一、实验目的
本次实验的主要目的是通过查看程序的运行结果及实际编写程序,练习使用
Java
语言的继承特性。
二、实验要求
1.
认真阅读实验内容,完成实验内容所设的题目
2.
能够应用多种编辑环境编写
JAVA
语言源程序
3.
认真体会多态与继承的作用
4.
将实验结果书写在实验报告中
三、实验内容
1.
运行下列程序,观察程序的运行结果
A.程序一
package org.example;
public class Main
{
public static void main(String[] arg)
{
Son y=new Son();
System.out.println("a of son is :" +y.a);
System.out.println("b of son is :" +y.b);
y.miner();
y.sminer();
System.out.println("a of son is :" +y.a);
System.out.println("b of son is :" +y.b);
}
}
class Father //父类的定义
{
int a=200;
int b=100;
public void miner()
{
a--;
}
}class Son extends Father //子类的定义
{
public void sminer()
{
b++;
}
}
B程序二
package org.example;
public class Main
{
public static void main(String args[])
{
son id3 = new son();
id3.print();
}
}
class father
{
int a=3;//父类成员变量
public void print()
{
System.out.println("father");
}
}
class son extends father {
float a = 4f;//子类成员变量
public void print() {
int a = 5;//局部变量
super.print();
System.out.println("son");
System.out.println(a);
System.out.println(this.a);
System.out.println(super.a);
}
}
C.程序三
package org.example;
public class Main
{
public static void main(String[] arg)
{
father x=new father();
son y=new son();
System.out.print("add() of father is :");
x.print();
System.out.print("add() of son is :");
y.print();
System.out.println("sub() of son is :" +y.sub());
System.out.println("sub(int i) of son is :" +y.sub(10));
System.out.println("sub(int i,int j) of son is :" +y.sub(10,5));
System.out.println("sub(String s) of son is :" +y.sub("6"));
}
}
class father
{
private int i,j;
father()
{
this.i=23;
this.j=35;
}
protected int add()
{
return i+j;
}
public void print()
{
System.out.println("i+j="+add());
}
}
class son extends father
{
private int x;
son()
{
this.x=25;
} protected int add()//方法被覆盖
{
return x+35;
}
public int sub()//方法的重载
{
return x-15;
}
public int sub(int i)
{
return x-i;
}
public int sub(int i,int j)
{
return x-i-j;
}
public int sub(String s)
{
int f=Integer.parseInt(s);
return x-f;
}
}
D.程序四
package org.example;
public class Main
{
public void useSubAsfather(father x)
{
System.out.println(x.getx()+"!!!!!!");
}
public static void main(String arg[])
{
father superA=new father(),superB;
son subA=new son(),subB;
(new Main()).useSubAsfather(subA);
superB=subA;
System.out.println("superA.getx():"+superB.getx());
subB=(son)superB;
System.out.println(subB.getx()+" "+subB.gety());
}
}
class father
{
private int x=100;
public int getx(){
return x;
}
}
class son extends father
{
private int y=200;
public int gety()
{
return y;
}
}
运行结果如下
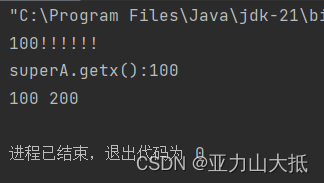
2.查看运行结果,完成下列程序
package org.example;
public class S35 {
private int x;
S35() {
this.setValues(12.5f);
}
private void setValues(float v) {
}
S35(float y) {
this.setValues(y, 10);
}
void setValues(float x, int y) {
this.x = 20;
System.out.println("result is " + (this.x + x + y));
}
public static void main(String[] args) {
S35 s1 = new S35();
}
}
运行结果为:
result is 42.5
3.编写程序实现下列功能
(1) 定义一个矩形类,实现求面积和周长的操作,再定义一个正方形类,要求
其继承于矩形类,并求出正方形的面积,要求显示出计算结果。数据可以用常数。
class Rectangle {
protected int width;
protected int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
public int area() {
return width * height;
}
public int perimeter() {
return 2 * (width + height);
}
}
class Square extends Rectangle {
public Square(int sideLength) {
super(sideLength, sideLength);
}
}
public class Main {
public static void main(String[] args) {
// 创建一个矩形
Rectangle rectangle = new Rectangle(3, 4);
System.out.println("矩形的面积: " + rectangle.area());
System.out.println("矩形的周长: " + rectangle.perimeter());
// 创建一个正方形
Square square = new Square(5);
System.out.println("正方形的面积: " + square.area());
}
}
(2) 设计一个计算完成两数的加、减、乘与除的运算的类。
package org.example;
public class Main {
public static void main(String[] args) {
Main calculator = new Main();
// 两数相加
double additionResult = calculator.add(10, 5);
System.out.println("10 + 5 = " + additionResult);
// 两数相减
double subtractionResult = calculator.subtract(10, 5);
System.out.println("10 - 5 = " + subtractionResult);
// 两数相乘
double multiplicationResult = calculator.multiply(10, 5);
System.out.println("10 * 5 = " + multiplicationResult);
// 两数相除
double divisionResult = calculator.divide(10, 5);
System.out.println("10 / 5 = " + divisionResult);
}
// 加法
public double add(double num1, double num2) {
return num1 + num2;
}
// 减法
public double subtract(double num1, double num2) {
return num1 - num2;
}
// 乘法
public double multiply(double num1, double num2) {
return num1 * num2;
}
// 除法
public double divide(double num1, double num2) {
if (num2 == 0) {
System.out.println("Error: Division by zero");
return Double.NaN;
}
return num1 / num2;
}
}
(3) 编程创建一个 Point 类,在其中定义两个变量表示一个点的坐
值,再定义构造函数初始化为坐标原点,然后定义一个方法实现点的移动,再
定义一个方法打印当前点的坐标。并创建一个对象验
证。
package org.example;
public class Main {
private int x;
private int y;
// 构造函数初始化为坐标原点
public Main() {
this.x = 0;
this.y = 0;
}
// 移动点的方法
public void move(int dx, int dy) {
this.x += dx;
this.y += dy;
}
// 打印当前点的坐标方法
public void printCoordinates() {
System.out.println("Current coordinates: (" + this.x + ", " + this.y + ")");
}
// 验证
public static void main(String[] args) {
Main point = new Main();
point.printCoordinates(); // 输出 (0, 0)
point.move(3, 4);
point.printCoordinates(); // 输出 (3, 4)
}
}
(4)编写一个类,该类有如下一个方法:
public int f(int a,int b){
//要求该方法返回 a 和 b 的最大公约数
}
再编写一个该类的子类,要求子类重写方法 f(),而且重写的方法将返回两
个整数的最小公倍数。
要求:在重写的方法的方法体中首先调用被隐藏的方法返回 a 和 b 的最大公
约数 m,然后将(a*b)/m 返回,在应用程序的主类中分别使用父类和子类创建对
象,并分别调用方法 f()计算两个正整数的最大公约数和最小公倍数。
package org.example;
public class Calculate {
// 方法返回 a 和 b 的最大公约数
public int f(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
}
package org.example;
// 子类,重写父类方法返回两个整数的最小公倍数
public class CalculateLCM extends Calculate {
// 重写 f 方法,返回两个整数的最小公倍数
@Override
public int f(int a, int b) {
int gcd = super.f(a, b); // 调用父类方法返回 a 和 b 的最大公约数
return (a * b) / gcd; // 返回两个整数的最小公倍数
}
}
package org.example;
public class MainApp {
public static void main(String[] args) {
// 使用父类创建对象
Calculate calculate = new Calculate();
int gcd = calculate.f(12, 18);
System.out.println("最大公约数:" + gcd);
// 使用子类创建对象
CalculateLCM calculateLCM = new CalculateLCM();
int lcm = calculateLCM.f(12, 18);
System.out.println("最小公倍数:" + lcm);
}
}
(5)编写一个 Java 应用程序 Test 类,实现成员方法 max(a,b)的重载。具体
要求如下:
a. 编写 void max(int a,int b)成员方法,对两个整数进行大小的比较,输出打
印较大的那个整数。
b.编写 void max(float a,float b)成员方法,对两个 float 数进行大小的比较,
输出打印较大的那个 float 数。
c. 编写 void max(double a,double b)成员方法,对两个 double 数进行大小的
比较,输出打印较大的那个 double 数。
package org.example;
public class Main {
// 对两个整数进行大小的比较
void max(int a, int b) {
if (a > b) {
System.out.println("较大的整数是:" + a);
} else {
System.out.println("较大的整数是:" + b);
}
}
// 对两个float数进行大小的比较
void max(float a, float b) {
if (a > b) {
System.out.println("较大的float数是:" + a);
} else {
System.out.println("较大的float数是:" + b);
}
}
// 对两个double数进行大小的比较
void max(double a, double b) {
if (a > b) {
System.out.println("较大的double数是:" + a);
} else {
System.out.println("较大的double数是:" + b);
}
}
public static void main(String[] args) {
Main test = new Main();
test.max(5, 10);
test.max(3.14f, 2.71f);
test.max(3.1415926, 2.7182818);
}
}
下面是实验总结
继承是面向对象编程中的重要特性,可以帮助我们实现代码的重用和扩展。
子类可以继承父类的属性和方法,同时可以重写父类的方法或者新增自己的方法。
在使用继承时,需要注意父类和子类之间的关系,确保子类是父类的特殊化。
继承可以帮助我们构建更加清晰和易于维护的代码结构。
在实际编写程序时,可以根据具体的需求来设计合适的继承关系,以实现代码的复用和扩展。