{text_1}\"\"\"
"""
response = get_completion(prompt)
print("Completion for Text 1:")
print(response)
这段代码的目的是处理一段文本,并将其中的指令按步骤格式化
text_1 = f"""
Making a cup of tea is easy! First, you need to get some \
water boiling. While that's happening, \
grab a cup and put a tea bag in it. Once the water is \
hot enough, just pour it over the tea bag. \
Let it sit for a bit so the tea can steep. After a \
few minutes, take out the tea bag. If you \
like, you can add some sugar or milk to taste. \
And that's it! You've got yourself a delicious \
cup of tea to enjoy.
"""
这段代码定义了一个包含多行文本的字符串text_1
,描述了制作一杯茶的步骤。文本中包含了一些换行符\
以保持代码格式的整洁。
prompt = f"""
You will be provided with text delimited by triple quotes.
If it contains a sequence of instructions, \
re-write those instructions in the following format:
Step 1 - ...
Step 2 - …
…
Step N - …
If the text does not contain a sequence of instructions, \
then simply write "No steps provided."
\"\"\"{text_1}\"\"\"
"""
这段代码构建了一个新的提示字符串prompt
,其内容如下:
You will be provided with text delimited by triple quotes.
If it contains a sequence of instructions,
re-write those instructions in the following format:
Step 1 - ...
Step 2 - …
…
Step N - …
If the text does not contain a sequence of instructions,
then simply write "No steps provided."
"""Making a cup of tea is easy! First, you need to get some water boiling. While that's happening, grab a cup and put a tea bag in it. Once the water is hot enough, just pour it over the tea bag. Let it sit for a bit so the tea can steep. After a few minutes, take out the tea bag. If you like, you can add some sugar or milk to taste. And that's it! You've got yourself a delicious cup of tea to enjoy."""
这个提示要求模型将包含在三重引号中的文本重新格式化为分步骤的指令格式。如果文本不包含指令,则输出“No steps provided.”
response = get_completion(prompt)
这行代码调用了前面定义的get_completion
函数,传入构建的prompt
作为参数。该函数使用OpenAI的API生成对提示的响应。
print("Completion for Text 1:")
print(response)
这两行代码打印出模型生成的响应。具体来说,将显示模型如何将给定的制作茶的步骤重新格式化为分步骤的形式。
总结起来,这段代码的作用是:
- 定义一段描述如何制作茶的文本。
- 构建一个提示,要求模型将文本中的指令重新格式化为分步骤的形式。
- 使用OpenAI的模型生成响应并打印输出。
然后我们运行代码
这个就是输出内容
后面我们就开始介绍对应代码了,因为后面几乎都是相同概念
text_2 = f"""
The sun is shining brightly today, and the birds are \
singing. It's a beautiful day to go for a \
walk in the park. The flowers are blooming, and the \
trees are swaying gently in the breeze. People \
are out and about, enjoying the lovely weather. \
Some are having picnics, while others are playing \
games or simply relaxing on the grass. It's a \
perfect day to spend time outdoors and appreciate the \
beauty of nature.
"""
prompt = f"""
You will be provided with text delimited by triple quotes.
If it contains a sequence of instructions, \
re-write those instructions in the following format:
Step 1 - ...
Step 2 - …
…
Step N - …
If the text does not contain a sequence of instructions, \
then simply write \"No steps provided.\"
\"\"\"{text_2}\"\"\"
"""
response = get_completion(prompt)
print("Completion for Text 2:")
print(response)
这段代码定义了一个新的文本,并要求模型判断该文本是否包含指令。如果包含指令,将其重新格式化为步骤形式;如果不包含指令,则输出“No steps provided”。
text_2 = f"""
The sun is shining brightly today, and the birds are \
singing. It's a beautiful day to go for a \
walk in the park. The flowers are blooming, and the \
trees are swaying gently in the breeze. People \
are out and about, enjoying the lovely weather. \
Some are having picnics, while others are playing \
games or simply relaxing on the grass. It's a \
perfect day to spend time outdoors and appreciate the \
beauty of nature.
"""
这段代码定义了一个包含多行文本的字符串text_2
,描述了一个美好的户外场景。文本中使用了换行符\
以保持代码格式的整洁。
prompt = f"""
You will be provided with text delimited by triple quotes.
If it contains a sequence of instructions, \
re-write those instructions in the following format:
Step 1 - ...
Step 2 - …
…
Step N - …
If the text does not contain a sequence of instructions, \
then simply write "No steps provided."
\"\"\"{text_2}\"\"\"
"""
这段代码构建了一个新的提示字符串prompt
,其内容如下:
You will be provided with text delimited by triple quotes.
If it contains a sequence of instructions,
re-write those instructions in the following format:
Step 1 - ...
Step 2 - …
…
Step N - …
If the text does not contain a sequence of instructions,
then simply write "No steps provided."
"""The sun is shining brightly today, and the birds are singing. It's a beautiful day to go for a walk in the park. The flowers are blooming, and the trees are swaying gently in the breeze. People are out and about, enjoying the lovely weather. Some are having picnics, while others are playing games or simply relaxing on the grass. It's a perfect day to spend time outdoors and appreciate the beauty of nature."""
这个提示要求模型将包含在三重引号中的文本重新格式化为分步骤的指令格式。如果文本不包含指令,则输出“No steps provided.”
response = get_completion(prompt)
这行代码调用了前面定义的get_completion
函数,传入构建的prompt
作为参数。该函数使用OpenAI的API生成对提示的响应。
print("Completion for Text 2:")
print(response)
这两行代码打印出模型生成的响应。具体来说,将显示模型判断文本是否包含指令,并根据判断结果输出相应内容。
总结起来,这段代码的作用是:
- 定义一段描述户外美好场景的文本。
- 构建一个提示,要求模型判断文本是否包含指令,并根据判断结果重新格式化或输出“No steps provided.”
- 使用OpenAI的模型生成响应并打印输出。
运行后,我们会发现text2是一段描写美好的风景,并没有指令所以很显然这个就是No steps provided.
先发布一下吧!!! 让后过段时间再更新
那么,我们该如何学习大模型?
作为一名热心肠的互联网老兵,我决定把宝贵的AI知识分享给大家。 至于能学习到多少就看你的学习毅力和能力了 。我已将重要的AI大模型资料包括AI大模型入门学习思维导图、精品AI大模型学习书籍手册、视频教程、实战学习等录播视频免费分享出来。
一、大模型全套的学习路线
学习大型人工智能模型,如GPT-3、BERT或任何其他先进的神经网络模型,需要系统的方法和持续的努力。既然要系统的学习大模型,那么学习路线是必不可少的,下面的这份路线能帮助你快速梳理知识,形成自己的体系。
L1级别:AI大模型时代的华丽登场
L2级别:AI大模型API应用开发工程
L3级别:大模型应用架构进阶实践
L4级别:大模型微调与私有化部署
一般掌握到第四个级别,市场上大多数岗位都是可以胜任,但要还不是天花板,天花板级别要求更加严格,对于算法和实战是非常苛刻的。建议普通人掌握到L4级别即可。
以上的AI大模型学习路线,不知道为什么发出来就有点糊,高清版可以微信扫描下方CSDN官方认证二维码免费领取【保证100%免费
】
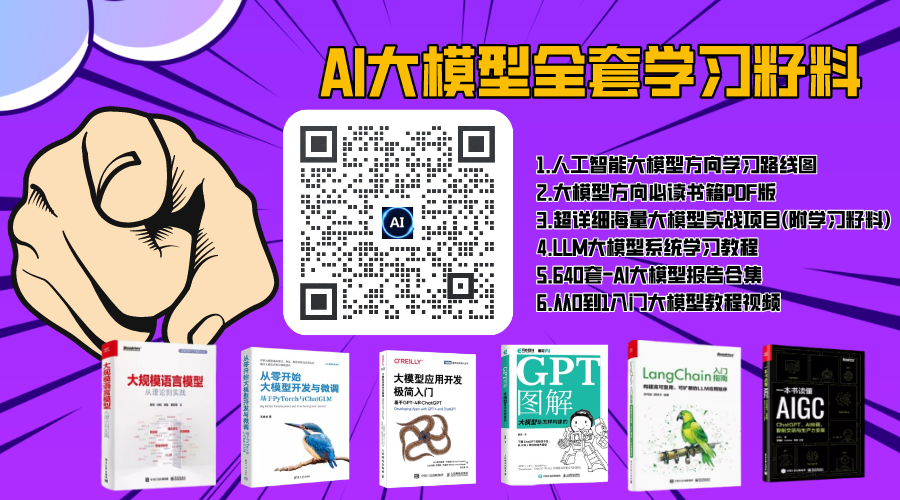
二、640套AI大模型报告合集
这套包含640份报告的合集,涵盖了AI大模型的理论研究、技术实现、行业应用等多个方面。无论您是科研人员、工程师,还是对AI大模型感兴趣的爱好者,这套报告合集都将为您提供宝贵的信息和启示。
三、大模型经典PDF籍
随着人工智能技术的飞速发展,AI大模型已经成为了当今科技领域的一大热点。这些大型预训练模型,如GPT-3、BERT、XLNet等,以其强大的语言理解和生成能力,正在改变我们对人工智能的认识。 那以下这些PDF籍就是非常不错的学习资源。
四、AI大模型商业化落地方案
作为普通人,入局大模型时代需要持续学习和实践,不断提高自己的技能和认知水平,同时也需要有责任感和伦理意识,为人工智能的健康发展贡献力量。