什么是异常
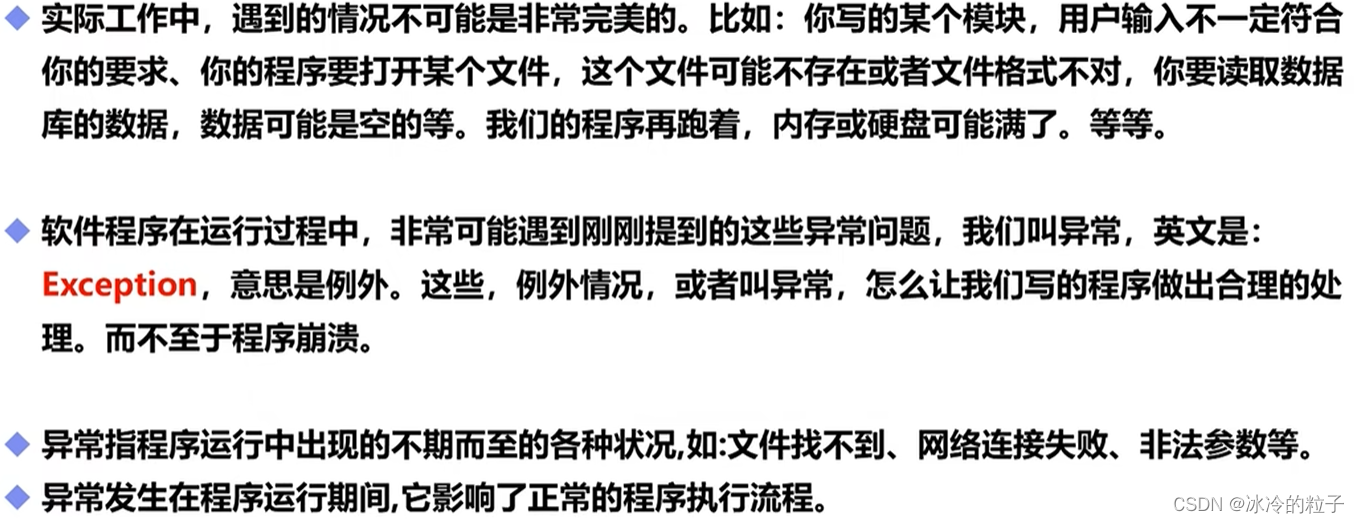
异常体系结构
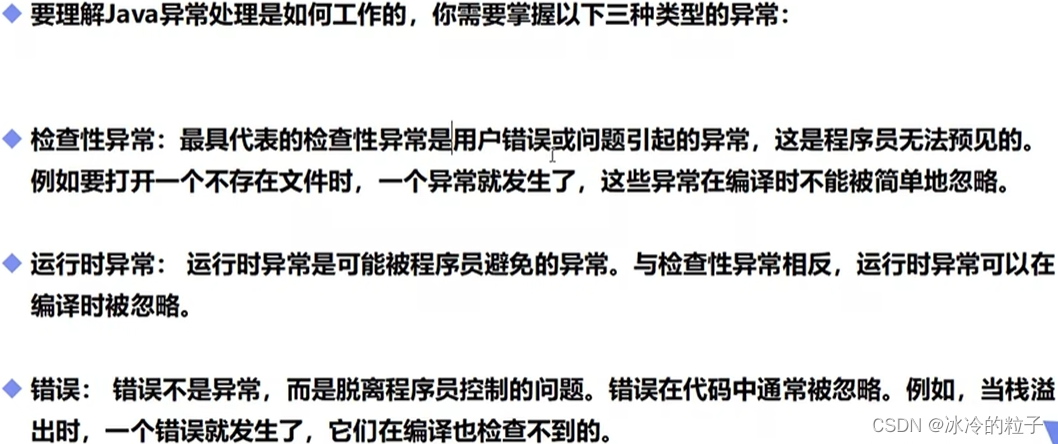
异常的继承关系
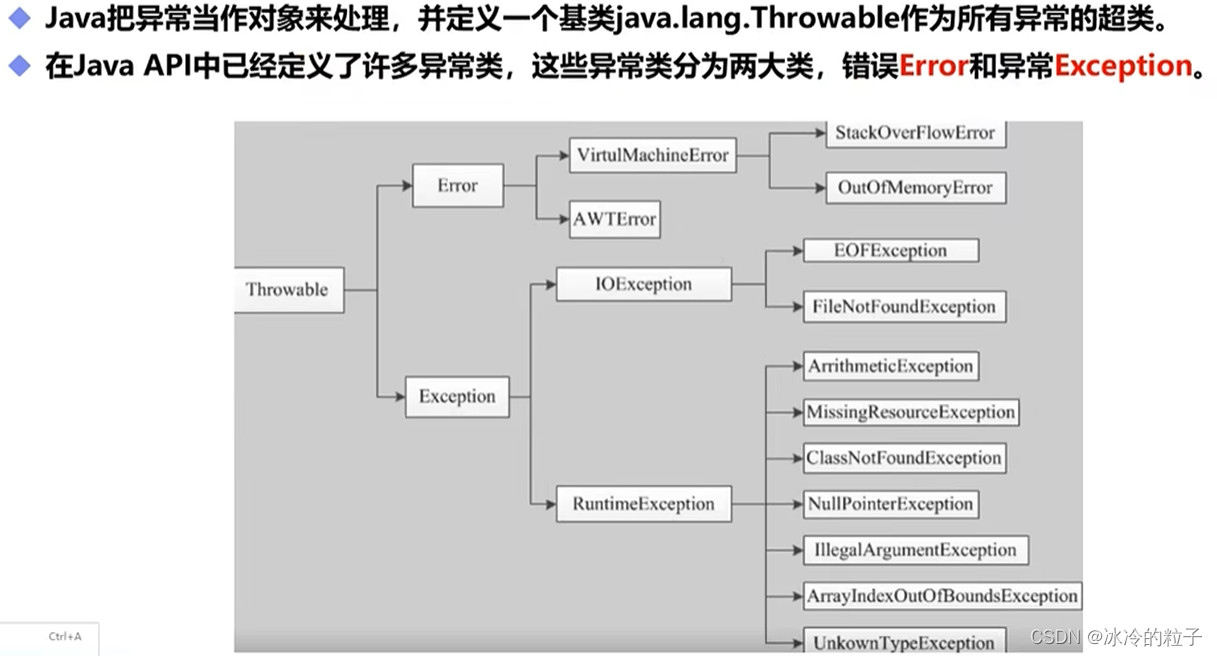
Error
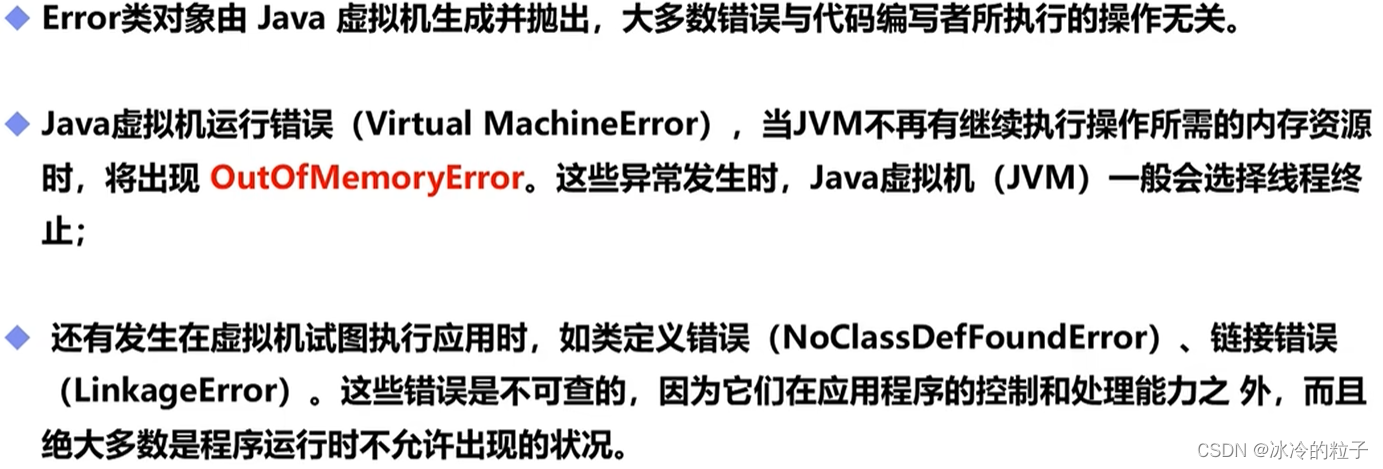
Exception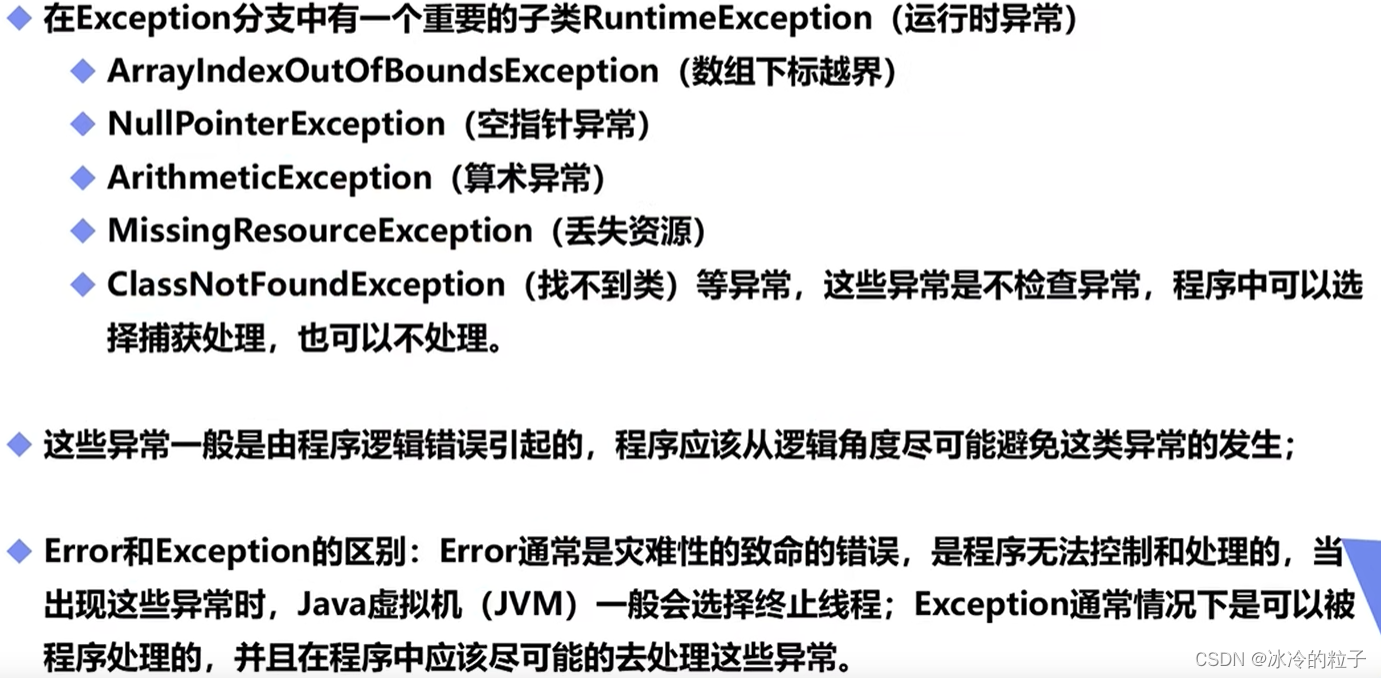
异常处理机制
- try:用{}将可能产生异常的代码包裹
- catch:与try搭配使用,捕获try包裹代码中抛出的异常并进行后续动作
- finally:跟在try后,在try和catch之后执行,可用于关闭资源或后续处理
- throw:手动抛出一个异常
- throws:
处理异常
public class ErrorTest {
public static int division(int a, int b) throws Exception {
if(a % b != 0){
throw new Exception("不好意思除不开");
}
return a / b;
}
}
class TestClass {
public static void main(String[] args) {
int num1 = 4;
int num2 = 3;
int division= 0;
try {
division = ErrorTest.division(num1, num2);
} catch (ArithmeticException e) {
System.out.println("算数异常");
} catch (Exception e) {
System.out.println("产生了异常,关键信息如下:");
e.printStackTrace();
} finally {
System.out.println("进行一些善后工作");
}
System.out.println(division);
}
}
自定义异常
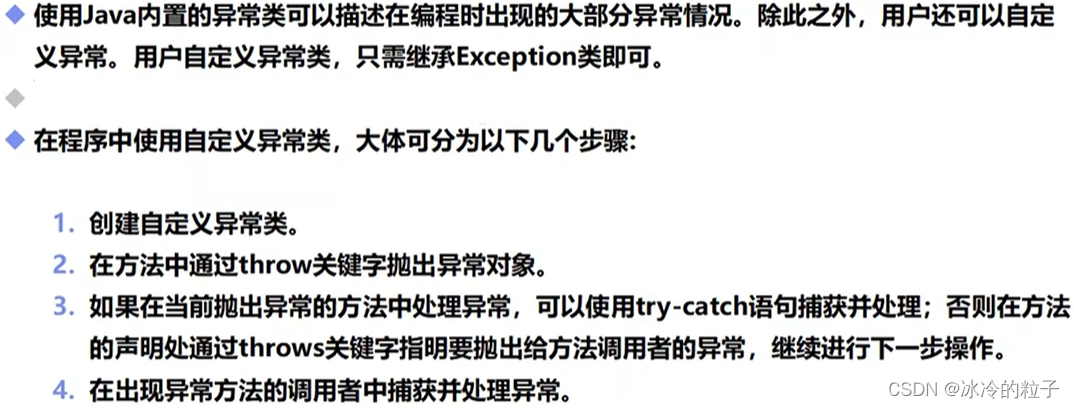
public class ErrorTest {
public static int division(int a, int b) throws Exception {
if(a <= 0){
throw new MyException(a);
}
return a / b;
}
}
class MyException extends Exception{
private int num;
public MyException(int num) {
this.num = num;
}
@Override
public String toString() {
return "MyException{" +
"num=" + num +
'}';
}
}
class TestClass {
public static void main(String[] args) {
int num1 = -4;
int num2 = 2;
try {
ErrorTest.division(num1, num2);
} catch (MyException e) {
System.out.println("产生了异常,关键信息如下:");
e.printStackTrace();
} catch (Exception e) {
System.out.println("产生了异常,关键信息如下:");
e.printStackTrace();
}
}
}
总结
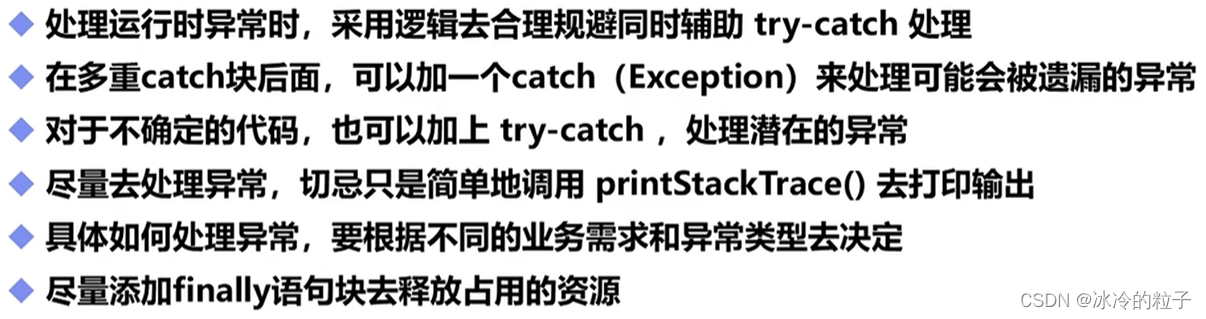