1.实现单击、双击、长按功能
using UnityEngine;
using UnityEngine.Events;
using UnityEngine.EventSystems;
public class ButtonControl_Click_Press_Double : MonoBehaviour, IPointerClickHandler, IPointerDownHandler, IPointerUpHandler, IPointerExitHandler
{
public float pressDurationTime = 1;
public bool responseOnceByPress = false;
public float doubleClickIntervalTime = 0.5f;
public UnityEvent onDoubleClick;
public UnityEvent onPress;
public UnityEvent onClick;
private bool isDown = false;
private bool isPress = false;
private float downTime = 0;
private float clickIntervalTime = 0;
private int clickTimes = 0;
void Update()
{
if (isDown)
{
if (responseOnceByPress && isPress)
{
return;
}
downTime += Time.deltaTime;
if (downTime > pressDurationTime)
{
isPress = true;
onPress.Invoke();
}
}
if (clickTimes >= 1)
{
clickIntervalTime += Time.deltaTime;
if (clickIntervalTime >= doubleClickIntervalTime)
{
if (clickTimes >= 2)
{
onDoubleClick.Invoke();
}
else
{
onClick.Invoke();
}
clickTimes = 0;
clickIntervalTime = 0;
}
}
}
public void OnPointerDown(PointerEventData eventData)
{
isDown = true;
downTime = 0;
}
public void OnPointerUp(PointerEventData eventData)
{
isDown = false;
}
public void OnPointerExit(PointerEventData eventData)
{
isDown = false;
isPress = false;
}
public void OnPointerClick(PointerEventData eventData)
{
if (!isPress)
{
//onClick.Invoke();
clickTimes += 1;
}
else
isPress = false;
}
}
2.使用
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ButtonsControl_1 : MonoBehaviour
{
ButtonControl_Click_Press_Double btn;
void Start()
{
btn = GetComponent<ButtonControl_Click_Press_Double>();
btn.onClick.AddListener(Click);
btn.onPress.AddListener(Press);
btn.onDoubleClick.AddListener(DoubleClick);
}
void Click()
{
Debug.Log("click");
}
void Press()
{
Debug.Log("press");
}
void DoubleClick()
{
Debug.Log("double click");
}
}
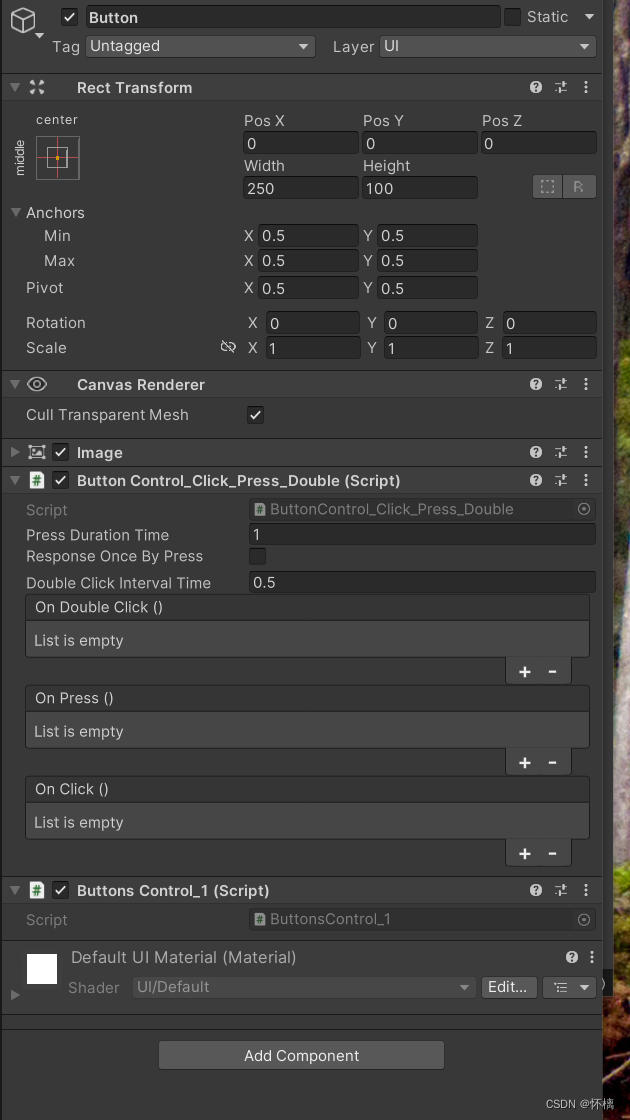