感觉比学C那会好了点,不怎么出现照着抄但是就是不能跑的情况,哭死,但是学的顺又不复习,第二天跟没学一样,笑死,要是能给我开个过目不忘的挂,爽的不要不要的
呵呵呵蠢女人,别忘了你C++的作业没做,C的坑还没补
目录
模板
0.1 函数模板
开局BUG:提示对重载函数的调用不明确,是一开始图简单,三个函数名写成了 SWAPINT , SWAPDOUBLE , 模板函数是SWAP改成SWAP(A,B)没用,模板函数名改成MYSWAP报错消失编辑
正确
0.1.1 注意事项
0.1.2 函数模板案例——数组排序
0.1.3 普通函数和函数模板的区别
0.1.4 普通函数和函数模板的调用规则
0.1.5 模板局限性
模板
C++另一种编程思想为泛型编程,主要利用的技术就是模板
两种机制:函数模板,类模板
0.1 函数模板
作用:建立一个通用函数,其函数返回值类型和形参类型可以不具体制定,用一个虚拟的类型来代表
语法:template < typename T>,函数声明或定义
template--声明创建模板;typename--表明其后面的符号是一种数据类型,可以用CLASS代替;T--通用的数据类型,名称可以替换,通常为大写字母
开局BUG:提示对重载函数的调用不明确,是一开始图简单,三个函数名写成了 SWAPINT , SWAPDOUBLE , 模板函数是SWAP
改成SWAP<INT>(A,B)没用,模板函数名改成MYSWAP报错消失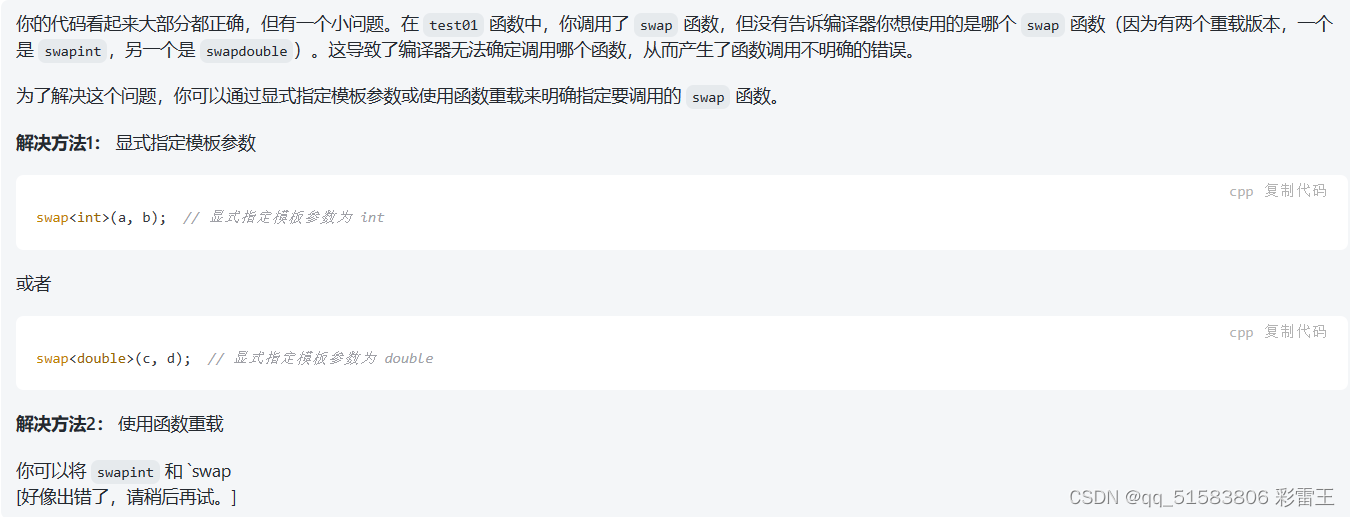
#include<iostream>
using namespace std;
void swapint(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
void swapdouble(double& a, double& b) {
double temp = a;
a = b;
b = temp;
}
//声明一个模板,告诉编译器后面代码中紧跟着的T不要报错,T是一个通用的数据类型
template <typename T>
void swap(T &a, T &b) {
T t= a;
a = b;
b = t;
}
void test01() {
int a = 10; int b = 30;
swapint(a, b);
cout << a << "\t" << b << endl;
double c = 4.333; double d = 231.21;
swapdouble(c, d);
cout << c << "\t" << d << endl;
swap(a, b);
cout << a << "\t" << b << endl;
}
int main()
{
test01();
system("pause");
return 0;
}
正确
#include<iostream>
using namespace std;
void swapint(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
void swapdouble(double& a, double& b) {
double temp = a;
a = b;
b = temp;
}
//声明一个模板,告诉编译器后面代码中紧跟着的T不要报错,T是一个通用的数据类型
template <typename T>
void myswap(T &a, T &b) {
T t= a;
a = b;
b = t;
}
void test01() {
int a = 10; int b = 30;
swapint(a, b);
cout << a << "\t" << b << endl;
double c = 4.333; double d = 231.21;
swapdouble(c, d);
cout << c << "\t" << d << endl;
//自动类型推导
myswap(a, b);
cout << a << "\t" << b << endl;
myswap(c, d);
cout << c << "\t" << d << endl;
//显示指定类型
myswap<int>(a, b);
cout << a << "\t" << b << endl;
myswap<double>(c, d);
cout << c << "\t" << d << endl;
}
int main()
{
test01();
system("pause");
return 0;
}
0.1.1 注意事项
自动类型推导,必须推导出一致的数据类型才可以使用
模板必须要确定出T的数据类型,才可以使用(可以不调用T,但是要确定类型)
#include<iostream>
using namespace std;
template<class T>
void myswap(T& a, T& b) {
T temp = a;
a = b;
b = temp;
}
template<class T>
void func() {
cout << "function 的函数调用" << endl;
}
void test01() {
int a = 0; int b = 77;
double c = 43.23;
char d = 'c';
myswap(a, b);
cout << a << "\t" << b << endl;
//myswap(a, c);错误
//func();错误
func<int>();
func<double>();
func<string>();
func<char>();
}
int main()
{
test01();
system("pause");
return 0;
}
0.1.2 函数模板案例——数组排序
从大到小,排序算法为选择排序,测试CHAR数组,INT数组
函数模板使用调用数组参数时,限定的是数组的单元的数据类型……反正就那意思
#include<iostream>
using namespace std;
template<class T>
void func(T arr[], int len) {//只是用T代替不确定的数据类型,嗯,是不确定的
for (int i = 0; i < len; i++) {
int max=i;
for (int j = i+1; j < len; j++) {
if (arr[max] < arr[j]) {
max = j;
}
}
//arr[i] = max;交换,不是简单的赋值,乐
if (i!= max) {
T temp = arr[i];
arr[i] = arr[max];
arr[max] = temp;
}
}
}
void test01() {
char chararr[] = { "fwsfgwegeh" };
int intarr[] = { 2,43,4,5,3,55,2, };
int lenchar = sizeof(chararr) / sizeof(chararr[0]);
int lenint = sizeof(intarr) / sizeof(intarr[0]);
cout << lenchar << " " << lenint << endl;
for (int i = 0; i < lenchar; i++) {
cout << chararr[i] << " ";
}
cout << endl;
for (int i = 0; i < lenint; i++) {
cout << intarr[i] << " ";
}
cout << endl;
func(chararr, lenchar);
for (int i = 0; i < lenchar; i++) {
cout << chararr[i] << " ";
}
cout << endl;
func(intarr, lenint);
for (int i = 0; i < lenint; i++) {
cout << intarr[i] << " ";
}
cout << endl;
}
int main()
{
test01();
system("pause");
return 0;
}
0.1.3 普通函数和函数模板的区别
普通函数调用时可以发生自动类型转(隐式类型转换)
函数模板调用时,如果利用自动类型推导,不会发生隐式类型转换
如果利用显示指定类型的方式,可以发生隐式类型转换
补:敲代码发现,引用方式接收参数的,都不会发生,暂时时这样的……
#include<iostream>
using namespace std;
int func(int a,int b) {
return a + b;
}
template <class T>
T myadd(T& a, T& b) {
return a + b;
}
template <class T>
T hhmyadd(T a, T b) {
return a + b;
}
void test01() {
int a = 10; int b = 50;
char c = 'a';
cout << func(a, b) << endl;
cout << func(a, c) << endl;//c强制转换成了INT型ACSII码
cout << myadd(a, b) << endl;
//cout << myadd(a, c) << endl;//自动类型推导,不行
//cout << myadd<int>(a, c) << endl;//显示不行
cout << hhmyadd<int>(a, c) << endl;//显示,可以
}
int main()
{
test01();
system("pause");
return 0;
}
建议使用显示指定类型的方式
0.1.4 普通函数和函数模板的调用规则
如果都可以实现,优先调用普通函数
可以通过 空模板参数列表 来强制调用函数模板
函数模板也可以发生重载
如果函数模板可以产生更好的匹配,优先调用函数模板
#include<iostream>
using namespace std;
void func(int a,int b) {
cout << "调用 普通 函数" << endl;
}//如果只给声明,还是调用普通,但是报错
void func(int a, int b,int c) {
cout << "调用 普通chongzai 函数" << endl;
}
template <class T>
void func(T a, T b) {
cout << "调用 模板" << endl;
}
template <class T>
void func(T a, T b,T c) {
cout << "调用 重载 模板" << endl;
}
void test01() {
int a = 10; int b = 50;
int c = 899;
func(a, b);
func<>(a,b);//通过 空模板参数列表 来强制调用函数模板
func(a, b, c);
char c1 = 'a'; char c2 = 'c'; char c3 = 'r';
func(c1, c2, c3);//如果函数模板可以产生更好的匹配,优先调用函数模板
func(a, b, c1);
}
int main()
{
test01();
system("pause");
return 0;
}
0.1.5 模板局限性
传入数组-赋值操作,传入类--比较操作;无法正常运行
解决:模板的重载,可以为特定的(自定义)类型提供具体化的模板;运算符重载【感觉差不多,乐】
学习模板不是为了写模板,是为了在STL能够运用系统提供的模板
#include<iostream>
#include<string>
using namespace std;
class Person {
public:
string m_name;
int m_age;
Person(string name,int age) {
m_name = name;
m_age = age;
}
};
template<class T>
bool mycompare(T& a, T& b) {
if (a == b) {
return true;
}
else {
return false;
}
}
//为特定的类型提供具体化的模板
template<> bool mycompare(Person& a, Person& b) {
if (a.m_name == b.m_name && a.m_age == b.m_age) {
return true;
}
else {
return false;
}
}
void test01() {
int a = 9; int b = 9;
if (mycompare(a, b)) {
cout << "xiangdeng" << endl;
}
else {
cout << " bu bu bu " << endl;
}
}
void test02() {
Person a("张三",12);
Person b("张三", 12);
if (mycompare(a, b)) {
cout << "xiangdeng" << endl;
}
else {
cout << " bu bu bu " << endl;
}
}
int main()
{
test01();
test02();
system("pause");
return 0;
}