目录
前言
一、 C语言中的类型转换
二、为什么C++需要四种类型转换
三、C++中新增的四种强制类型转换操作符以及它们的应用场景
1.static_cast
2.reinterpret_cast
3.const_cast
4.dynamic_cast
前言
在C语言中,如果赋值运算符左右两侧的类型不同,或者形参与实参类型不同,或者返回值类型与接收返回值类型不同,这几种情况都会发生类型转换。但是C语言的类型转换是由缺陷的,比如:转换的可视性比较差,转换书写的形式单一没有对类型之间的转换进行区别。基于以上原因,C++增添了四种强制类型转换操作符,目的是为了增加类型转换的可视化性。
首先我们先来看一下C语言中的类型转换。
一、 C语言中的类型转换
C语言中的类型转换有两种:隐式类型转换 显示类型转换。
void Test ()
{
int i = 1;
double d = i; // 隐式类型转换
printf("%d, %f\n" , i, d);
int* p = &i;
int address = (int)p; // 显示强制类型转换
printf("%x, %d\n" , p, address);//%x是指以十六进制
}
缺陷:转换的可视性比较差,所有的转换形式都是以一种相同形式书写,难以跟踪错误的转换。
二、为什么C++需要四种类型转换
三、C++中新增的四种强制类型转换操作符以及它们的应用场景
static_cast reinterpret_cast const_cast dynamic_cast
1.static_cast
void Test()
{
double d = 9.8;
int i = static_cast<int>(d);
cout << i << endl;
}
2.reinterpret_cast
void Test()
{
int i = 9;
int* p = reinterpret_cast<int*>(i);
i = reinterpret_cast<int>(p);
cout << (void*)p << endl;
cout << i << endl;
}
3.const_cast
void Test()
{
const int i = 7;
int* p = const_cast<int*>(&i);
*p = 9;
cout << &i << endl;
cout << p << endl;
cout << i << endl;
cout << *p << endl;
}
上面运行结果i打印出来为什么仍然是7而不是9呢?这是因为:
const修饰的变量编译器通常对其进行优化,系统取变量i的值时其实是不在内存中读取的,而是在寄存器中读取,或者是系统直接取常量7的值而不通过内存来读取i的值。
如果要想让系统在内存中读取被const修饰的变量的值,在const前加上关键字volatile即可。加上关键字volatile后的运行结果如下:
4.dynamic_cast
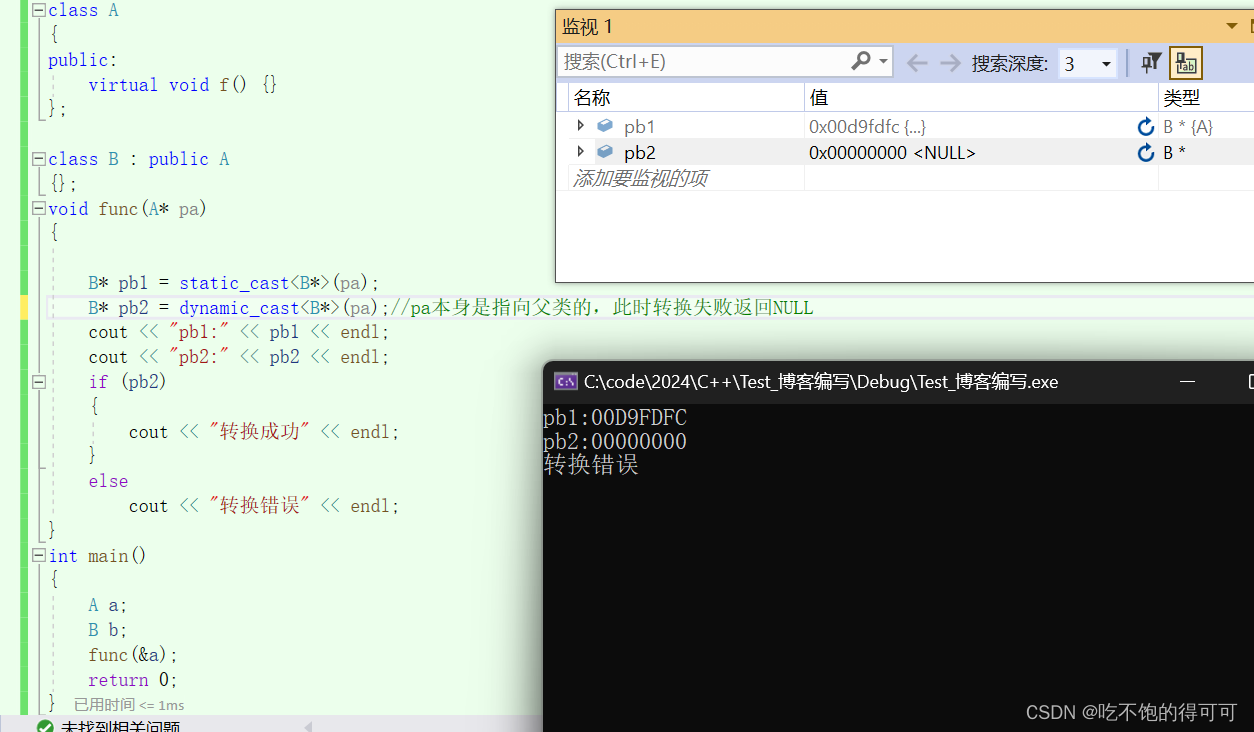