文章目录
- 1. 核心方法:
- HttpServletRequest 类中
- HttpServletResponse 类中
- HttpSession类中
- Cookie类中
- 2.实现登录界面
Cookie 是浏览器在本地持久化存储数据的一种机制。
Cookie 的数据从哪里来?
是服务器返回给浏览器的。
Cookie 的数据长什么啥样??
Cookie 中是键值对结构的数据,并且这里的键值对都是程序猿自定义的。
Cookie 有什么作用?
Cookie 就是可以在浏览器这边存储一些“临时性数据”。其中最典型的一种使用方法,就是用来存储“身份标识”。
身份标示就是 sessionid ,这里就涉及到 Cookie 和 Session 之间的联动了。Cookie 是在浏览器中存储,Session 是在服务器中存的。为了能存储每个用户的详细信息,这里给每个用户分配一个 sessionid(唯一值)。后续再访问该网站的其他页面,请求中就会带上刚才的 sessionid ,进一步的服务器就可以知道当前是哪个用户在操作。
Cookie 到哪里去了?
Cookie 的内容会在下次访问该网站的时候,自动的被带到 HTTP 请求中。
Cookie 怎么存的??
浏览器按照不同的“域名”,分别存储Cookie。域名和域名之间的 Cookie 是不能干扰的。Cookie 是存储在硬盘上的,而且存储往往会有一个超时的时间。
结合 servlet,进一步的针对 cookie 和 session 进行一些实战操作。
1. 核心方法:
HttpServletRequest 类中
方法 | 描述 |
---|---|
HttpSession getSession() | 在服务器中获取会话。参数如果为true,则当不存在会话时新建会话;反之,不存在会话返回null。 |
Cookie[] getCookies() | 返回一个数组,包含客户端发送该请求的所有Cookie对象。会自动把Cookie中的格式解析成键值对。 |
HttpServletResponse 类中
方法 | 描述 |
---|---|
void addCookie(Cookie cookie) | 把指定的 Cookie 添加到响应中 |
HttpSession类中
方法 | 描述 |
---|---|
Object getAttribute(String name) | 该方法返回在该 session 会话中具有指定名称的对象,如果没有指定名称的对象,则返回 null |
void setAttribute(String name, Object value) | 该方法使用指定的名称绑定一个对象到该 session 会话 |
boolean isNew() | 判定当前是否是新创建出的会话 |
Cookie类中
方法 | 描述 |
---|---|
String getName() | 该方法返回 cookie 的名称。名称在创建后不能改变。(这个值是 SetCooke 字段设置给浏览器的) |
String getValue() | 该方法获取与 cookie 关联的值 |
void setValue(String newValue) | 该方法设置与 cookie 关联的值。 |
//访问 setCookie 请求的时候,代码中就会构造 Cookie 放到响应中
@WebServlet("/setCookie")
public class setCookieServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Cookie cookie = new Cookie("data","2024-3-9");
resp.addCookie(cookie);
Cookie cookie2 = new Cookie("time","16:45");
resp.addCookie(cookie2);
resp.getWriter().write("setCookie ok");
}
}
@WebServlet("/getCookie")
public class getCookieServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Cookie[] cookies = req.getCookies();
if(cookies != null) {
for (Cookie cookie : cookies) {
System.out.println(cookie.getName() + ":" + cookie.getValue());
}
} else {
System.out.println("请求中没有 Cookie");
}
resp.getWriter().write("ok");
}
}
设置完Cookie后浏览器中就可以看到了,后续再次发送,服务器就能拿到 cookie 内容(因为浏览器中已经有了)
2.实现登录界面
这个过程中,感觉到,Cookie 里的数据只是在浏览器暂时歇歇脚。实际上真正发挥作用,还是得在服务器这边的逻辑中生效的。
下面还可以使用 Cookie 结合 Session 实现登录效果。此时就能更清楚的看出来,当前Cookie工作过程了。
Servlet 也提供了Session 相关的支持。实现登录功能,不需要直接使用Cookie api,直接使用 session 的 api 就可以了。
登录页面代码逻辑:
- 获取到用户名和密码
- 验证用户输入的账号密码是否正确
- 如果登录成功则创建对话,在会话中保存自定义的数据。
- 让页面自动跳转
跳转页面代码逻辑:
- 验证用户登录状态( 用getSession(false) )
- 如果验证为登录状态,则根据Session中的内容构造页面
前端简易代码(form表单的形式):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登录</title>
</head>
<body>
<form action="login" method="post">
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="登录">
</form>
</body>
</html>
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
//登录界面
@WebServlet("/login")
public class loginServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//1.获取到用户名和密码
String userName = req.getParameter("username");
String password = req.getParameter("password");
if(userName == null || password == null || userName.equals("") || password.equals("")) {
resp.setContentType("text/html;charset=utf8");
resp.getWriter().write("请求的参数不完整!!");
return;
}
//2.验证用户密码是否正确
// username = 张三,password = 000;
if (!userName.equals("张三")) {
resp.setContentType("text/html;charset=utf8");
resp.getWriter().write("用户名错误");
return;
}
if (!password.equals("000")) {
resp.setContentType("text/html;charset=utf8");
resp.getWriter().write("用户密码错误!!");
return;
}
//3.用户登录成功!! 此时就可以给这个用户创建会话了。
//效果有两方面:(true)
// (1)如果当前用户没有 session,就会创建出 session
// (2)如果已经有了 session,就能够查询到这个session
HttpSession session = req.getSession(true);
//在会话中保存自定义的数据
session.setAttribute("time",System.currentTimeMillis());
session.setAttribute("username",userName);
// 让页面自动跳转
//用servlet生成一个动态页面
resp.sendRedirect("index");
}
}
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
//跳转页面
@WebServlet("/index")
public class indexServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//验证用户登录状态
HttpSession session = req.getSession(false);
if(session == null) {
//用户尚未登录
resp.setContentType("text/html;charset=utf8");
resp.getWriter().write("请先登录,再访问主页!!");
return;
}
//已经登录成功了
String username = (String) session.getAttribute("username");
Long time = (Long) session.getAttribute("time");
System.out.println("username = " + username + "time = " + time);
//根据这样的内容构造主页
resp.setContentType("text/html;charset=utf8");
resp.getWriter().write("欢迎您," + username + "上次登录的时间是:" + time );
}
}
m.out.println("username = " + username + "time = " + time);
//根据这样的内容构造主页
resp.setContentType("text/html;charset=utf8");
resp.getWriter().write("欢迎您," + username + "上次登录的时间是:" + time );
}
}
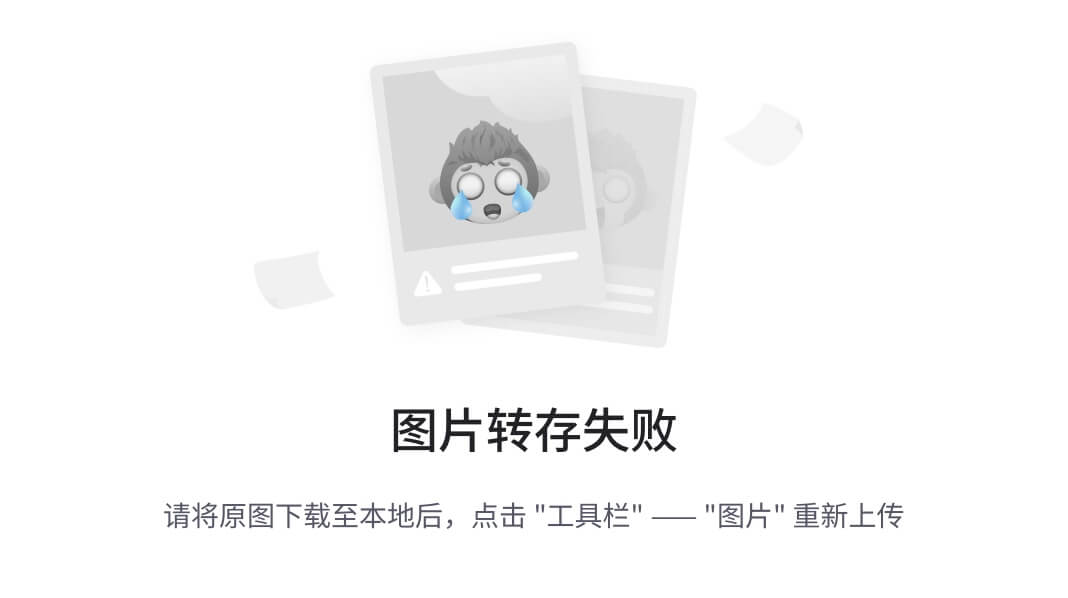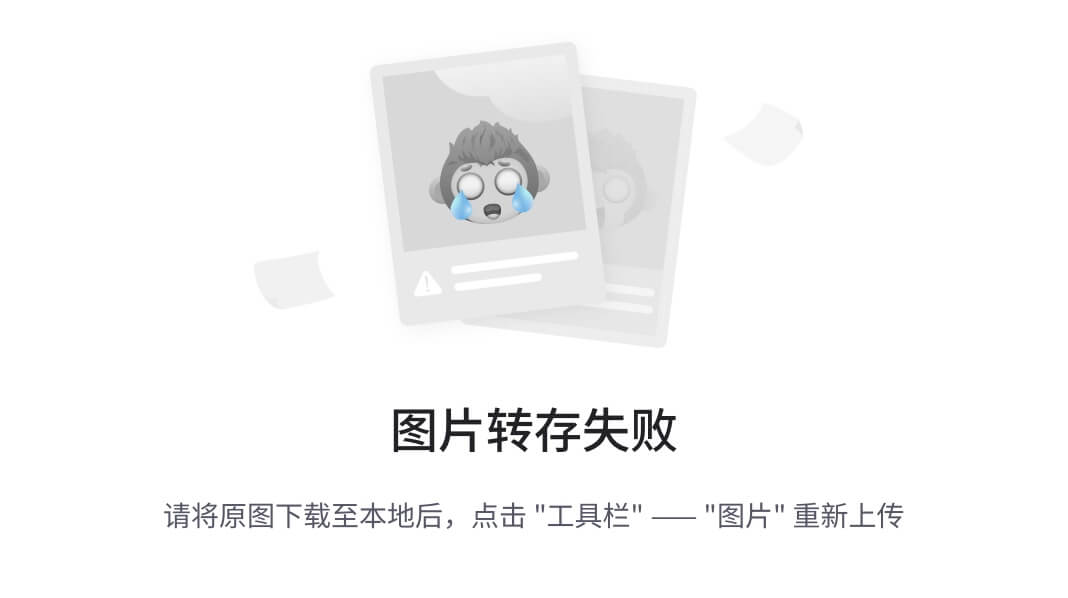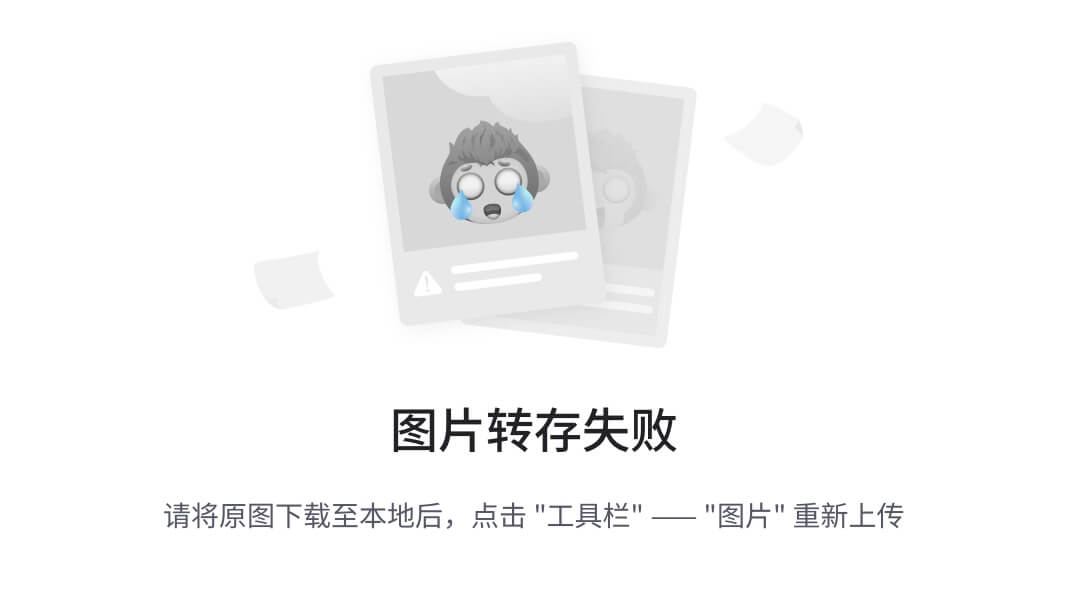