学习目标:
能够使用Requests库发送 get/post/put/delete请求,获取响应状态码、数据能够使用UnitTest管理测试用例。
目录
一、Requests库安装和简介
二、设置http请求语法(重要)
三、应用案例(重要)
1、tpshop搜索:GET
2、tpshop登录:POST
3、ihrm登录:POST
4、修改和删除:PUT、DELETE
一、Requests库安装和简介
- 简介: Requests库 是 Python编写的,基于urllib 的 HTTP库,所以可以发送各种http请求。
- 安装:
pip install requests -i 镜像源
二、设置http请求语法(重要)
resp = requests.请求方法(url='URL地址', params={k:v}, headers={k:v}, data={k:v}, json= {k:v},cookies='cookie数据'(如:令牌))
请求方法:
get请求 - get()
post请求 - post()
put请求 - put()
delete请求 - delete()
- url: 待请求的url - string类型
- params:查询参数 - 字典
- headers:请求头 - 字典
- data:表单格式的 请求体 - 字典
- json:json格式的 请求体 - 字典
- cookies:cookie数据 - string类型
- resp:响应结果
【补充】python中的字符串可以用单引号也可以用双引号包裹,sql语句中的字符串必须用单引号包裹。
入门案例:使用Requests库访问 百度 http://www.baidu.com
三、应用案例(重要)
tpshop前台:
1、tpshop搜索:GET
【带查询参数的get请求】使用Requests库,请求 tpshop商城 搜索商品接口。查询 iphone
准备工作:使用网页的开发者工具抓包获取请求的url(方便写代码的时候复制粘贴)
import requests
# 发送 get 请求,指定 url,获取 响应结果
# 方法一
# resp = requests.get(url="http://demo6.tp-shop.cn/Home/Goods/search.html?q=iphone")
# 方法2
resp = requests.get(url="http://demo6.tp-shop.cn/Home/Goods/search.html",
params={"q":"iphone"})
# 查询响应结果
print(resp.text)
2、tpshop登录:POST
【带表单数据的post请求】使用Requests库,完成 tpshop商城 登录接口调用。返回 ”验证码错误“ 即可。
准备工作:使用网页的开发者工具抓包获取请求的url,注意要输入错误的密码,不然就跳转了,抓不到包。
import requests
# 发送 post 请求,指定url、请求头、请求体, 获取响应结果
resp = requests.post(url="http://demo6.tp-shop.cn/index.php?m=Home&c=User&a=do_login&t=0.27081766186875456",
headers={"content-type":"application/x-www-form-urlencoded; charset=UTF-8"},
json={"username":"188xxxxxxxx","password":"111111","verify_code":"xy8p"})
# 打印响应结果 - 文本
print(resp.text)
# 打印响应结果 - json
print(resp.json())
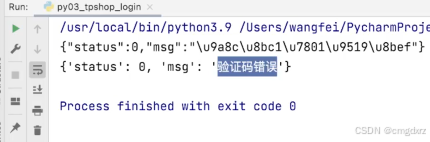
3、ihrm登录:POST
【带 json数据 的post请求】使用Requests库,完成 iHRM系统 成功登录。返回 ”令牌数据“。
import requests
# 发送 post 登录请求,指定 url、请求头、请求体,获取响应结果
resp = requests.post(url="http://ihrm-test.itheima.net/api/sys/login",
# headers={"Content-Type": "application/json"},
json={"mobile": "13800000002", "password": "123456"})
# 打印响应结果
print(resp.json())
#最终结果中的data数据就是令牌

- 如果响应结果是个网页,用text打印结果
- 如果响应结果是个表单或json数据,用json打印结果
4、修改和删除:PUT、DELETE
【发送 put、delete请求】使用Requests库发送 ihrm系统 修改员工信息、删除员工信息 请求。
# -------- 修改 put
import requests
resp = requests.put(url="http://ihrm-test.itheima.net/api/sys/user/1467780995754229760",
headers={"Authorization": "Bearer 4c51c601-c3f7-4d1a-a738-7848f2439f45"},
json={"username": "齐天大圣"})
print(resp.json())
# -------- 删除 delete
import requests
resp = requests.delete(url="http://ihrm-test.itheima.net/api/sys/user/1467780995754229760",
headers={"Authorization": "Bearer 4c51c601-c3f7-4d1a-a738-7848f2439f45"})
print(resp.json())
全部内容:
Requests库01|使用Requests库发送 get/post/put/delete请求-CSDN博客
Requests库02|Cookie和Session-CSDN博客
Requests库03|Unittest框架集成Requests库-CSDN博客