import pyautogui
import cv2
import numpy as np
import math
def find_and_click(template_path, target_x, target_y, match_threshold=0.8):
"""
在屏幕上查找目标图片并点击。
Args:
template_path: 目标图片的路径。
target_x: 预设的坐标 x 轴值。
target_y: 预设的坐标 y 轴值。
match_threshold: 匹配阈值,默认值为 0.8。
Returns:
如果找到目标图片,则返回 True,否则返回 False。
"""
# 加载目标图片
template = cv2.imread(template_path, cv2.IMREAD_GRAYSCALE)
# 获取屏幕截图
screenshot = pyautogui.screenshot()
screenshot = np.array(screenshot)
screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
# 使用模板匹配查找目标图片
result = cv2.matchTemplate(screenshot, template, cv2.TM_CCOEFF_NORMED)
# 获取匹配结果
match_locations = np.where(result >= match_threshold)
# 如果找到匹配结果
if len(match_locations[0]) > 0:
# 获取匹配结果的中心坐标
match_x = match_locations[1][0]
match_y = match_locations[0][0]
# 计算匹配结果与预设坐标的距离
distance = math.sqrt((match_x - target_x)**2 + (match_y - target_y)**2)
# 如果有多个匹配结果,则选择距离最近的进行点击
if len(match_locations[0]) > 1:
for i in range(1, len(match_locations[0])):
x = match_locations[1][i]
y = match_locations[0][i]
new_distance = math.sqrt((x - target_x)**2 + (y - target_y)**2)
if new_distance < distance:
match_x = x
match_y = y
distance = new_distance
# 点击目标图片
pyautogui.click(match_x, match_y)
return True
# 如果没有找到匹配结果
else:
return False
# 示例代码
if __name__ == "__main__":
# 目标图片路径
template_path = "4.png"
# 预设的坐标
target_x = 100
target_y = 100
# 查找并点击目标图片
if find_and_click(template_path, target_x, target_y):
print("目标图片已点击!")
else:
print("未找到目标图片!")
优化代码,输出近似图片的位置坐标
import pyautogui
import cv2
import numpy as np
import math
def find_and_print_matches(template_path, target_x, target_y, match_threshold=0.8):
"""
在屏幕上查找目标图片并打印所有匹配结果的位置。
Args:
template_path: 目标图片的路径。
target_x: 预设的坐标 x 轴值。
target_y: 预设的坐标 y 轴值。
match_threshold: 匹配阈值,默认值为 0.8。
"""
# 加载目标图片
template = cv2.imread(template_path, cv2.IMREAD_GRAYSCALE)
# 获取屏幕截图
screenshot = pyautogui.screenshot()
screenshot = np.array(screenshot)
screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY)
# 使用模板匹配查找目标图片
result = cv2.matchTemplate(screenshot, template, cv2.TM_CCOEFF_NORMED)
# 获取匹配结果
match_locations = np.where(result >= match_threshold)
# 如果找到匹配结果
if len(match_locations[0]) > 0:
# 打印所有匹配结果的位置
print("找到以下匹配结果:")
for i in range(len(match_locations[0])):
match_x = match_locations[1][i]
match_y = match_locations[0][i]
distance = math.sqrt((match_x - target_x)**2 + (match_y - target_y)**2)
print(f"位置:({match_x}, {match_y}),距离:{distance:.2f}")
else:
print("未找到匹配结果!")
# 示例代码
if __name__ == "__main__":
# 目标图片路径
template_path = "4.png"
# 预设的坐标
target_x = 100
target_y = 200
# 查找并打印匹配结果
find_and_print_matches(template_path, target_x, target_y)
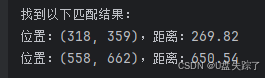