一、原生Ajax
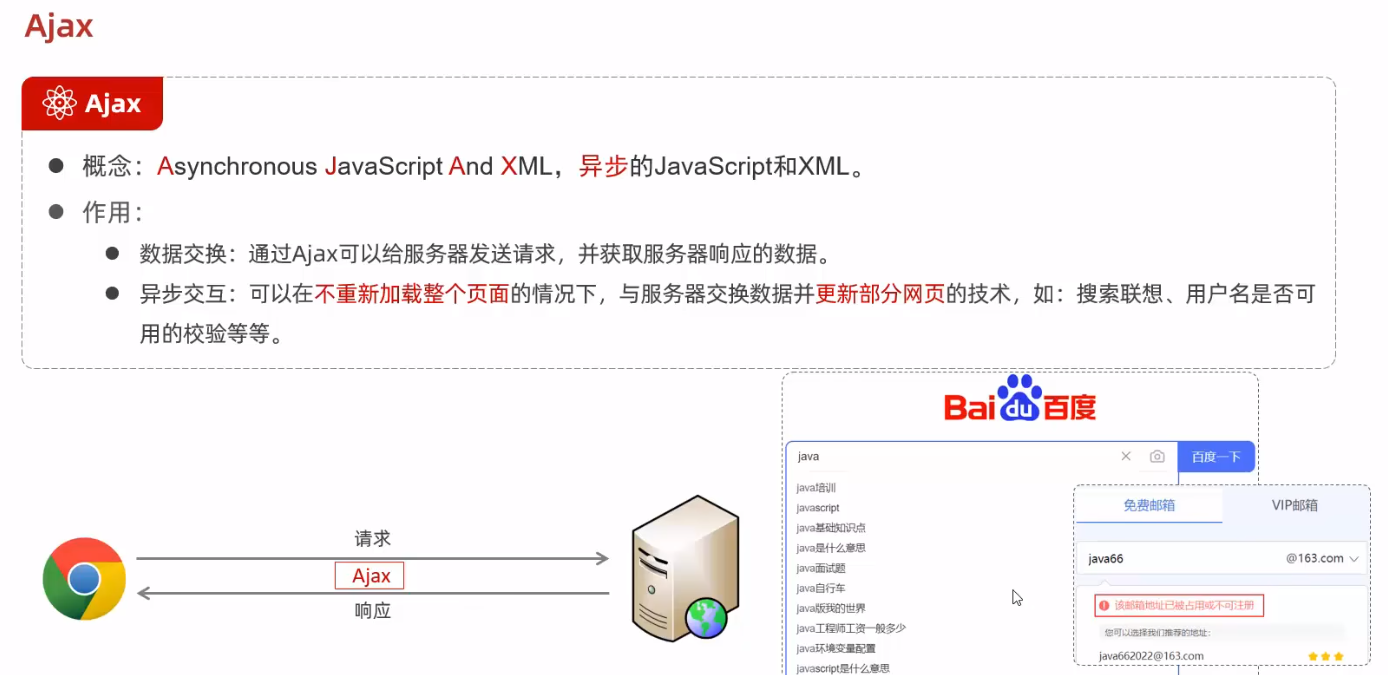
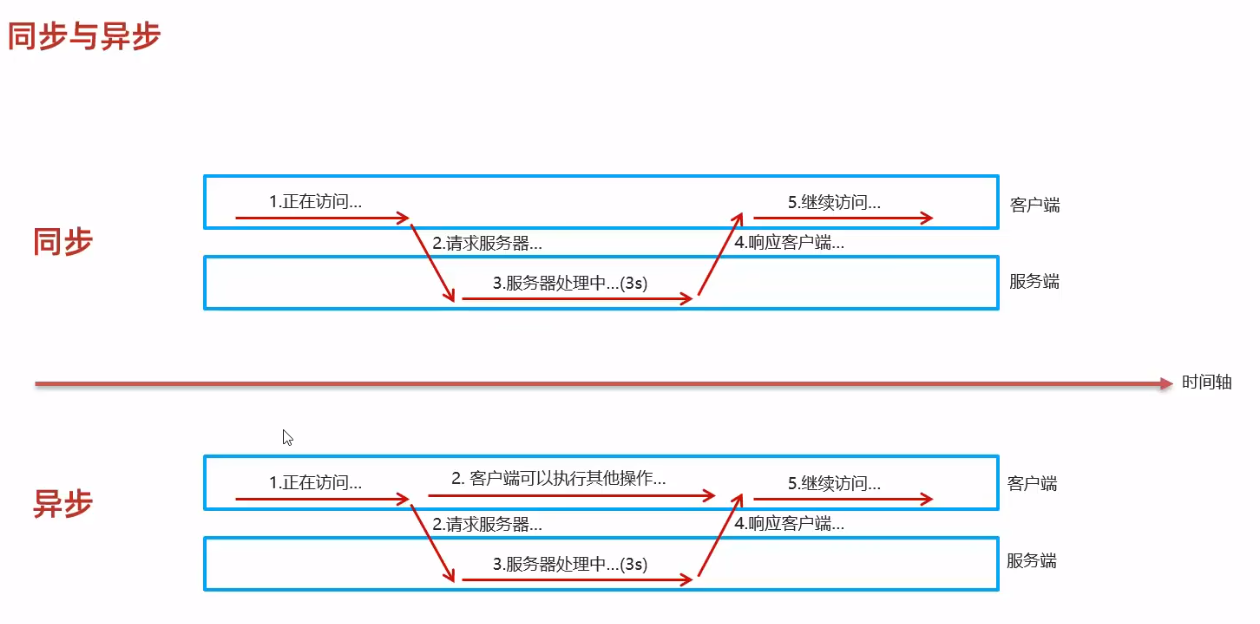
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="button" value="获取数据" onclick="getData()">
<div id="div1"></div>
</body>
<script>
var xmlhttprequest = new XMLHttpRequest();
xmlhttprequest.open("GET","http://yapi.smart-xwork.cn/mock/169327/emp/list");
xmlhttprequest.send();
xmlhttprequest.onreadystatechange = function () {
if(xmlhttprequest.readyState==4 && xmlhttprequest.status==200){
document.getElementById("div1").innerHTML = xmlhttprequest.responseText;
}
}
</script>
</html>
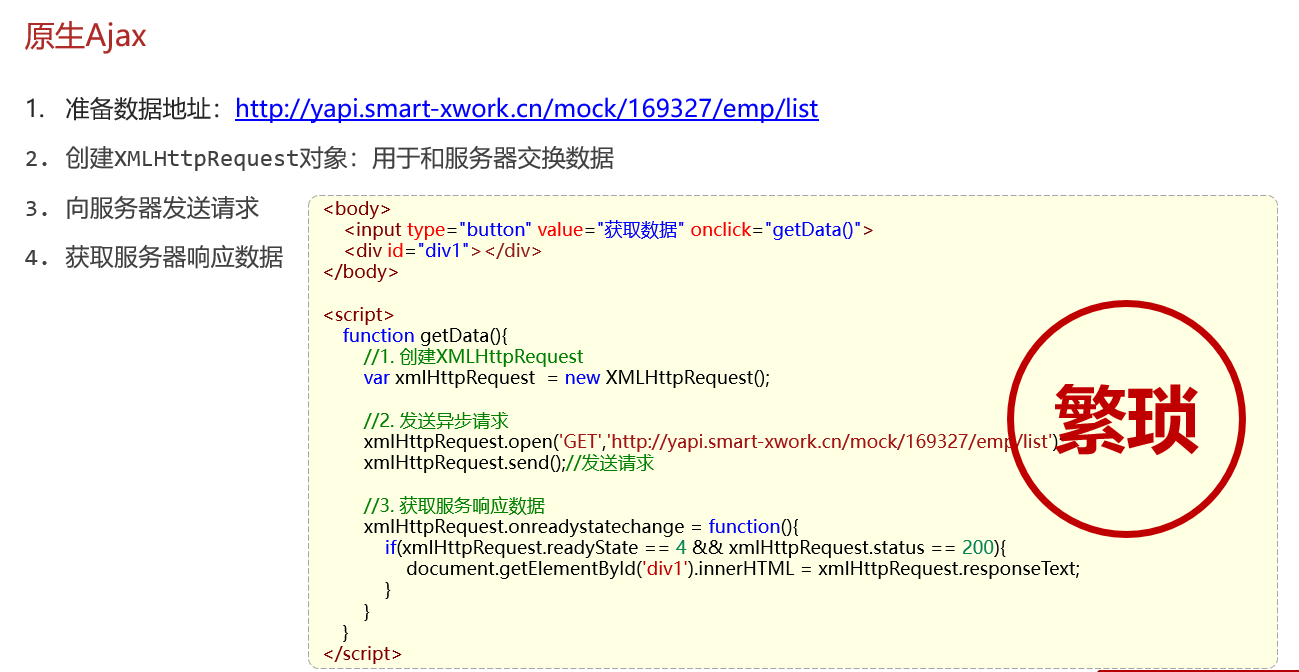

二、Axios
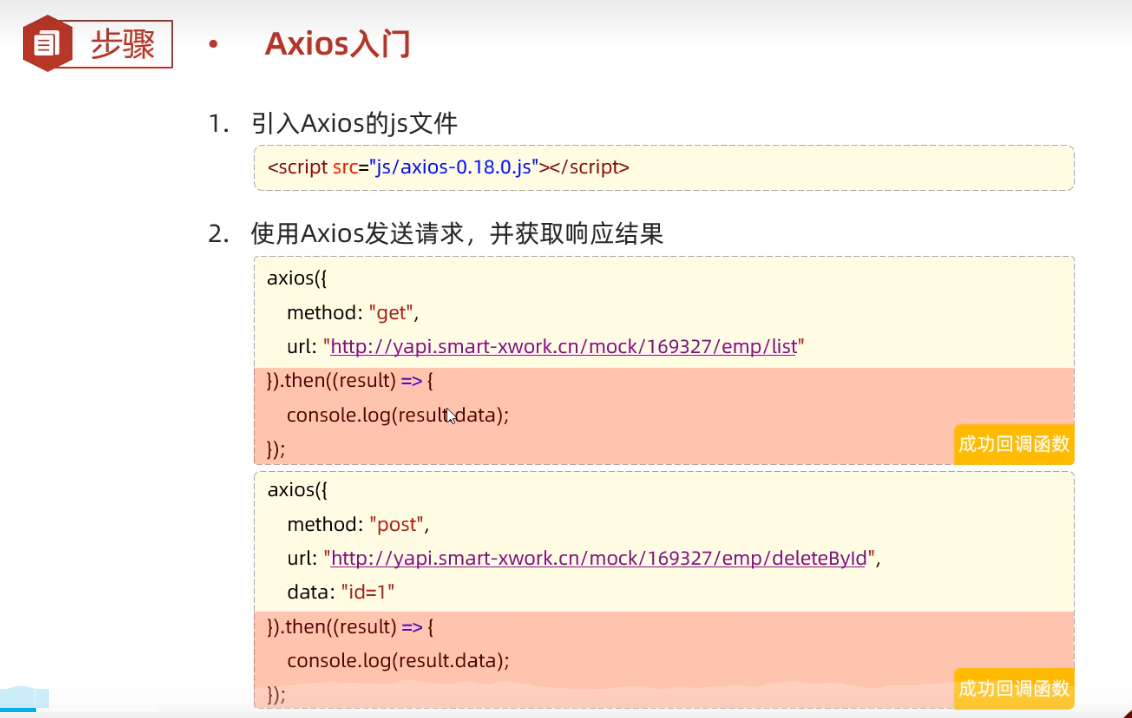
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="js/axios-0.18.0.js"></script>
</head>
<body>
<input type="button" value="get" onclick="getMethod()">
<input type="button" value="post" onclick="postMethod()">
</body>
<script>
function getMethod() {
axios.get("http://yapi.smart-xwork.cn/mock/169327/emp/list").then((result)=>{
console.log(result.data);
})
}
function postMethod(){
axios.post("http://yapi.smart-xwork.cn/mock/169327/emp/deleteById","id=1").then((result)=>{
console.log(result.data);
})
}
</script>
</html>
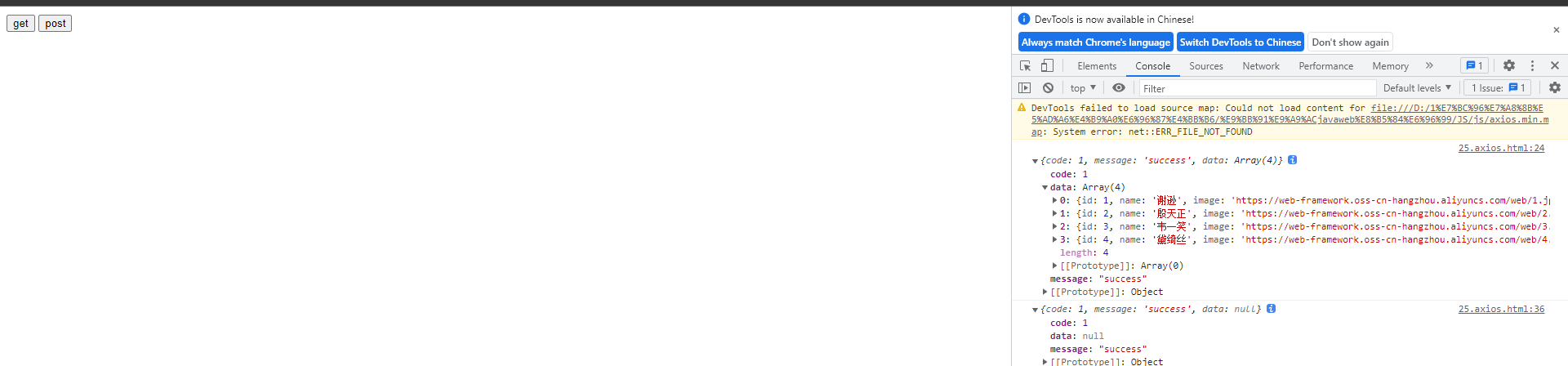
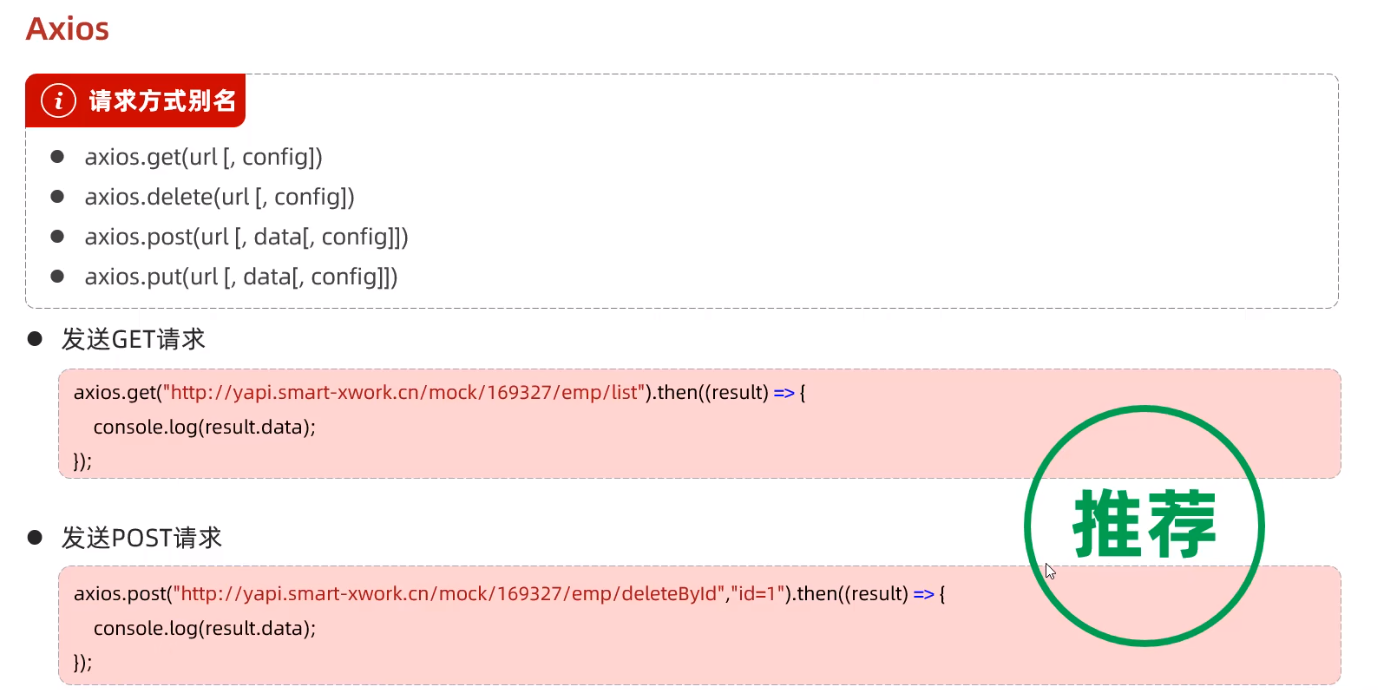
三、Axios案例
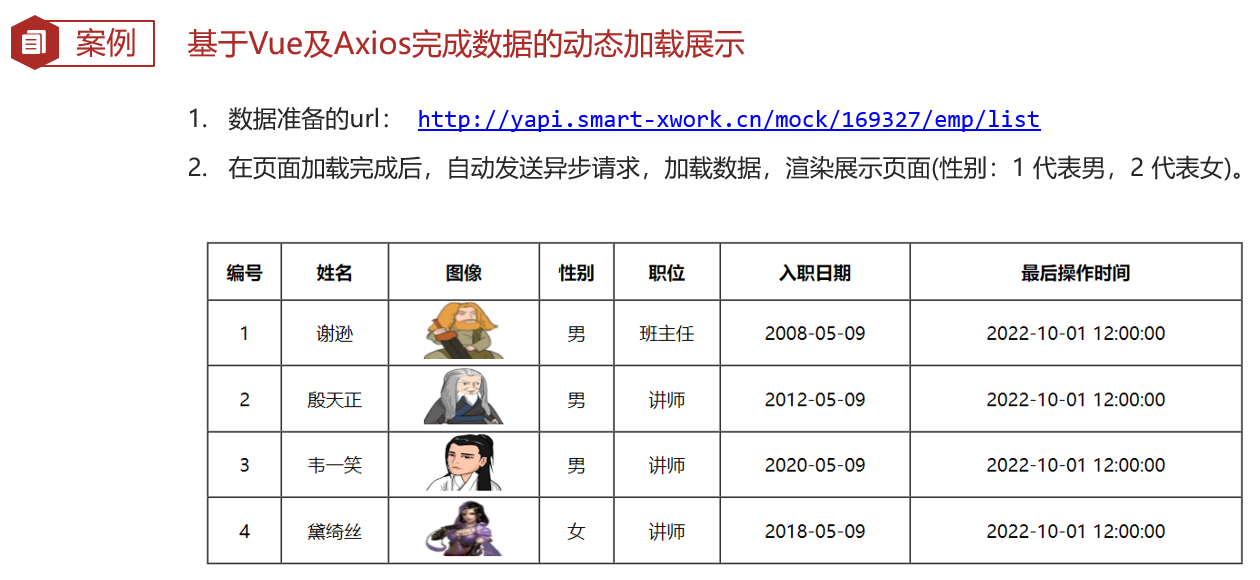
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>axios案例</title>
<script src="js/vue.js"></script>
<script src="js/axios-0.18.0.js"></script>
<style>
#app{
max-width: 1680px;
width:80%;
margin: 0 auto;
}
</style>
</head>
<body>
<div id="app">
<table border="1" cellspacing="0" style="margin: auto;">
<tr>
<th>编号</th>
<th>姓名</th>
<th>图像</th>
<th>性别</th>
<th>职位</th>
<th>入职日期</th>
<th>最后操作时间</th>
</tr>
<tr v-for="(user,index) in userall">
<td>{{index+1}}</td>
<td>{{user.name}}</td>
<td><img v-bind:src="user.image" width="100px"></td>
<td v-if="user.gender==1">男</td>
<td v-if="user.gender==2">女</td>
<td>{{user.job}}</td>
<td>{{user.entrydate}}</td>
<td>{{user.updatetime}}</td>
</tr>
</table>
</div>
</body>
<script>
new Vue({
el: "#app",
data:{
userall:[]
},
methods:{
},
mounted(){
axios.get("http://yapi.smart-xwork.cn/mock/169327/emp/list").then((result)=>{
this.userall=result.data.data;
});
}
})
</script>
</html>
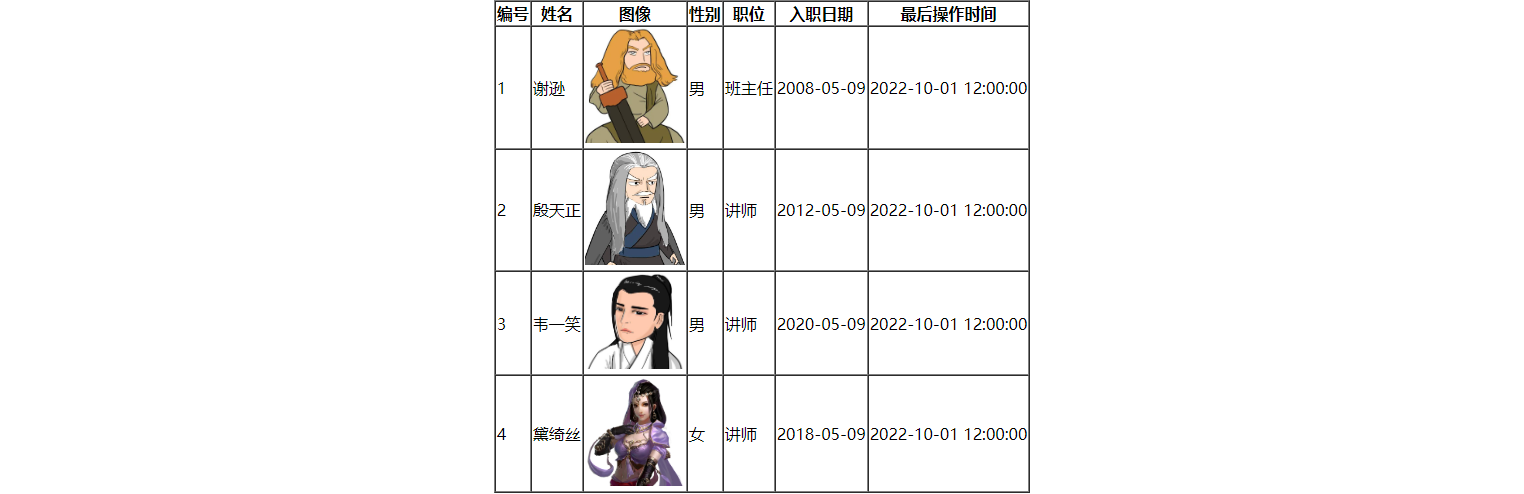