引入依赖
<dependency>
<groupId>com.github.whvcse</groupId>
<artifactId>easy-captcha</artifactId>
<version>1.6.2</version>
</dependency>
代码实现
package com.qiangesoft.captcha.controller;
import com.wf.captcha.*;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
@RequestMapping("/captcha")
@RestController
public class MyCaptchaController {
public static final int TYPE_DEFAULT = 1;
public static final int TYPE_ONLY_NUMBER = 2;
public static final int TYPE_ONLY_CHAR = 3;
public static final int TYPE_ONLY_UPPER = 4;
public static final int TYPE_ONLY_LOWER = 5;
public static final int TYPE_NUM_AND_UPPER = 6;
@GetMapping("/arithmetic")
public void arithmetic(HttpServletResponse response) throws IOException {
setResponse(response, "arithmetic.jpg");
ArithmeticCaptcha arithmeticCaptcha = new ArithmeticCaptcha(150, 65);
arithmeticCaptcha.setLen(3);
arithmeticCaptcha.out(response.getOutputStream());
}
@GetMapping("/chinese")
public void chinese(HttpServletResponse response) throws IOException {
setResponse(response, "chinese.jpg");
ChineseCaptcha chineseCaptcha = new ChineseCaptcha(150, 65);
chineseCaptcha.setLen(4);
chineseCaptcha.out(response.getOutputStream());
}
@GetMapping("/chineseGif")
public void chineseGif(HttpServletResponse response) throws IOException {
setResponse(response, "chineseGif.gif");
ChineseGifCaptcha chineseGifCaptcha = new ChineseGifCaptcha(150, 65);
chineseGifCaptcha.setLen(4);
chineseGifCaptcha.out(response.getOutputStream());
}
@GetMapping("/gif")
public void gif(HttpServletResponse response) throws IOException {
setResponse(response, "gif.gif");
GifCaptcha gifCaptcha = new GifCaptcha(150, 65);
gifCaptcha.setLen(4);
gifCaptcha.setCharType(TYPE_ONLY_NUMBER);
gifCaptcha.out(response.getOutputStream());
}
@GetMapping("/spec")
public void spec(HttpServletResponse response) throws IOException {
setResponse(response, "spec.jpg");
SpecCaptcha specCaptcha = new SpecCaptcha(150, 65);
specCaptcha.setLen(4);
specCaptcha.out(response.getOutputStream());
}
private void setResponse(HttpServletResponse response, String fileName) {
response.setContentType("application/octet-stream;charset=utf-8");
response.setHeader("content-disposition", "attachment;filename=" + URLEncoder.encode(fileName, StandardCharsets.UTF_8));
}
}
测试
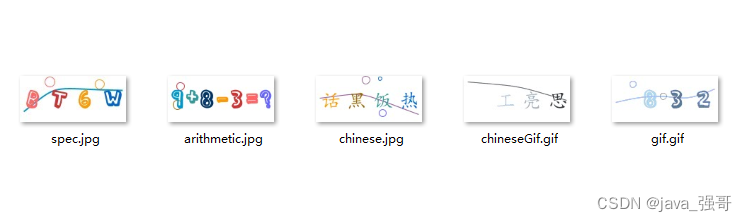