函数
- pygame.font.init
- pygame.font.quit
- pygame.font.get_init
- True,如果字体模块已初始化
- get_init() -> bool
- pygame.font.get_default_font
- 获取默认字体的文件名
- get_default_font() -> string
- pygame.font.get_sdl_ttf_version
- 获取SDL_ttf版本
- get_sdl_ttf_version(linked=True) -> (major, minor, patch)
- pygame.font.get_fonts
- 获取所有可用字体
- get_fonts() -> list of strings
- pygame.font.match_font
- 在系统上查找特定字体
- match_font(name, bold=False, italic=False) -> path
- pygame.font.SysFont
- 从系统字体创建字体对象
- SysFont(name, size, bold=False, italic=False) -> Font
- pygame.font.Font
- 从文件创建新的字体对象
- Font(file_path=None, size=12) -> Font
- Font(file_path, size) -> Font
- Font(pathlib.Path, size) -> Font
- Font(object, size) -> Font
- Font类:
- pygame.font.Font.bold
- 获取或设置字体是否应以粗体呈现。
- bold -> bool
- pygame.font.Font.italic
- 获取或设置字体是否应以假斜体呈现。
- italic -> bool
- pygame.font.Font.underline
- 获取或设置是否应使用下划线呈现字体。
- underline -> bool
- pygame.font.Font.strikethrough
- 获取或设置是否应使用删除线呈现字体。
- strikethrough -> bool
- pygame.font.Font.render
- 绘制文本
- render(text, antialias, color, background=None) -> Surface
- pygame.font.Font.size
- 确定呈现文本所需的空间量
- size(text) -> (width, height)
- pygame.font.Font.set_underline
- 控制是否使用下划线呈现文本
- set_underline(bool) -> None
- pygame.font.Font.get_underline
- 检查文本是否将带有下划线呈现
- get_underline() -> bool
- pygame.font.Font.set_strikethrough
- 控制是否使用删除线呈现文本
- set_strikethrough(bool) -> None
- pygame.font.Font.get_strikethrough
- 检查文本是否会使用删除线呈现
- get_strikethrough() -> bool
- pygame.font.Font.set_bold
- 启用粗体呈现文本
- set_bold(bool) -> None
- pygame.font.Font.get_bold
- 检查文本是否会以粗体显示
- get_bold() -> bool
- pygame.font.Font.set_italic
- 启用斜体呈现文本
- set_italic(bool) -> None
- pygame.font.Font.metrics
- 获取传递的字符串中每个字符的指标
- metrics(text) -> list
- pygame.font.Font.get_italic
- 检查文本是否呈现为斜体
- get_italic() -> bool
- pygame.font.Font.get_linesize
- 获取字体文本的行距
- get_linesize() -> int
- pygame.font.Font.get_height
- 获取字体的高度
- get_height() -> int
- pygame.font.Font.get_ascent
- 获取字体的上升
- get_ascent() -> int
- pygame.font.Font.get_descent
- 获取字体的下降
- get_descent() -> int
- pygame.font.Font.set_script
- 设置文本形状的脚本代码
- set_script(str) -> None
示例
import sys
import pygame
from pygame.locals import *
pygame.init()
screen = pygame.display.set_mode((800, 600), flags=0)
fontinit = pygame.font.get_init()
print('fontinit:', fontinit)
ttf_version = pygame.font.get_sdl_ttf_version()
print('ttf_version:', ttf_version)
fonts = pygame.font.get_fonts()
print('fonts:', fonts)
default_font = pygame.font.get_default_font()
print('default font:', default_font)
match_font = pygame.font.match_font(name='华文行楷', bold=False, italic=False)
print('match font', match_font)
font = pygame.font.Font("C:/Windows/Fonts/simhei.ttf", 50)
text_font = font.render('这是Font创建的文本.', True, (255, 0, 0), (255, 255, 255))
screen.blit(text_font, (100, 100))
print(text_font.get_height())
print(text_font.get_width())
print(text_font.get_rect())
print(text_font.get_bounding_rect())
sysFont = pygame.font.SysFont('幼圆', 25)
sysFont.set_bold(True)
sysFont.set_italic(True)
sysFont.set_strikethrough(True)
text_sysFont = sysFont.render('这是SysFont创建的文本', True, (255, 0, 0), (255, 255, 255))
screen.blit(text_sysFont, (100, 200))
sysFontDefault = pygame.font.SysFont(None, 15)
text_sysFontDefault = sysFontDefault.render('SysFont Default font', True, (0, 255, 0), (0, 0, 255))
screen.blit(text_sysFontDefault, (100, 300))
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
if event.type == MOUSEBUTTONUP:
if event.button == 1:
pass
pygame.display.flip()
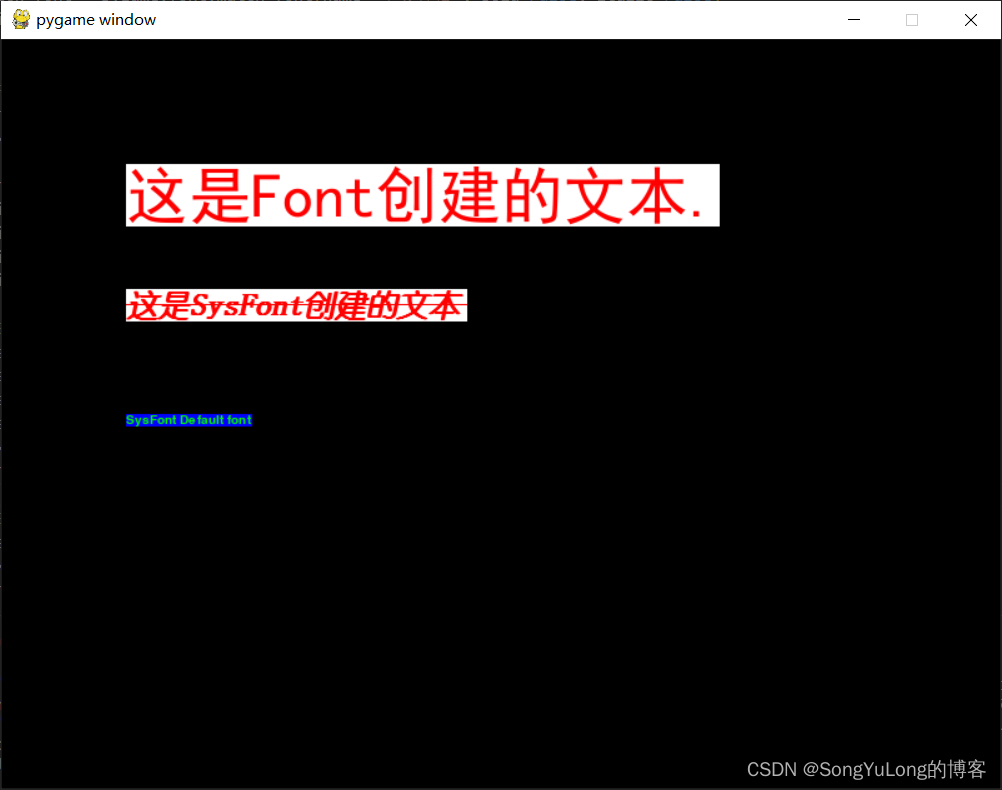