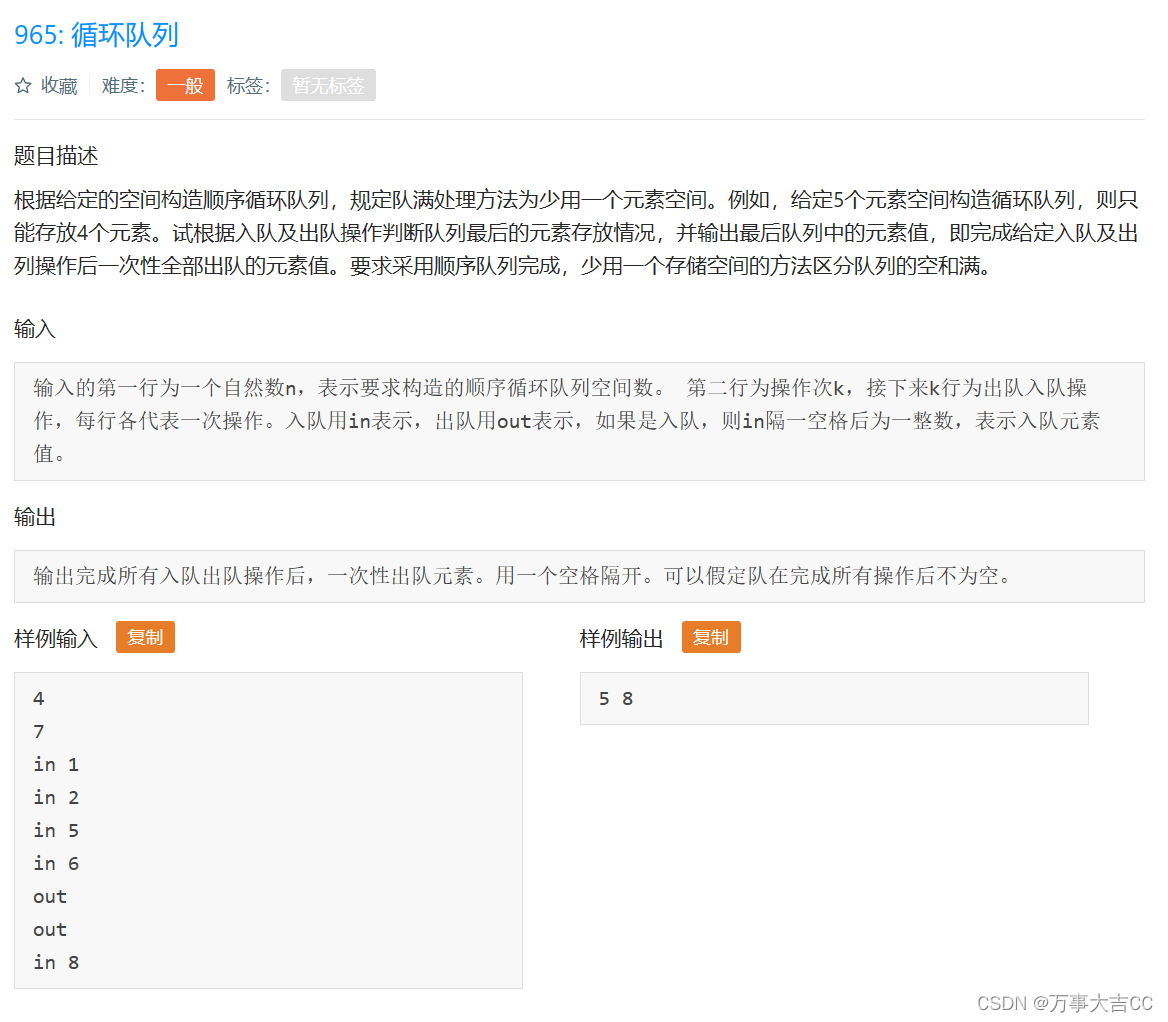
解法:顺序表实现
#include<iostream>
#include<vector>
using namespace std;
struct SeqList {
int* data;
int front;
int rear;
int len;
};
void initList(SeqList* list,int size) {
list->data = new int[size];
list->len = size;
list->front = 0;
list->rear = 0;
return;
}
bool empty(SeqList* list) {
return list->front == list->rear;
}
bool isFull(SeqList* list) {
return (list->rear + 1) % list->len == list->front;
}
void push(SeqList* list,int x) {
if (!isFull(list)) {
list->data[list->rear] = x;
list->rear = (list->rear + 1) % list->len;
}
return;
}
int pop(SeqList* list) {
int t = -1;
if (!empty(list)) {
t = list->data[list->front];
list->front = (list->front + 1) % list->len;
}
return t;
}
int main() {
SeqList l;
int n, k;
cin >> n >> k;
initList(&l,n);
string s;
int a;
while (k--) {
cin >> s;
if (s == "in") {
cin >> a;
push(&l, a);
}
else if (s == "out") {
pop(&l);
}
}
while (!empty(&l)) {
cout << pop(&l) << " ";
}
return 0;
}
解法:链表实现
#include<iostream>
using namespace std;
class Myqueue {
public:
struct Node {
int val;
Node* next;
Node(int x) :val(x), next(NULL) {};
};
Myqueue() {
dummyHead = new Node(0);
tail = dummyHead;
len = 1;
capacity = 0;
}
void initQueue(int size) {
capacity = size;
}
void push(int x) {
if (len < capacity) {
Node* newNode = new Node(x);
tail->next = newNode;
tail = tail->next;
len++;
}
}
int pop() {
if (len > 0) {
int t = dummyHead->next->val;
Node* tmp = dummyHead->next;
dummyHead->next = dummyHead->next->next;
delete tmp;
len--;
return t;
}
return -1;
}
void printList() {
Node* cur = dummyHead->next;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
}
private:
Node* dummyHead;
Node* tail;
int len;
int capacity;
};
int main() {
Myqueue q;
int n, k;
cin >> n >> k;
q.initQueue(n);
string s;
int a;
while (k--) {
cin >> s;
if (s == "in") {
cin >> a;
q.push(a);
}
else if (s == "out") {
q.pop();
}
}
q.printList();
return 0;
}
解法:STL实现
#include<iostream>
#include<queue>
using namespace std;
int main() {
queue<int> q;
int n, k;
cin >> n >> k;
string s;
int a;
while (k--) {
cin >> s;
if (s == "in") {
cin >> a;
if (q.size() + 1 < n) {
q.push(a);
}
}
else if (s == "out") {
if (!q.empty()) {
q.pop();
}
}
}
while (!q.empty()) {
cout << q.front() << " ";
q.pop();
}
return 0;
}