#include<stdio.h>
#include<stdlib.h>
typedef struct Node
{
int data;
struct Node* next;
}Node;
Node* init_single_list()
{
Node* node = (Node*)malloc(sizeof(Node));
node->next = NULL;
node->data = 0;
return node;
}
static int insert_from_head(Node* head,int data)
{
if(!head)
return -1;
Node* tmp_node = (Node*)malloc(sizeof(Node));
tmp_node->data = data;
tmp_node->next = head->next;
head->next = tmp_node;
head->data++;
printf("data of list is: %d\n",head->data);
return 0;
}
static int insert_from_tail(Node* head,int data)
{
if(!head)
return -1;
Node* node = (Node*)malloc(sizeof(Node));
Node* tmp_node = head;
node->data = data;
node->next = NULL;
if (NULL == tmp_node->next)
{
tmp_node->next = node;
printf("in hear\n");
head->data++;
printf("head -> data in 1 = %d\n",head -> data);
return 0;
}
tmp_node = tmp_node->next;
while(tmp_node->next)
{
tmp_node = tmp_node->next;
}
tmp_node->next = node;
head->data++;
printf("head -> data in 2 = %d\n",head -> data);
return 0;
}
static int print_single_list(Node* head)
{
if((!head->next) && (!head))
return -1;
Node* tmp_node = head->next;
while(tmp_node)
{
printf("%d ",tmp_node->data);
tmp_node = tmp_node->next;
}
putchar('\n');
return 0;
}
static int delete_node(Node* head,int data)
{
if((!head->next) && (!head))
return -1;
Node* node = head;
Node* tmp_node = node->next;
while(tmp_node)
{
if (tmp_node->data == data)
{
node->next = tmp_node->next;
free(tmp_node);
printf("head->data start is %d\n",head->data);
head->data--;
printf("head->data end-- is %d\n",head->data);
return 0;
}
node = tmp_node;
tmp_node = tmp_node->next;
}
return -1;
}
static int insert_from_node_tail(Node* head,int num,int data)
{
if((!head->next) && (!head))
return -1;
Node* tmp_node = head;
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
while (tmp_node->next)
{
if (tmp_node->next->data == num)
{
node->next = tmp_node->next->next;
tmp_node->next->next = node;
head->data++;
printf("insert_from_node_tail ok\n");
return 0;
}
tmp_node = tmp_node->next;
}
return -1;
}
static int insert_from_node_head(Node* head,int num,int data)
{
if((!head->next) && (!head))
return -1;
Node* tmp_node = head;
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
while (tmp_node->next)
{
if (tmp_node->next->data == num)
{
node->next = tmp_node->next;
tmp_node->next = node;
head->data++;
printf("insert_from_node_head ok\n");
return 0;
}
tmp_node = tmp_node->next;
}
return -1;
}
int main()
{
Node* head = init_single_list();
int list_d;
int insert_value;
printf("please input data to insert the list and how many you want to insert\n");
scanf("%d",&list_d);
printf("list_d is %d\n",list_d);
for (int i = 1; i <= list_d ; i++)
{
printf("please input insert_value %d:\n",i);
scanf("%d",&insert_value);
insert_from_tail(head,insert_value);
printf("insert_value is %d\n",insert_value);
}
print_single_list(head);
printf("in main_1 head->data is %d\n",head->data);
insert_from_node_tail(head,12,55555);
print_single_list(head);
printf("in main_2 head->data is %d\n",head->data);
insert_from_node_head(head,55555,6666);
print_single_list(head);
printf("in main_3 head->data is %d\n",head->data);
int ret = delete_node(head,12);
if(-1 == ret)
printf("the data is not in the list\n");
else
print_single_list(head);
return 0;
}
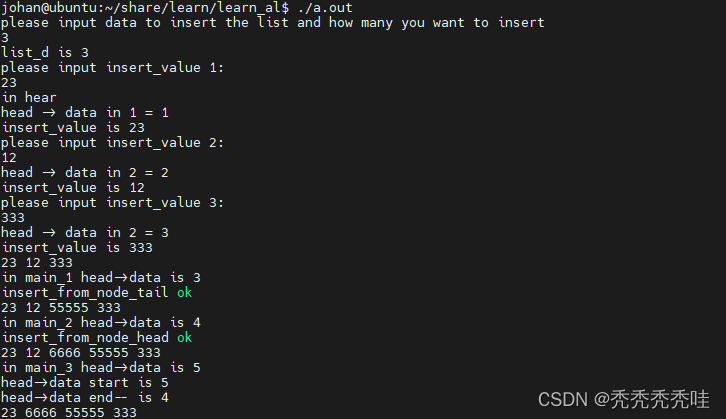