1.关于deque容器说明
deque容器与vector容器差不多,但deque是双端开口容器,可以在两端插入和删除元素
push_front( )//在头部插入
push_back( )//在尾部插入
pop_front( )//在头部删除
pop_back( ) //在尾部删除
其他相应函数与vector差不多,想了解的可以看我写的关于vector单端开口容器基本函数操作https://mp.csdn.net/mp_blog/creation/editor/136658972
2.例题: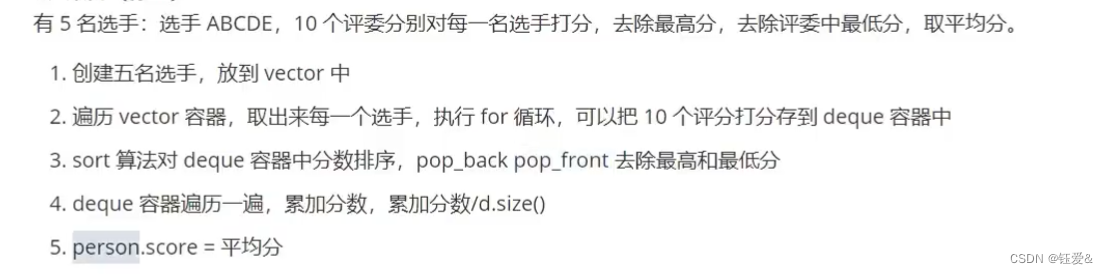
#include<iostream>
#include<vector>
#include<deque>
#include<string>
#include<algorithm>//sort() 头文件
#include<cstdlib> //rand()与srand()头文件
#include<ctime> //time()头文件
#include<numeric>//accumlate()头文件 (求和)
using namespace std;
class student
{
public:
string name;
float score;
public:
student()
{
}
student(string name,float score)
{
this->name=name;
this->score=score;
}
};
void createstudent(vector<student> &v)//创建选手名,刚开使成绩赋为0
{
string a="ABCDE";
for(int i=0;i<5;i++)
{
string name="选手";
name+=a[i];
v.push_back(student(name,0));
}
}
void showstudent(vector<student> &v)//输出选手的名字与成绩
{
vector<student>::iterator it=v.begin();
for( ;it!=v.end();it++)
{
cout<<(*it).name<<" "<<(*it).score<<endl;
}
}
void playgame(vector<student> &v)//比赛得到成绩
{
srand(time(0));//设置随机数种子
vector<student>::iterator it=v.begin();//每人逐渐比赛
for( ;it!=v.end();it++)
{
deque<float> d;//存放评委的评分
for(int i=0;i<10;i++)
{
d.push_back(rand()%41+60);//产生随机数60~100
// printvector(d);
}
//排序
sort(d.begin(),d.end());
//去掉最高分与最低分
d.pop_back();
d.pop_front();
//求平均分
(*it).score=(float)accumulate(d.begin(),d.end(),0)/d.size();
}
}
int main()
{
vector<student> v;
createstudent(v);
playgame(v);
showstudent(v);
return 0;
}
提示:accumulate(d.begin(),d.end(),0) //在区间[d.begin(),d.end()]求和,并在和的基础上加上0,如果后面是100,那就加上100