1、前端编辑页面加载水果库存信息逻辑edit.js
let queryString = window.location.search.substring(1)
if(queryString){
var fid = queryString.split("=")[1]
window.onload=function(){
loadFruit(fid)
}
loadFruit = function(fid){
axios({
method:'get',
url:'edit',
params:{
fid:fid
}
}).then(response=>{
let fruit = response.data.data
$("#fid").value=fruit.fid
$("#fname").value=fruit.fname
$("#price").value=fruit.price
$("#fcount").value=fruit.fcount
$("#remark").value=fruit.remark
})
}
/* update=function(){
let fid = $("#fid").value
let fname = $("#fname").value
let price = $("#price").value
let fcount = $("#fcount").value
let remark = $("#remark").value
axios({
method:'post',
url:"update",
data:{
fid:fid,
fname:fname,
price:price,
fcount:fcount,
remark:remark
}
}).then(response=>{
if(response.data.flag){
window.location.href="index.html"
}
})
}*/
}
2、 在FruitDao接口中添加通过id查询数据的方法
package com.csdn.fruit.dao;
import com.csdn.fruit.pojo.Fruit;
import java.util.List;
//dao :Data Access Object 数据访问对象
//接口设计
public interface FruitDao {
void addFruit(Fruit fruit);
void delFruit(String fname);
void updateFruit(Fruit fruit);
List<Fruit> getFruitList();
Fruit getFruitByFname(String fname);
Fruit getFruitByFid(Integer fid);
}
3、在FruitDaoImpl实现类中实现getFruitByFid()方法
package com.csdn.fruit.dao.impl;
import com.csdn.fruit.dao.FruitDao;
import com.csdn.fruit.pojo.Fruit;
import com.csdn.mymvc.dao.BaseDao;
import java.util.List;
public class FruitDaoImpl extends BaseDao<Fruit> implements FruitDao {
@Override
public void addFruit(Fruit fruit) {
String sql = "insert into t_fruit values (0,?,?,?,?)";
super.executeUpdate(sql, fruit.getFname(), fruit.getPrice(), fruit.getFcount(), fruit.getRemark());
}
@Override
public void delFruit(String fname) {
String sql = "delete from t_fruit where fname=?";
super.executeUpdate(sql, fname);
}
@Override
public void updateFruit(Fruit fruit) {
String sql = "update t_fruit set fcount=? where fname = ?";
super.executeUpdate(sql, fruit.getFcount(), fruit.getFname());
}
@Override
public List<Fruit> getFruitList() {
return super.executeQuery("select * from t_fruit");
}
@Override
public Fruit getFruitByFname(String fname) {
return load("select * from t_fruit where fname = ?", fname);
}
@Override
public Fruit getFruitByFid(Integer fid) {
return load("select * from t_fruit where fid=?", fid);
}
}
4、编写EditServlet类实现前后端数据交互
package com.csdn.fruit.servlet;
import com.csdn.fruit.dao.FruitDao;
import com.csdn.fruit.dao.impl.FruitDaoImpl;
import com.csdn.fruit.dto.Result;
import com.csdn.fruit.pojo.Fruit;
import com.csdn.fruit.util.ResponseUtil;
import jakarta.servlet.ServletException;
import jakarta.servlet.annotation.WebServlet;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/edit")
public class EditServlet extends HttpServlet {
FruitDao fruitDao = new FruitDaoImpl();
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
Integer fid = Integer.parseInt(req.getParameter("fid"));
Fruit fruit = fruitDao.getFruitByFid(fid);
ResponseUtil.print(resp, Result.OK(fruit));
}
}
5、用到的工具类
5.1、ResponseUtil工具类
package com.csdn.fruit.util;
import com.csdn.fruit.dto.Result;
import jakarta.servlet.ServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
public class ResponseUtil {
public static void print(ServletResponse response, Result result) throws IOException {
response.setCharacterEncoding("UTF-8");
response.setContentType("application/json;charset=utf-8");
PrintWriter out = response.getWriter();
out.println(GsonUtil.toJson(result));
out.flush();
}
}
5.2、GsonUtil工具类
package com.csdn.fruit.util;
import com.google.gson.Gson;
public class GsonUtil {
public static String toJson(Object obj) {
//java object -> java json string
Gson gson = new Gson();
return gson.toJson(obj);
}
}
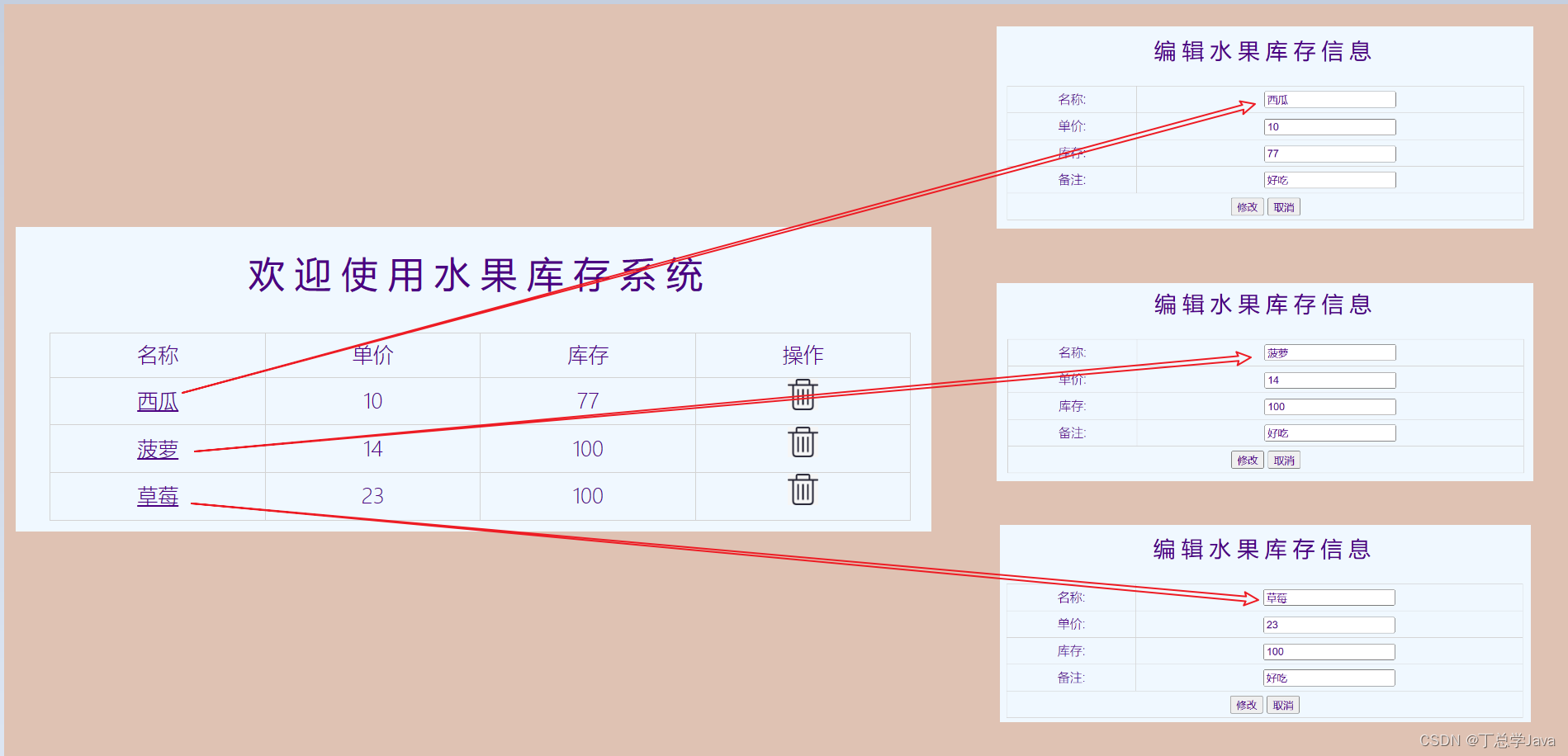