目录
fopen函数
chdir函数
fclose函数
fwrite和fread函数
open函数
umask函数
write函数
read函数
close函数
文件描述符fd
进程访问文件的本质分析
fopen函数
参数mode:
w方式打开文件:1、如果被打开文件不存在,系统会在使用fopen函数的进程的工作目录下创建一个新文件,文件名就是参数path。2、w方式打开文件系统会将文件的内容截断清空。
a方式打开文件:1、如果被打开文件不存在,系统会在使用fopen函数的进程的工作目录下创建一个新文件,文件名就是参数path。2、a方式打开文件,向被打开文件写入内容在有内容的后面续写。
我们来验证一下1、如果被打开文件不存在,系统会在使用fopen函数的进程的工作目录下创建一个新文件,文件名就是参数path。(我们可以通过 ll /proc/进程PID 这一指令查看进程的cwd即进程的目前工作目录来验证;利用chdir函数来改变进程的cwd从而得以验证)。注意:/proc目录中是正在运行的进程
chdir函数
不改变进程的cwd在进程内部使用fopen函数在进程的cwd下创建一个新的文件:
改变进程的cwd:
函数的返回值:
返回被打开文件的地址,类型为FILE*,打开文件失败则返回NULL
fclose函数
fwrite和fread函数
open函数
常见的lags参数:
umask函数
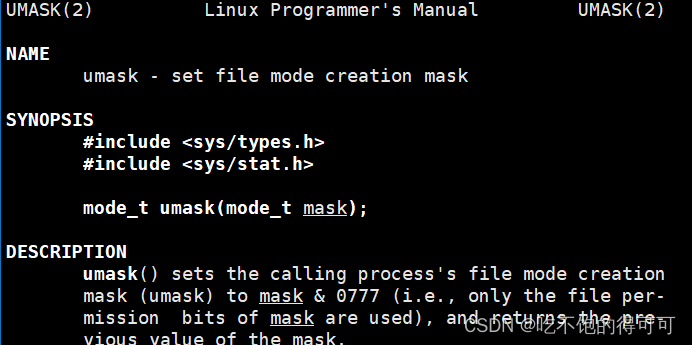
write函数
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
int main()
{
umask(0);
int fd = open("myfile", O_WRONLY|O_CREAT|O_TRUNC, 0644);
if(fd < 0){
perror("open");
return 1;
}
int count = 5;
const char *msg = "hello bit!\n";
int len = strlen(msg);
while(count--){
write(fd, msg, len);//msg:缓冲区首地址 len: 本次读取,期望写入多少个字节的数
据。 返回值:实际写了多少字节数据
}
close(fd);
return 0;
}
read函数
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
int main()
{
int fd = open("myfile", O_RDONLY);
if(fd < 0){
perror("open");
return 1;
}
const char *msg = "hello bit!\n";
char buf[1024];
while(1){
ssize_t s = read(fd, buf, strlen(msg));//类比write
if(s > 0){
printf("%s", buf);
}else{
break;
}
}
close(fd);
return 0;
}
close函数
open、write、read、close函数其实都是对文件操作的系统调用接口。库函数fopen、fread、fwrite、fclose内部都是封装了上面对应的系统接口!
文件描述符fd
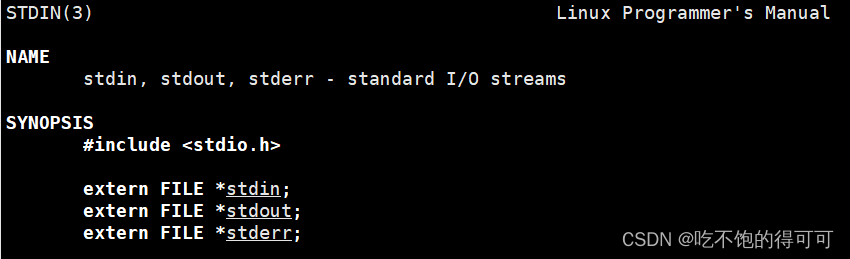
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <string.h>
int main()
{
char buf[1024];
ssize_t s = read(0, buf, sizeof(buf));
if(s > 0){
buf[s] = 0;
write(1, buf, strlen(buf));
write(2, buf, strlen(buf));
}
return 0;
}
进程访问文件的本质分析
1、首先我们来重新认识一下文件:
文件 = 文件内容 + 文件属性 文件分为打开的文件和没有打开的文件。
文件被打开必须要加载到内存里。
而在操作系统中其实是进程打开了文件!-----研究文件打开本质是研究文件和进程之间的关系
没有打开的文件是存放在磁盘上的,磁盘是外部设备,根据冯诺依曼体系访问文件就等于访问硬件,由于操作系统不允许用户直接访问它内部的硬件数据,因此操作系统提供了相应的系统调用供用户对文件进行操作,并且一般都是对文件操作的库函数封装了相应系统调用。例如:fprintf函数是向显示器文件流stdout、stderr中输入内容然后由显示器硬件将内容显示出来。