- 将容器空间分成五个区域:顶部(Top)、底部(Bottom)、左侧(Left)、右侧(Right)和中心(Center)。每个区域都可以放置一个节点(Node),比如另一个布局容器或UI控件
- BorderPane中的一些区域可以为空,只设置需要的区域即可
- 顶部和底部默认是横向扩展全宽的
- 左右两侧则会扩展到足够容纳内容的高度
- 中心区域通常用来放置主要的内容,默认会填充剩余的空间
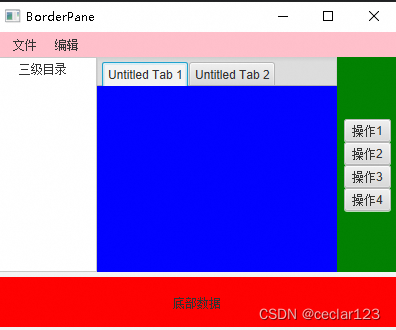
实现方式
Java实现
BorderPane pane = new BorderPane();
pane.setTop(new Label("top"));
pane.setCenter(new Label("center"));
pane.setBottom(new Label("bottom"));
pane.setLeft(new Label("left"));
pane.setRight(new Label("right"));
pane.setPadding(new Insets(5, 10, 10, 5));
FXML实现
<BorderPane xmlns="http://javafx.com/javafx" xmlns:fx="http://javafx.com/fxml" prefHeight="400.0" prefWidth="600.0">
<top>
<Button text="top"/>
</top>
<center>
<Button text="center"/>
</center>
<bottom>
<Button text="bottom"/>
</bottom>
<left>
<Button text="left"/>
</left>
<right>
<Button text="right"/>
</right>
</BorderPane>
综合案例
BorderPane pane = new BorderPane();
MenuBar menuBar = new MenuBar();
menuBar.setStyle("-fx-background-color: pink;");
Menu file = new Menu("文件");
file.getItems().add(new Menu("新建"));
file.getItems().add(new Menu("打开"));
menuBar.getMenus().add(file);
menuBar.getMenus().add(new Menu("编辑"));
pane.setTop(menuBar);
TabPane tabPane = new TabPane();
tabPane.setStyle("-fx-background-color: blue;");
Tab tab1 = new Tab("Untitled Tab 1");
tab1.setContent(new FlowPane());
tabPane.getTabs().add(tab1);
Tab tab2 = new Tab("Untitled Tab 2");
tab2.setContent(new FlowPane());
tabPane.getTabs().add(tab2);
pane.setCenter(tabPane);
FlowPane flowPaneBottom = new FlowPane();
flowPaneBottom.setStyle("-fx-background-color: red;");
flowPaneBottom.setAlignment(Pos.CENTER);
flowPaneBottom.getChildren().add(new Label("底部数据"));
pane.setBottom(flowPaneBottom);
TreeItem<String> root = new TreeItem<String>("root");
root.getChildren().add(new TreeItem<String>("一级目录"));
root.getChildren().add(new TreeItem<String>("二级目录"));
root.getChildren().add(new TreeItem<String>("三级目录"));
TreeView<String> treeView = new TreeView<String>(root);
treeView.setPrefWidth(100);
pane.setLeft(treeView);
FlowPane flowPaneRight = new FlowPane();
flowPaneRight.setStyle("-fx-background-color: green;");
flowPaneRight.setOrientation(Orientation.VERTICAL);
flowPaneRight.setAlignment(Pos.CENTER);
flowPaneRight.getChildren().add(new Button("操作1"));
flowPaneRight.getChildren().add(new Button("操作2"));
flowPaneRight.getChildren().add(new Button("操作3"));
flowPaneRight.getChildren().add(new Button("操作4"));
flowPaneRight.getChildren().add(new Button("操作5"));
pane.setRight(flowPaneRight);
return pane;
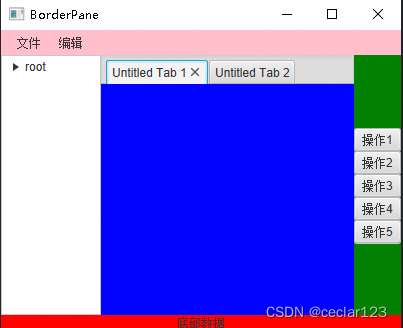
<BorderPane prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/17.0.2-ea" xmlns:fx="http://javafx.com/fxml/1" >
<top>
<MenuBar style="-fx-background-color: pink;">
<Menu styleClass="menu-item" text="文件">
<Menu text="新建"/>
<Menu text="打开"/>
</Menu>
<Menu text="编辑"/>
</MenuBar>
</top>
<center>
<TabPane fx:id="tabPane1" prefHeight="200.0" prefWidth="200.0" styleClass="tab-pane" style="-fx-background-color: blue;"
tabClosingPolicy="UNAVAILABLE" BorderPane.alignment="CENTER">
<tabs>
<Tab fx:id="tab1" text="Untitled Tab 1">
<content>
<FlowPane fx:id="flowPane1" minHeight="0.0" minWidth="0.0" prefHeight="180.0"
prefWidth="200.0"/>
</content>
</Tab>
<Tab fx:id="tab2" text="Untitled Tab 2">
<content>
<FlowPane minHeight="0.0" minWidth="0.0" prefHeight="180.0" prefWidth="200.0">
<Button styleClass="btn btn-primary" text="添加Button"/>
<Button styleClass="btn btn-primary" text="添加Tab"/>
</FlowPane>
</content>
</Tab>
</tabs>
</TabPane>
</center>
<left>
<TreeView prefWidth="100" >
<TreeItem value="root">
<children>
<TreeItem value="一级目录"/>
<TreeItem value="二级目录"/>
<TreeItem value="三级目录"/>
</children>
</TreeItem>
</TreeView>
</left>
<right>
<FlowPane alignment="CENTER" orientation="VERTICAL" prefWidth="60" style="-fx-background-color: green;">
<Button text="操作1"/>
<Button text="操作2"/>
<Button text="操作3"/>
<Button text="操作4"/>
</FlowPane>
</right>
<bottom>
<FlowPane alignment="CENTER" prefHeight="50" style="-fx-background-color: red;" BorderPane.alignment="CENTER">
<Label text="底部数据"/>
<BorderPane.margin>
<Insets top="5.0" left="0" right="0" bottom="5"/>
</BorderPane.margin>
</FlowPane>
</bottom>
</BorderPane>