CSDN的各位友友们你们好,今天千泽为大家带来的是
燕山大学-面向对象程序设计实验- 实验三 类和对象 —构造函数与析构函数,
接下来让我们一起进入c++的神奇小世界吧,相信看完你也能写出自己的 实验报告!
如果对您有帮助的话希望能够得到您的支持和帮助,我会持续更新的!
🚀3.1 实验目的
1.理解掌握this指针的作用和用法;
2.理解掌握构造函数的定义和作用;
3.掌握构造函数的使用;
4.理解掌握拷贝构造函数的定义和使用;
5.理解掌握构造函数的重载;
6.理解掌握析构函数的定义和使用。
🚀3.2 实验内容
🚀3.2.1程序阅读
1.理解下面的程序,并在VC++6.0下运行查看结果,回答程序后面的问题。
#include <iostream>
class CPoint
{
public:
void Set(int x,int y);
void Print();
private:
int x;
int y;
};
void CPoint::Set(int x,int y)
{
x = x;
y = y;
}
void CPoint::Print()
{
cout<<"x="<<x<<",y="<<y<<endl;
}
void main()
{
CPoint pt;
pt.Set(10,20);
pt.Print();
}
🚩问题一:以上程序编译能通过吗,试解释该程序?
答:补充声明命名空间using namespace std后,编译可以通过,但结果不正确。程序在CPoint类中对成员变量和成员函数进行了声明,随后在CPoint类外对成员函数Set和Print进行了实现。在main函数中,构CPoint类对象pt,随后调用pt.Set和pt.Print完成了成员变量赋值和输出。
🚩问题二:以上程序的运行结构是否正确,如果不正确,试分析为什么,应该如何改正?
答:运行结果不正确。原因为成员函数Set的形参和成员变量名重名,均为x和y,系统未赋值。输出时的变量值为随机值。需要将Set的形参改一下名字,修改后代码如下:
#include <iostream>
using namespace std;
class CPoint{
public:
void Set(int x,int y);
void Print();
private:
int x;
int y;
};
void CPoint::Set(int _x,int _y){
x = _x;
y = _y;
}
void CPoint::Print(){
cout<<"x="<<x<<",y="<<y<<endl;
}
int main(){
CPoint pt;
pt.Set(10,20);
pt.Print();
}
🚀2.理解下面的程序,并在VC++6.0下运行查看结果,回答程序后面的问题。
#include <iostream>
class CPerson
{
public:
void Print();
private:
CPerson();
private:
int age;
char *name;
};
CPerson::CPerson()
{
}
void CPerson::Print()
{
cout<<"name="<<name<<",age="<<age<<endl;
}
void main()
{
CPerson ps(23,"张三");
ps.Print();
}
🚩问题一:以上程序存在三处大错误,在不改变主函数内容的前提下,试改正该程序。
#include <iostream>
using namespace std;
class CPerson{
public:
void Print();
CPerson(int age,char*name);//构造函数应该为public,且应该有参数
private:
int age;
char *name;
};
CPerson::CPerson(int _age,char*_name){//构造函数需要代码实现
age=_age;
name=_name;
}
void CPerson::Print(){
cout<<"name="<<name<<",age="<<age<<endl;
}
int main(){
CPerson ps(23,"张三");
ps.Print();
}
🚀3.2.2 程序设计
1.设计实现一个CPoint类,满足以下要求:
a. 该类包含两个整型成员变量x(横坐标)和y(纵坐标),以及一个输出函数Print()用来输出横坐标和纵坐标,要求不可以在类的外部直接访问成员变量;
b.可以采用没有参数的构造函数初始化对象,此时的成员变量采用默认值0;
c.可以采用直接输入参数的方式来初始化该类的成员变量;
d.可以采用其它的CPoint对象来初始化该类的成员变量;
e.设计一个主函数来测试以上功能。
答:
#include <iostream>
using namespace std;
class CPoint{
private:
int x;
int y;
public:
void Print(){
cout<<"横坐标为 "<<x<<endl;
cout<<"纵坐标为 "<<y<<endl;
}
CPoint(int _x=0,int _y=0){
x=_x;
y=_y;
}
CPoint(CPoint &a){
x=a.x;
y=a.y;
}
void Set(int _x,int _y){
x=_x;
y=_y;
}
};
int main(){
CPoint c1;//无参数构造函数,默认初始化成员变量值为0
c1.Print();
CPoint c2(1,2);//带参数构造函数初始化成员变量值
c2.Print();
CPoint c3;//使用成员函数Set设置成员变量
c3.Set(3,4);
c3.Print();
CPoint c4(c3);//使用其他CPoint对象初始化成员变量
c4.Print();
return 0;
}
🚀3.3思考题
1.设计一个CStudent(学生)类,并使CStudent类具有以下特点:
a.该类具有学生姓名、学号、程序设计、信号处理、数据结构三门课程的成绩;
b.学生全部信息由键盘输入,以提高程序的适应性;
c.通过成员函数统计学生平均成绩,当课程数量增加时,成员函数无须修改仍可以求取平均成绩;
d.输出学生的基本信息、各科成绩与平均成绩;
e.学生对象的定义采用对象数组实现;
f.统计不及格学生人数。
答:
#include<iostream>
#include<cstring>
#include<string>
#include<cstdlib>
using namespace std;
const int N = 30;
int StuNum;
class CStudent{
private:
string name;
int age;
int courseNum;
double courseScore[10];
string courseName[10];
public:
CStudent(){
}
CStudent(string _name,int _age,int _courseNum,double *_courseScore,string *_courseName){
name=_name;
age=_age;
courseNum=_courseNum;
for(int i=0;i<courseNum;i++){
courseScore[i]=_courseScore[i];
courseName[i]=_courseName[i];
}
}
double getAverage(){
double all=0;
for(int i=0;i<courseNum;i++)
all+=courseScore[i];
return all/courseNum;
}
void printAverage(){
cout<<"Average:"<<getAverage()<<endl;
}
void printScores(){
for(int i=0;i<courseNum;i++)
cout<<courseName[i]<<":"<<courseScore[i]<<endl;
}
void printInfo(){
cout<<"Name:"<<name<<endl;
cout<<"Age:"<<age<<endl;
cout<<"CourseNum:"<<courseNum<<endl;
printScores();
printAverage();
}
int noPassNum(CStudent *stu,int n){
int num=0;
for(int i=0;i<n;i++){
for(int j=0;j<stu[i].courseNum;j++){
if(stu[i].courseScore[j]<60){
num++;
break;
}
}
}
return num;
}
};
int main(){
cout<<"请输入学生数量:";
cin>>StuNum;
CStudent stu[N];
string name;
int age;
int courseNum;
double courseScore[10];
string courseName[10];
for(int i=0;i<StuNum;i++){
memset(courseScore,0,sizeof(courseScore));
for(int j=0;j<10;j++)
courseName[j]="";
cout<<"请输入第"<<i+1<<"位同学的信息"<<endl;
cout<<"请输入姓名、年龄、修读课程数目,以空格分割"<<endl;
cin>>name>>age>>courseNum;
cout<<"请输入修读课程名称和该课程分数,以空格分割。不同课程之间以回车分割。"<<endl;
for(int j=0;j<courseNum;j++){
cin>>courseName[j]>>courseScore[j];
}
stu[i]=CStudent(name,age,courseNum,courseScore,courseName);
}
system("cls");
cout<<"共有"<<StuNum<<"名同学"<<",其中有"<<stu[0].noPassNum(stu,StuNum)<<"名同学不及格!"<<endl;
cout<<"各同学详细信息如下:"<<endl;
cout<<"****************************"<<endl;
for(int i=0;i<StuNum;i++){
stu[i].printInfo();
cout<<"****************************"<<endl;
}
system("pause");
return 0;
}
今天的分享就到这里啦,小伙伴们要多多动手实践练习哦!!!
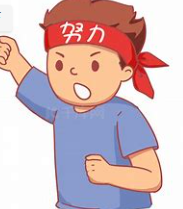